mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-20 01:20:54 +00:00
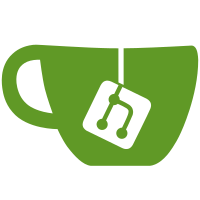
Currently we are not including randomized stack size when calculating mmap_base address in arch_pick_mmap_layout for topdown case. This might cause that mmap_base starts in the stack reserved area because stack is randomized by 1GB for 64b (8MB for 32b) and the minimum gap is 128MB. If the stack really grows down to mmap_base then we can get silent mmap region overwrite by the stack values. Let's include maximum stack randomization size into MIN_GAP which is used as the low bound for the gap in mmap. Signed-off-by: Michal Hocko <mhocko@suse.cz> LKML-Reference: <1252400515-6866-1-git-send-email-mhocko@suse.cz> Acked-by: Jiri Kosina <jkosina@suse.cz> Signed-off-by: H. Peter Anvin <hpa@zytor.com> Cc: Stable Team <stable@kernel.org>
136 lines
3.3 KiB
C
136 lines
3.3 KiB
C
/*
|
|
* Flexible mmap layout support
|
|
*
|
|
* Based on code by Ingo Molnar and Andi Kleen, copyrighted
|
|
* as follows:
|
|
*
|
|
* Copyright 2003-2009 Red Hat Inc.
|
|
* All Rights Reserved.
|
|
* Copyright 2005 Andi Kleen, SUSE Labs.
|
|
* Copyright 2007 Jiri Kosina, SUSE Labs.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License as published by
|
|
* the Free Software Foundation; either version 2 of the License, or
|
|
* (at your option) any later version.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* You should have received a copy of the GNU General Public License
|
|
* along with this program; if not, write to the Free Software
|
|
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
|
|
*/
|
|
|
|
#include <linux/personality.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/random.h>
|
|
#include <linux/limits.h>
|
|
#include <linux/sched.h>
|
|
#include <asm/elf.h>
|
|
|
|
static unsigned int stack_maxrandom_size(void)
|
|
{
|
|
unsigned int max = 0;
|
|
if ((current->flags & PF_RANDOMIZE) &&
|
|
!(current->personality & ADDR_NO_RANDOMIZE)) {
|
|
max = ((-1U) & STACK_RND_MASK) << PAGE_SHIFT;
|
|
}
|
|
|
|
return max;
|
|
}
|
|
|
|
|
|
/*
|
|
* Top of mmap area (just below the process stack).
|
|
*
|
|
* Leave an at least ~128 MB hole with possible stack randomization.
|
|
*/
|
|
#define MIN_GAP (128*1024*1024UL + stack_maxrandom_size())
|
|
#define MAX_GAP (TASK_SIZE/6*5)
|
|
|
|
/*
|
|
* True on X86_32 or when emulating IA32 on X86_64
|
|
*/
|
|
static int mmap_is_ia32(void)
|
|
{
|
|
#ifdef CONFIG_X86_32
|
|
return 1;
|
|
#endif
|
|
#ifdef CONFIG_IA32_EMULATION
|
|
if (test_thread_flag(TIF_IA32))
|
|
return 1;
|
|
#endif
|
|
return 0;
|
|
}
|
|
|
|
static int mmap_is_legacy(void)
|
|
{
|
|
if (current->personality & ADDR_COMPAT_LAYOUT)
|
|
return 1;
|
|
|
|
if (current->signal->rlim[RLIMIT_STACK].rlim_cur == RLIM_INFINITY)
|
|
return 1;
|
|
|
|
return sysctl_legacy_va_layout;
|
|
}
|
|
|
|
static unsigned long mmap_rnd(void)
|
|
{
|
|
unsigned long rnd = 0;
|
|
|
|
/*
|
|
* 8 bits of randomness in 32bit mmaps, 20 address space bits
|
|
* 28 bits of randomness in 64bit mmaps, 40 address space bits
|
|
*/
|
|
if (current->flags & PF_RANDOMIZE) {
|
|
if (mmap_is_ia32())
|
|
rnd = (long)get_random_int() % (1<<8);
|
|
else
|
|
rnd = (long)(get_random_int() % (1<<28));
|
|
}
|
|
return rnd << PAGE_SHIFT;
|
|
}
|
|
|
|
static unsigned long mmap_base(void)
|
|
{
|
|
unsigned long gap = current->signal->rlim[RLIMIT_STACK].rlim_cur;
|
|
|
|
if (gap < MIN_GAP)
|
|
gap = MIN_GAP;
|
|
else if (gap > MAX_GAP)
|
|
gap = MAX_GAP;
|
|
|
|
return PAGE_ALIGN(TASK_SIZE - gap - mmap_rnd());
|
|
}
|
|
|
|
/*
|
|
* Bottom-up (legacy) layout on X86_32 did not support randomization, X86_64
|
|
* does, but not when emulating X86_32
|
|
*/
|
|
static unsigned long mmap_legacy_base(void)
|
|
{
|
|
if (mmap_is_ia32())
|
|
return TASK_UNMAPPED_BASE;
|
|
else
|
|
return TASK_UNMAPPED_BASE + mmap_rnd();
|
|
}
|
|
|
|
/*
|
|
* This function, called very early during the creation of a new
|
|
* process VM image, sets up which VM layout function to use:
|
|
*/
|
|
void arch_pick_mmap_layout(struct mm_struct *mm)
|
|
{
|
|
if (mmap_is_legacy()) {
|
|
mm->mmap_base = mmap_legacy_base();
|
|
mm->get_unmapped_area = arch_get_unmapped_area;
|
|
mm->unmap_area = arch_unmap_area;
|
|
} else {
|
|
mm->mmap_base = mmap_base();
|
|
mm->get_unmapped_area = arch_get_unmapped_area_topdown;
|
|
mm->unmap_area = arch_unmap_area_topdown;
|
|
}
|
|
}
|