mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-21 01:51:18 +00:00
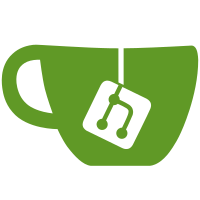
Initial tests with the CXL CPER implementation identified that error reports were being duplicated in the log and the trace event [1]. Then it was discovered that the notification handler took sleeping locks while the GHES event handling runs in spin_lock_irqsave() context [2] While the duplicate reporting was fixed in v6.8-rc4, the fix for the sleeping-lock-vs-atomic collision would enjoy more time to settle and gain some test cycles. Given how late it is in the development cycle, remove the CXL hookup for now and try again during the next merge window. Note that end result is that v6.8 does not emit CXL CPER payloads to the kernel log, but this is in line with the CXL trend to move error reporting to trace events instead of the kernel log. Cc: Ard Biesheuvel <ardb@kernel.org> Cc: Rafael J. Wysocki <rafael@kernel.org> Cc: Jonathan Cameron <Jonathan.Cameron@huawei.com> Reviewed-by: Ira Weiny <ira.weiny@intel.com> Link: http://lore.kernel.org/r/20240108165855.00002f5a@Huawei.com [1] Closes: http://lore.kernel.org/r/b963c490-2c13-4b79-bbe7-34c6568423c7@moroto.mountain [2] Signed-off-by: Dan Williams <dan.j.williams@intel.com>
143 lines
2.9 KiB
C
143 lines
2.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/* Copyright(c) 2023 Intel Corporation. */
|
|
#ifndef _LINUX_CXL_EVENT_H
|
|
#define _LINUX_CXL_EVENT_H
|
|
|
|
/*
|
|
* Common Event Record Format
|
|
* CXL rev 3.0 section 8.2.9.2.1; Table 8-42
|
|
*/
|
|
struct cxl_event_record_hdr {
|
|
u8 length;
|
|
u8 flags[3];
|
|
__le16 handle;
|
|
__le16 related_handle;
|
|
__le64 timestamp;
|
|
u8 maint_op_class;
|
|
u8 reserved[15];
|
|
} __packed;
|
|
|
|
#define CXL_EVENT_RECORD_DATA_LENGTH 0x50
|
|
struct cxl_event_generic {
|
|
struct cxl_event_record_hdr hdr;
|
|
u8 data[CXL_EVENT_RECORD_DATA_LENGTH];
|
|
} __packed;
|
|
|
|
/*
|
|
* General Media Event Record
|
|
* CXL rev 3.0 Section 8.2.9.2.1.1; Table 8-43
|
|
*/
|
|
#define CXL_EVENT_GEN_MED_COMP_ID_SIZE 0x10
|
|
struct cxl_event_gen_media {
|
|
struct cxl_event_record_hdr hdr;
|
|
__le64 phys_addr;
|
|
u8 descriptor;
|
|
u8 type;
|
|
u8 transaction_type;
|
|
u8 validity_flags[2];
|
|
u8 channel;
|
|
u8 rank;
|
|
u8 device[3];
|
|
u8 component_id[CXL_EVENT_GEN_MED_COMP_ID_SIZE];
|
|
u8 reserved[46];
|
|
} __packed;
|
|
|
|
/*
|
|
* DRAM Event Record - DER
|
|
* CXL rev 3.0 section 8.2.9.2.1.2; Table 3-44
|
|
*/
|
|
#define CXL_EVENT_DER_CORRECTION_MASK_SIZE 0x20
|
|
struct cxl_event_dram {
|
|
struct cxl_event_record_hdr hdr;
|
|
__le64 phys_addr;
|
|
u8 descriptor;
|
|
u8 type;
|
|
u8 transaction_type;
|
|
u8 validity_flags[2];
|
|
u8 channel;
|
|
u8 rank;
|
|
u8 nibble_mask[3];
|
|
u8 bank_group;
|
|
u8 bank;
|
|
u8 row[3];
|
|
u8 column[2];
|
|
u8 correction_mask[CXL_EVENT_DER_CORRECTION_MASK_SIZE];
|
|
u8 reserved[0x17];
|
|
} __packed;
|
|
|
|
/*
|
|
* Get Health Info Record
|
|
* CXL rev 3.0 section 8.2.9.8.3.1; Table 8-100
|
|
*/
|
|
struct cxl_get_health_info {
|
|
u8 health_status;
|
|
u8 media_status;
|
|
u8 add_status;
|
|
u8 life_used;
|
|
u8 device_temp[2];
|
|
u8 dirty_shutdown_cnt[4];
|
|
u8 cor_vol_err_cnt[4];
|
|
u8 cor_per_err_cnt[4];
|
|
} __packed;
|
|
|
|
/*
|
|
* Memory Module Event Record
|
|
* CXL rev 3.0 section 8.2.9.2.1.3; Table 8-45
|
|
*/
|
|
struct cxl_event_mem_module {
|
|
struct cxl_event_record_hdr hdr;
|
|
u8 event_type;
|
|
struct cxl_get_health_info info;
|
|
u8 reserved[0x3d];
|
|
} __packed;
|
|
|
|
union cxl_event {
|
|
struct cxl_event_generic generic;
|
|
struct cxl_event_gen_media gen_media;
|
|
struct cxl_event_dram dram;
|
|
struct cxl_event_mem_module mem_module;
|
|
} __packed;
|
|
|
|
/*
|
|
* Common Event Record Format; in event logs
|
|
* CXL rev 3.0 section 8.2.9.2.1; Table 8-42
|
|
*/
|
|
struct cxl_event_record_raw {
|
|
uuid_t id;
|
|
union cxl_event event;
|
|
} __packed;
|
|
|
|
enum cxl_event_type {
|
|
CXL_CPER_EVENT_GENERIC,
|
|
CXL_CPER_EVENT_GEN_MEDIA,
|
|
CXL_CPER_EVENT_DRAM,
|
|
CXL_CPER_EVENT_MEM_MODULE,
|
|
};
|
|
|
|
#define CPER_CXL_DEVICE_ID_VALID BIT(0)
|
|
#define CPER_CXL_DEVICE_SN_VALID BIT(1)
|
|
#define CPER_CXL_COMP_EVENT_LOG_VALID BIT(2)
|
|
struct cxl_cper_event_rec {
|
|
struct {
|
|
u32 length;
|
|
u64 validation_bits;
|
|
struct cper_cxl_event_devid {
|
|
u16 vendor_id;
|
|
u16 device_id;
|
|
u8 func_num;
|
|
u8 device_num;
|
|
u8 bus_num;
|
|
u16 segment_num;
|
|
u16 slot_num; /* bits 2:0 reserved */
|
|
u8 reserved;
|
|
} __packed device_id;
|
|
struct cper_cxl_event_sn {
|
|
u32 lower_dw;
|
|
u32 upper_dw;
|
|
} __packed dev_serial_num;
|
|
} __packed hdr;
|
|
|
|
union cxl_event event;
|
|
} __packed;
|
|
|
|
#endif /* _LINUX_CXL_EVENT_H */
|