mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-24 09:50:04 +00:00
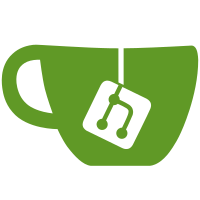
The memcpy template is a bit unusual in the way it manages the stack
pointer: depending on the execution path through the function, the SP
assumes different values as different subsets of the register file are
preserved and restored again. This is problematic when it comes to EHABI
unwind info, as it is not instruction accurate, and does not allow
tracking the SP value as it changes.
Commit 279f487e0b
("ARM: 8225/1: Add unwinding support for memory
copy functions") addressed this by carving up the function in different
chunks as far as the unwinder is concerned, and keeping a set of unwind
directives for each of them, each corresponding with the state of the
stack pointer during execution of the chunk in question. This not only
duplicates unwind info unnecessarily, but it also complicates unwinding
the stack upon overflow.
Instead, let's do what the compiler does when the SP is updated halfway
through a function, which is to use a frame pointer and emit the
appropriate unwind directives to communicate this to the unwinder.
Note that Thumb-2 uses R7 for this, while ARM uses R11 aka FP. So let's
avoid touching R7 in the body of the template, so that Thumb-2 can use
it as the frame pointer. R11 was not modified in the first place.
Signed-off-by: Ard Biesheuvel <ardb@kernel.org>
Tested-by: Keith Packard <keithpac@amazon.com>
Tested-by: Marc Zyngier <maz@kernel.org>
Tested-by: Vladimir Murzin <vladimir.murzin@arm.com> # ARMv7M
125 lines
2.3 KiB
ArmAsm
125 lines
2.3 KiB
ArmAsm
/* SPDX-License-Identifier: GPL-2.0-only */
|
|
/*
|
|
* linux/arch/arm/lib/copy_to_user.S
|
|
*
|
|
* Author: Nicolas Pitre
|
|
* Created: Sep 29, 2005
|
|
* Copyright: MontaVista Software, Inc.
|
|
*/
|
|
|
|
#include <linux/linkage.h>
|
|
#include <asm/assembler.h>
|
|
#include <asm/unwind.h>
|
|
|
|
/*
|
|
* Prototype:
|
|
*
|
|
* size_t arm_copy_to_user(void *to, const void *from, size_t n)
|
|
*
|
|
* Purpose:
|
|
*
|
|
* copy a block to user memory from kernel memory
|
|
*
|
|
* Params:
|
|
*
|
|
* to = user memory
|
|
* from = kernel memory
|
|
* n = number of bytes to copy
|
|
*
|
|
* Return value:
|
|
*
|
|
* Number of bytes NOT copied.
|
|
*/
|
|
|
|
#define LDR1W_SHIFT 0
|
|
|
|
.macro ldr1w ptr reg abort
|
|
W(ldr) \reg, [\ptr], #4
|
|
.endm
|
|
|
|
.macro ldr4w ptr reg1 reg2 reg3 reg4 abort
|
|
ldmia \ptr!, {\reg1, \reg2, \reg3, \reg4}
|
|
.endm
|
|
|
|
.macro ldr8w ptr reg1 reg2 reg3 reg4 reg5 reg6 reg7 reg8 abort
|
|
ldmia \ptr!, {\reg1, \reg2, \reg3, \reg4, \reg5, \reg6, \reg7, \reg8}
|
|
.endm
|
|
|
|
.macro ldr1b ptr reg cond=al abort
|
|
ldrb\cond \reg, [\ptr], #1
|
|
.endm
|
|
|
|
#ifdef CONFIG_CPU_USE_DOMAINS
|
|
|
|
#ifndef CONFIG_THUMB2_KERNEL
|
|
#define STR1W_SHIFT 0
|
|
#else
|
|
#define STR1W_SHIFT 1
|
|
#endif
|
|
|
|
.macro str1w ptr reg abort
|
|
strusr \reg, \ptr, 4, abort=\abort
|
|
.endm
|
|
|
|
.macro str8w ptr reg1 reg2 reg3 reg4 reg5 reg6 reg7 reg8 abort
|
|
str1w \ptr, \reg1, \abort
|
|
str1w \ptr, \reg2, \abort
|
|
str1w \ptr, \reg3, \abort
|
|
str1w \ptr, \reg4, \abort
|
|
str1w \ptr, \reg5, \abort
|
|
str1w \ptr, \reg6, \abort
|
|
str1w \ptr, \reg7, \abort
|
|
str1w \ptr, \reg8, \abort
|
|
.endm
|
|
|
|
#else
|
|
|
|
#define STR1W_SHIFT 0
|
|
|
|
.macro str1w ptr reg abort
|
|
USERL(\abort, W(str) \reg, [\ptr], #4)
|
|
.endm
|
|
|
|
.macro str8w ptr reg1 reg2 reg3 reg4 reg5 reg6 reg7 reg8 abort
|
|
USERL(\abort, stmia \ptr!, {\reg1, \reg2, \reg3, \reg4, \reg5, \reg6, \reg7, \reg8})
|
|
.endm
|
|
|
|
#endif /* CONFIG_CPU_USE_DOMAINS */
|
|
|
|
.macro str1b ptr reg cond=al abort
|
|
strusr \reg, \ptr, 1, \cond, abort=\abort
|
|
.endm
|
|
|
|
.macro enter regs:vararg
|
|
mov r3, #0
|
|
UNWIND( .save {r0, r2, r3, \regs} )
|
|
stmdb sp!, {r0, r2, r3, \regs}
|
|
.endm
|
|
|
|
.macro exit regs:vararg
|
|
add sp, sp, #8
|
|
ldmfd sp!, {r0, \regs}
|
|
.endm
|
|
|
|
.text
|
|
|
|
ENTRY(__copy_to_user_std)
|
|
WEAK(arm_copy_to_user)
|
|
#ifdef CONFIG_CPU_SPECTRE
|
|
ldr r3, =TASK_SIZE
|
|
uaccess_mask_range_ptr r0, r2, r3, ip
|
|
#endif
|
|
|
|
#include "copy_template.S"
|
|
|
|
ENDPROC(arm_copy_to_user)
|
|
ENDPROC(__copy_to_user_std)
|
|
|
|
.pushsection .text.fixup,"ax"
|
|
.align 0
|
|
copy_abort_preamble
|
|
ldmfd sp!, {r1, r2, r3}
|
|
sub r0, r0, r1
|
|
rsb r0, r0, r2
|
|
copy_abort_end
|
|
.popsection
|