mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-25 11:55:37 +00:00
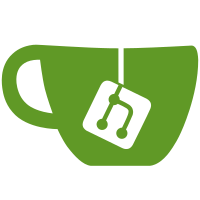
The dfops structure used by multi-transaction operations is typically stored on the stack and carried around by the associated transaction. The lifecycle of dfops does not quite match that of the transaction, but they are tightly related in that the former depends on the latter. The relationship of these objects is tight enough that we can avoid the cumbersome boilerplate code required in most cases to manage them separately by just embedding an xfs_defer_ops in the transaction itself. This means that a transaction allocation returns with an initialized dfops, a transaction commit finishes pending deferred items before the tx commit, a transaction cancel cancels the dfops before the transaction and a transaction dup operation transfers the current dfops state to the new transaction. The dup operation is slightly complicated by the fact that we can no longer just copy a dfops pointer from the old transaction to the new transaction. This is solved through a dfops move helper that transfers the pending items and other dfops state across the transactions. This also requires that transaction rolling code always refer to the transaction for the current dfops reference. Finally, to facilitate incremental conversion to the internal dfops and continue to support the current external dfops mode of operation, create the new ->t_dfops_internal field with a layer of indirection. On allocation, ->t_dfops points to the internal dfops. This state is overridden by callers who re-init a local dfops on the transaction. Once ->t_dfops is overridden, the external dfops reference is maintained as the transaction rolls. This patch adds the fundamental ability to support an internal dfops. All codepaths that perform deferred processing continue to override the internal dfops until they are converted over in subsequent patches. Signed-off-by: Brian Foster <bfoster@redhat.com> Reviewed-by: Bill O'Donnell <billodo@redhat.com> Reviewed-by: Christoph Hellwig <hch@lst.de> Reviewed-by: Darrick J. Wong <darrick.wong@oracle.com> Signed-off-by: Darrick J. Wong <darrick.wong@oracle.com>
76 lines
2.9 KiB
C
76 lines
2.9 KiB
C
// SPDX-License-Identifier: GPL-2.0+
|
|
/*
|
|
* Copyright (C) 2016 Oracle. All Rights Reserved.
|
|
* Author: Darrick J. Wong <darrick.wong@oracle.com>
|
|
*/
|
|
#ifndef __XFS_DEFER_H__
|
|
#define __XFS_DEFER_H__
|
|
|
|
struct xfs_defer_op_type;
|
|
struct xfs_defer_ops;
|
|
|
|
/*
|
|
* Save a log intent item and a list of extents, so that we can replay
|
|
* whatever action had to happen to the extent list and file the log done
|
|
* item.
|
|
*/
|
|
struct xfs_defer_pending {
|
|
const struct xfs_defer_op_type *dfp_type; /* function pointers */
|
|
struct list_head dfp_list; /* pending items */
|
|
void *dfp_intent; /* log intent item */
|
|
void *dfp_done; /* log done item */
|
|
struct list_head dfp_work; /* work items */
|
|
unsigned int dfp_count; /* # extent items */
|
|
};
|
|
|
|
/*
|
|
* Header for deferred operation list.
|
|
*
|
|
* dop_low is used by the allocator to activate the lowspace algorithm -
|
|
* when free space is running low the extent allocator may choose to
|
|
* allocate an extent from an AG without leaving sufficient space for
|
|
* a btree split when inserting the new extent. In this case the allocator
|
|
* will enable the lowspace algorithm which is supposed to allow further
|
|
* allocations (such as btree splits and newroots) to allocate from
|
|
* sequential AGs. In order to avoid locking AGs out of order the lowspace
|
|
* algorithm will start searching for free space from AG 0. If the correct
|
|
* transaction reservations have been made then this algorithm will eventually
|
|
* find all the space it needs.
|
|
*/
|
|
enum xfs_defer_ops_type {
|
|
XFS_DEFER_OPS_TYPE_BMAP,
|
|
XFS_DEFER_OPS_TYPE_REFCOUNT,
|
|
XFS_DEFER_OPS_TYPE_RMAP,
|
|
XFS_DEFER_OPS_TYPE_FREE,
|
|
XFS_DEFER_OPS_TYPE_AGFL_FREE,
|
|
XFS_DEFER_OPS_TYPE_MAX,
|
|
};
|
|
|
|
void xfs_defer_add(struct xfs_defer_ops *dop, enum xfs_defer_ops_type type,
|
|
struct list_head *h);
|
|
int xfs_defer_finish(struct xfs_trans **tp, struct xfs_defer_ops *dop);
|
|
void xfs_defer_cancel(struct xfs_defer_ops *dop);
|
|
void xfs_defer_init(struct xfs_trans *tp, struct xfs_defer_ops *dop);
|
|
bool xfs_defer_has_unfinished_work(struct xfs_defer_ops *dop);
|
|
int xfs_defer_ijoin(struct xfs_defer_ops *dop, struct xfs_inode *ip);
|
|
int xfs_defer_bjoin(struct xfs_defer_ops *dop, struct xfs_buf *bp);
|
|
void xfs_defer_move(struct xfs_defer_ops *dst, struct xfs_defer_ops *src);
|
|
|
|
/* Description of a deferred type. */
|
|
struct xfs_defer_op_type {
|
|
enum xfs_defer_ops_type type;
|
|
unsigned int max_items;
|
|
void (*abort_intent)(void *);
|
|
void *(*create_done)(struct xfs_trans *, void *, unsigned int);
|
|
int (*finish_item)(struct xfs_trans *, struct xfs_defer_ops *,
|
|
struct list_head *, void *, void **);
|
|
void (*finish_cleanup)(struct xfs_trans *, void *, int);
|
|
void (*cancel_item)(struct list_head *);
|
|
int (*diff_items)(void *, struct list_head *, struct list_head *);
|
|
void *(*create_intent)(struct xfs_trans *, uint);
|
|
void (*log_item)(struct xfs_trans *, void *, struct list_head *);
|
|
};
|
|
|
|
void xfs_defer_init_op_type(const struct xfs_defer_op_type *type);
|
|
|
|
#endif /* __XFS_DEFER_H__ */
|