mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-04 16:15:11 +00:00
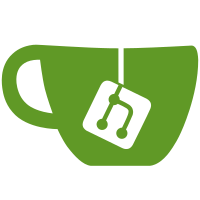
Some of the SRQ types are created using a WQ, and the WQ requires a
different parameter set to mlx5_umem_find_best_quantized_pgoff() as it has
a 5 bit page_offset.
Add the umem to the mlx5_srq_attr and defer computing the PAS data until
the code has figured out what kind of mailbox to use. Compute the PAS
directly from the umem for each of the four unique mailbox types.
This also avoids allocating memory to store the user PAS, instead it is
written directly to the mailbox as in most other cases.
Fixes: 01949d0109
("net/mlx5_core: Enable XRCs and SRQs when using ISSI > 0")
Link: https://lore.kernel.org/r/20201115114311.136250-8-leon@kernel.org
Signed-off-by: Leon Romanovsky <leonro@nvidia.com>
Signed-off-by: Jason Gunthorpe <jgg@nvidia.com>
69 lines
1.5 KiB
C
69 lines
1.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0 OR Linux-OpenIB */
|
|
/*
|
|
* Copyright (c) 2013-2018, Mellanox Technologies. All rights reserved.
|
|
*/
|
|
|
|
#ifndef MLX5_IB_SRQ_H
|
|
#define MLX5_IB_SRQ_H
|
|
|
|
enum {
|
|
MLX5_SRQ_FLAG_ERR = (1 << 0),
|
|
MLX5_SRQ_FLAG_WQ_SIG = (1 << 1),
|
|
MLX5_SRQ_FLAG_RNDV = (1 << 2),
|
|
};
|
|
|
|
struct mlx5_srq_attr {
|
|
u32 type;
|
|
u32 flags;
|
|
u32 log_size;
|
|
u32 wqe_shift;
|
|
u32 log_page_size;
|
|
u32 wqe_cnt;
|
|
u32 srqn;
|
|
u32 xrcd;
|
|
u32 page_offset;
|
|
u32 cqn;
|
|
u32 pd;
|
|
u32 lwm;
|
|
u32 user_index;
|
|
u64 db_record;
|
|
__be64 *pas;
|
|
struct ib_umem *umem;
|
|
u32 tm_log_list_size;
|
|
u32 tm_next_tag;
|
|
u32 tm_hw_phase_cnt;
|
|
u32 tm_sw_phase_cnt;
|
|
u16 uid;
|
|
};
|
|
|
|
struct mlx5_ib_dev;
|
|
|
|
struct mlx5_core_srq {
|
|
struct mlx5_core_rsc_common common; /* must be first */
|
|
u32 srqn;
|
|
int max;
|
|
size_t max_gs;
|
|
size_t max_avail_gather;
|
|
int wqe_shift;
|
|
void (*event)(struct mlx5_core_srq *srq, enum mlx5_event e);
|
|
|
|
u16 uid;
|
|
};
|
|
|
|
struct mlx5_srq_table {
|
|
struct notifier_block nb;
|
|
struct xarray array;
|
|
};
|
|
|
|
int mlx5_cmd_create_srq(struct mlx5_ib_dev *dev, struct mlx5_core_srq *srq,
|
|
struct mlx5_srq_attr *in);
|
|
int mlx5_cmd_destroy_srq(struct mlx5_ib_dev *dev, struct mlx5_core_srq *srq);
|
|
int mlx5_cmd_query_srq(struct mlx5_ib_dev *dev, struct mlx5_core_srq *srq,
|
|
struct mlx5_srq_attr *out);
|
|
int mlx5_cmd_arm_srq(struct mlx5_ib_dev *dev, struct mlx5_core_srq *srq,
|
|
u16 lwm, int is_srq);
|
|
struct mlx5_core_srq *mlx5_cmd_get_srq(struct mlx5_ib_dev *dev, u32 srqn);
|
|
|
|
int mlx5_init_srq_table(struct mlx5_ib_dev *dev);
|
|
void mlx5_cleanup_srq_table(struct mlx5_ib_dev *dev);
|
|
#endif /* MLX5_IB_SRQ_H */
|