mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-21 01:51:18 +00:00
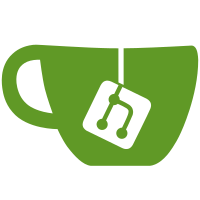
These hooks allows intercepting connect(), getsockname(), getpeername(), sendmsg() and recvmsg() for unix sockets. The unix socket hooks get write access to the address length because the address length is not fixed when dealing with unix sockets and needs to be modified when a unix socket address is modified by the hook. Because abstract socket unix addresses start with a NUL byte, we cannot recalculate the socket address in kernelspace after running the hook by calculating the length of the unix socket path using strlen(). These hooks can be used when users want to multiplex syscall to a single unix socket to multiple different processes behind the scenes by redirecting the connect() and other syscalls to process specific sockets. We do not implement support for intercepting bind() because when using bind() with unix sockets with a pathname address, this creates an inode in the filesystem which must be cleaned up. If we rewrite the address, the user might try to clean up the wrong file, leaking the socket in the filesystem where it is never cleaned up. Until we figure out a solution for this (and a use case for intercepting bind()), we opt to not allow rewriting the sockaddr in bind() calls. We also implement recvmsg() support for connected streams so that after a connect() that is modified by a sockaddr hook, any corresponding recmvsg() on the connected socket can also be modified to make the connected program think it is connected to the "intended" remote. Reviewed-by: Kuniyuki Iwashima <kuniyu@amazon.com> Signed-off-by: Daan De Meyer <daan.j.demeyer@gmail.com> Link: https://lore.kernel.org/r/20231011185113.140426-5-daan.j.demeyer@gmail.com Signed-off-by: Martin KaFai Lau <martin.lau@kernel.org>
84 lines
2.1 KiB
C
84 lines
2.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _BPF_CGROUP_DEFS_H
|
|
#define _BPF_CGROUP_DEFS_H
|
|
|
|
#ifdef CONFIG_CGROUP_BPF
|
|
|
|
#include <linux/list.h>
|
|
#include <linux/percpu-refcount.h>
|
|
#include <linux/workqueue.h>
|
|
|
|
struct bpf_prog_array;
|
|
|
|
#ifdef CONFIG_BPF_LSM
|
|
/* Maximum number of concurrently attachable per-cgroup LSM hooks. */
|
|
#define CGROUP_LSM_NUM 10
|
|
#else
|
|
#define CGROUP_LSM_NUM 0
|
|
#endif
|
|
|
|
enum cgroup_bpf_attach_type {
|
|
CGROUP_BPF_ATTACH_TYPE_INVALID = -1,
|
|
CGROUP_INET_INGRESS = 0,
|
|
CGROUP_INET_EGRESS,
|
|
CGROUP_INET_SOCK_CREATE,
|
|
CGROUP_SOCK_OPS,
|
|
CGROUP_DEVICE,
|
|
CGROUP_INET4_BIND,
|
|
CGROUP_INET6_BIND,
|
|
CGROUP_INET4_CONNECT,
|
|
CGROUP_INET6_CONNECT,
|
|
CGROUP_UNIX_CONNECT,
|
|
CGROUP_INET4_POST_BIND,
|
|
CGROUP_INET6_POST_BIND,
|
|
CGROUP_UDP4_SENDMSG,
|
|
CGROUP_UDP6_SENDMSG,
|
|
CGROUP_UNIX_SENDMSG,
|
|
CGROUP_SYSCTL,
|
|
CGROUP_UDP4_RECVMSG,
|
|
CGROUP_UDP6_RECVMSG,
|
|
CGROUP_UNIX_RECVMSG,
|
|
CGROUP_GETSOCKOPT,
|
|
CGROUP_SETSOCKOPT,
|
|
CGROUP_INET4_GETPEERNAME,
|
|
CGROUP_INET6_GETPEERNAME,
|
|
CGROUP_UNIX_GETPEERNAME,
|
|
CGROUP_INET4_GETSOCKNAME,
|
|
CGROUP_INET6_GETSOCKNAME,
|
|
CGROUP_UNIX_GETSOCKNAME,
|
|
CGROUP_INET_SOCK_RELEASE,
|
|
CGROUP_LSM_START,
|
|
CGROUP_LSM_END = CGROUP_LSM_START + CGROUP_LSM_NUM - 1,
|
|
MAX_CGROUP_BPF_ATTACH_TYPE
|
|
};
|
|
|
|
struct cgroup_bpf {
|
|
/* array of effective progs in this cgroup */
|
|
struct bpf_prog_array __rcu *effective[MAX_CGROUP_BPF_ATTACH_TYPE];
|
|
|
|
/* attached progs to this cgroup and attach flags
|
|
* when flags == 0 or BPF_F_ALLOW_OVERRIDE the progs list will
|
|
* have either zero or one element
|
|
* when BPF_F_ALLOW_MULTI the list can have up to BPF_CGROUP_MAX_PROGS
|
|
*/
|
|
struct hlist_head progs[MAX_CGROUP_BPF_ATTACH_TYPE];
|
|
u8 flags[MAX_CGROUP_BPF_ATTACH_TYPE];
|
|
|
|
/* list of cgroup shared storages */
|
|
struct list_head storages;
|
|
|
|
/* temp storage for effective prog array used by prog_attach/detach */
|
|
struct bpf_prog_array *inactive;
|
|
|
|
/* reference counter used to detach bpf programs after cgroup removal */
|
|
struct percpu_ref refcnt;
|
|
|
|
/* cgroup_bpf is released using a work queue */
|
|
struct work_struct release_work;
|
|
};
|
|
|
|
#else /* CONFIG_CGROUP_BPF */
|
|
struct cgroup_bpf {};
|
|
#endif /* CONFIG_CGROUP_BPF */
|
|
|
|
#endif
|