mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-15 23:25:07 +00:00
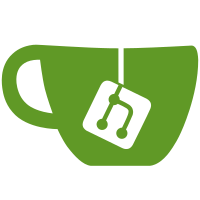
Rename __down_read() in __down_read_common() and teach it to abort waiting in case of pending signals and killable state argument passed. Note, that we shouldn't wake anybody up in EINTR path, as: We check for signal_pending_state() after (!waiter.task) test and under spinlock. So, current task wasn't able to be woken up. It may be in two cases: a writer is owner of the sem, or a writer is a first waiter of the sem. If a writer is owner of the sem, no one else may work with it in parallel. It will wake somebody, when it call up_write() or downgrade_write(). If a writer is the first waiter, it will be woken up, when the last active reader releases the sem, and sem->count became 0. Also note, that set_current_state() may be moved down to schedule() (after !waiter.task check), as all assignments in this type of semaphore (including wake_up), occur under spinlock, so we can't miss anything. Signed-off-by: Kirill Tkhai <ktkhai@virtuozzo.com> Signed-off-by: Peter Zijlstra (Intel) <peterz@infradead.org> Cc: Linus Torvalds <torvalds@linux-foundation.org> Cc: Peter Zijlstra <peterz@infradead.org> Cc: Thomas Gleixner <tglx@linutronix.de> Cc: arnd@arndb.de Cc: avagin@virtuozzo.com Cc: davem@davemloft.net Cc: fenghua.yu@intel.com Cc: gorcunov@virtuozzo.com Cc: heiko.carstens@de.ibm.com Cc: hpa@zytor.com Cc: ink@jurassic.park.msu.ru Cc: mattst88@gmail.com Cc: rth@twiddle.net Cc: schwidefsky@de.ibm.com Cc: tony.luck@intel.com Link: http://lkml.kernel.org/r/149789533283.9059.9829416940494747182.stgit@localhost.localdomain Signed-off-by: Ingo Molnar <mingo@kernel.org>
46 lines
1.5 KiB
C
46 lines
1.5 KiB
C
/* rwsem-spinlock.h: fallback C implementation
|
|
*
|
|
* Copyright (c) 2001 David Howells (dhowells@redhat.com).
|
|
* - Derived partially from ideas by Andrea Arcangeli <andrea@suse.de>
|
|
* - Derived also from comments by Linus
|
|
*/
|
|
|
|
#ifndef _LINUX_RWSEM_SPINLOCK_H
|
|
#define _LINUX_RWSEM_SPINLOCK_H
|
|
|
|
#ifndef _LINUX_RWSEM_H
|
|
#error "please don't include linux/rwsem-spinlock.h directly, use linux/rwsem.h instead"
|
|
#endif
|
|
|
|
#ifdef __KERNEL__
|
|
/*
|
|
* the rw-semaphore definition
|
|
* - if count is 0 then there are no active readers or writers
|
|
* - if count is +ve then that is the number of active readers
|
|
* - if count is -1 then there is one active writer
|
|
* - if wait_list is not empty, then there are processes waiting for the semaphore
|
|
*/
|
|
struct rw_semaphore {
|
|
__s32 count;
|
|
raw_spinlock_t wait_lock;
|
|
struct list_head wait_list;
|
|
#ifdef CONFIG_DEBUG_LOCK_ALLOC
|
|
struct lockdep_map dep_map;
|
|
#endif
|
|
};
|
|
|
|
#define RWSEM_UNLOCKED_VALUE 0x00000000
|
|
|
|
extern void __down_read(struct rw_semaphore *sem);
|
|
extern int __must_check __down_read_killable(struct rw_semaphore *sem);
|
|
extern int __down_read_trylock(struct rw_semaphore *sem);
|
|
extern void __down_write(struct rw_semaphore *sem);
|
|
extern int __must_check __down_write_killable(struct rw_semaphore *sem);
|
|
extern int __down_write_trylock(struct rw_semaphore *sem);
|
|
extern void __up_read(struct rw_semaphore *sem);
|
|
extern void __up_write(struct rw_semaphore *sem);
|
|
extern void __downgrade_write(struct rw_semaphore *sem);
|
|
extern int rwsem_is_locked(struct rw_semaphore *sem);
|
|
|
|
#endif /* __KERNEL__ */
|
|
#endif /* _LINUX_RWSEM_SPINLOCK_H */
|