mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
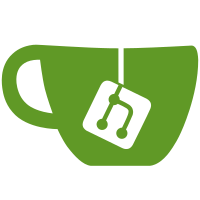
Function show_mem() is used to print system memory status when user requires or fail to allocate memory. Generally, this is a best effort information so any races with memory hotplug (or very theoretically an early initialization) should be tolerable and the worst that could happen is to print an imprecise node state. Drop the resize lock because this is the only place which might hold the lock from the interrupt context and so all other callers might use a simple spinlock. Even though this doesn't solve any real issue it makes the code easier to follow and tiny more effective. Link: http://lkml.kernel.org/r/20181129235532.9328-1-richard.weiyang@gmail.com Signed-off-by: Wei Yang <richard.weiyang@gmail.com> Acked-by: Michal Hocko <mhocko@suse.com> Reviewed-by: Oscar Salvador <osalvador@suse.de> Cc: Johannes Weiner <hannes@cmpxchg.org> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
49 lines
1.2 KiB
C
49 lines
1.2 KiB
C
/*
|
|
* Generic show_mem() implementation
|
|
*
|
|
* Copyright (C) 2008 Johannes Weiner <hannes@saeurebad.de>
|
|
* All code subject to the GPL version 2.
|
|
*/
|
|
|
|
#include <linux/mm.h>
|
|
#include <linux/quicklist.h>
|
|
#include <linux/cma.h>
|
|
|
|
void show_mem(unsigned int filter, nodemask_t *nodemask)
|
|
{
|
|
pg_data_t *pgdat;
|
|
unsigned long total = 0, reserved = 0, highmem = 0;
|
|
|
|
printk("Mem-Info:\n");
|
|
show_free_areas(filter, nodemask);
|
|
|
|
for_each_online_pgdat(pgdat) {
|
|
int zoneid;
|
|
|
|
for (zoneid = 0; zoneid < MAX_NR_ZONES; zoneid++) {
|
|
struct zone *zone = &pgdat->node_zones[zoneid];
|
|
if (!populated_zone(zone))
|
|
continue;
|
|
|
|
total += zone->present_pages;
|
|
reserved += zone->present_pages - zone_managed_pages(zone);
|
|
|
|
if (is_highmem_idx(zoneid))
|
|
highmem += zone->present_pages;
|
|
}
|
|
}
|
|
|
|
printk("%lu pages RAM\n", total);
|
|
printk("%lu pages HighMem/MovableOnly\n", highmem);
|
|
printk("%lu pages reserved\n", reserved);
|
|
#ifdef CONFIG_CMA
|
|
printk("%lu pages cma reserved\n", totalcma_pages);
|
|
#endif
|
|
#ifdef CONFIG_QUICKLIST
|
|
printk("%lu pages in pagetable cache\n",
|
|
quicklist_total_size());
|
|
#endif
|
|
#ifdef CONFIG_MEMORY_FAILURE
|
|
printk("%lu pages hwpoisoned\n", atomic_long_read(&num_poisoned_pages));
|
|
#endif
|
|
}
|