mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
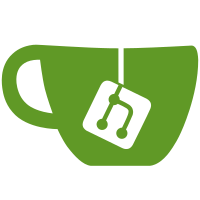
Declare snd_soc_codec_driver structures as const as they are only passed as an argument to the function snd_soc_register_codec. This argument is of type const, so declare the structures with this property as const. In file codecs/sn95031.c, snd_soc_codec_driver structure is also used in a copy operation along with getting passed to snd_soc_register_codec. So, it can be made const too. Done using Coccinelle: @match disable optional_qualifier@ identifier s; position p; @@ static struct snd_soc_codec_driver s@p={...}; @good1@ identifier match.s; position p; @@ snd_soc_register_codec(...,&s@p,...) @bad@ identifier match.s; position p!={match.p,good1.p}; @@ s@p @depends on !bad disable optional_qualifier@ identifier match.s; @@ static +const struct snd_soc_codec_driver s={...}; Signed-off-by: Bhumika Goyal <bhumirks@gmail.com> Signed-off-by: Mark Brown <broonie@kernel.org>
149 lines
3.8 KiB
C
149 lines
3.8 KiB
C
/* Copyright (c) 2010-2011,2013-2015 The Linux Foundation. All rights reserved.
|
|
*
|
|
* This program is free software; you can redistribute it and/or modify
|
|
* it under the terms of the GNU General Public License version 2 and
|
|
* only version 2 as published by the Free Software Foundation.
|
|
*
|
|
* This program is distributed in the hope that it will be useful,
|
|
* but WITHOUT ANY WARRANTY; without even the implied warranty of
|
|
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*
|
|
* max98357a.c -- MAX98357A ALSA SoC Codec driver
|
|
*/
|
|
|
|
#include <linux/acpi.h>
|
|
#include <linux/device.h>
|
|
#include <linux/err.h>
|
|
#include <linux/gpio.h>
|
|
#include <linux/gpio/consumer.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/mod_devicetable.h>
|
|
#include <linux/module.h>
|
|
#include <linux/of.h>
|
|
#include <linux/platform_device.h>
|
|
#include <sound/pcm.h>
|
|
#include <sound/soc.h>
|
|
#include <sound/soc-dai.h>
|
|
#include <sound/soc-dapm.h>
|
|
|
|
static int max98357a_daiops_trigger(struct snd_pcm_substream *substream,
|
|
int cmd, struct snd_soc_dai *dai)
|
|
{
|
|
struct gpio_desc *sdmode = snd_soc_dai_get_drvdata(dai);
|
|
|
|
if (!sdmode)
|
|
return 0;
|
|
|
|
switch (cmd) {
|
|
case SNDRV_PCM_TRIGGER_START:
|
|
case SNDRV_PCM_TRIGGER_RESUME:
|
|
case SNDRV_PCM_TRIGGER_PAUSE_RELEASE:
|
|
gpiod_set_value(sdmode, 1);
|
|
break;
|
|
case SNDRV_PCM_TRIGGER_STOP:
|
|
case SNDRV_PCM_TRIGGER_SUSPEND:
|
|
case SNDRV_PCM_TRIGGER_PAUSE_PUSH:
|
|
gpiod_set_value(sdmode, 0);
|
|
break;
|
|
}
|
|
|
|
return 0;
|
|
}
|
|
|
|
static const struct snd_soc_dapm_widget max98357a_dapm_widgets[] = {
|
|
SND_SOC_DAPM_OUTPUT("Speaker"),
|
|
};
|
|
|
|
static const struct snd_soc_dapm_route max98357a_dapm_routes[] = {
|
|
{"Speaker", NULL, "HiFi Playback"},
|
|
};
|
|
|
|
static int max98357a_codec_probe(struct snd_soc_codec *codec)
|
|
{
|
|
struct gpio_desc *sdmode;
|
|
|
|
sdmode = devm_gpiod_get_optional(codec->dev, "sdmode", GPIOD_OUT_LOW);
|
|
if (IS_ERR(sdmode))
|
|
return PTR_ERR(sdmode);
|
|
|
|
snd_soc_codec_set_drvdata(codec, sdmode);
|
|
|
|
return 0;
|
|
}
|
|
|
|
static const struct snd_soc_codec_driver max98357a_codec_driver = {
|
|
.probe = max98357a_codec_probe,
|
|
.component_driver = {
|
|
.dapm_widgets = max98357a_dapm_widgets,
|
|
.num_dapm_widgets = ARRAY_SIZE(max98357a_dapm_widgets),
|
|
.dapm_routes = max98357a_dapm_routes,
|
|
.num_dapm_routes = ARRAY_SIZE(max98357a_dapm_routes),
|
|
},
|
|
};
|
|
|
|
static const struct snd_soc_dai_ops max98357a_dai_ops = {
|
|
.trigger = max98357a_daiops_trigger,
|
|
};
|
|
|
|
static struct snd_soc_dai_driver max98357a_dai_driver = {
|
|
.name = "HiFi",
|
|
.playback = {
|
|
.stream_name = "HiFi Playback",
|
|
.formats = SNDRV_PCM_FMTBIT_S16 |
|
|
SNDRV_PCM_FMTBIT_S24 |
|
|
SNDRV_PCM_FMTBIT_S32,
|
|
.rates = SNDRV_PCM_RATE_8000 |
|
|
SNDRV_PCM_RATE_16000 |
|
|
SNDRV_PCM_RATE_48000 |
|
|
SNDRV_PCM_RATE_96000,
|
|
.rate_min = 8000,
|
|
.rate_max = 96000,
|
|
.channels_min = 1,
|
|
.channels_max = 2,
|
|
},
|
|
.ops = &max98357a_dai_ops,
|
|
};
|
|
|
|
static int max98357a_platform_probe(struct platform_device *pdev)
|
|
{
|
|
return snd_soc_register_codec(&pdev->dev, &max98357a_codec_driver,
|
|
&max98357a_dai_driver, 1);
|
|
}
|
|
|
|
static int max98357a_platform_remove(struct platform_device *pdev)
|
|
{
|
|
snd_soc_unregister_codec(&pdev->dev);
|
|
|
|
return 0;
|
|
}
|
|
|
|
#ifdef CONFIG_OF
|
|
static const struct of_device_id max98357a_device_id[] = {
|
|
{ .compatible = "maxim,max98357a" },
|
|
{}
|
|
};
|
|
MODULE_DEVICE_TABLE(of, max98357a_device_id);
|
|
#endif
|
|
|
|
#ifdef CONFIG_ACPI
|
|
static const struct acpi_device_id max98357a_acpi_match[] = {
|
|
{ "MX98357A", 0 },
|
|
{},
|
|
};
|
|
MODULE_DEVICE_TABLE(acpi, max98357a_acpi_match);
|
|
#endif
|
|
|
|
static struct platform_driver max98357a_platform_driver = {
|
|
.driver = {
|
|
.name = "max98357a",
|
|
.of_match_table = of_match_ptr(max98357a_device_id),
|
|
.acpi_match_table = ACPI_PTR(max98357a_acpi_match),
|
|
},
|
|
.probe = max98357a_platform_probe,
|
|
.remove = max98357a_platform_remove,
|
|
};
|
|
module_platform_driver(max98357a_platform_driver);
|
|
|
|
MODULE_DESCRIPTION("Maxim MAX98357A Codec Driver");
|
|
MODULE_LICENSE("GPL v2");
|