mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-13 14:14:37 +00:00
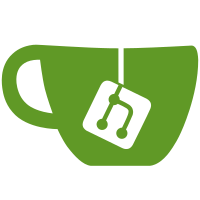
The arch_teardown_msi_irqs() function assumes that controller ops
pointers were already checked in arch_setup_msi_irqs(), but this
assumption is wrong: arch_teardown_msi_irqs() can be called even when
arch_setup_msi_irqs() returns an error (-ENOSYS).
This can happen in the following scenario:
- msi_capability_init() calls pci_msi_setup_msi_irqs()
- pci_msi_setup_msi_irqs() returns -ENOSYS
- msi_capability_init() notices the error and calls free_msi_irqs()
- free_msi_irqs() calls pci_msi_teardown_msi_irqs()
This is easier to see when CONFIG_PCI_MSI_IRQ_DOMAIN is not set and
pci_msi_setup_msi_irqs() and pci_msi_teardown_msi_irqs() are just
aliases to arch_setup_msi_irqs() and arch_teardown_msi_irqs().
The call to free_msi_irqs() upon pci_msi_setup_msi_irqs() failure
seems legit, as it does additional cleanup; e.g.
list_del(&entry->list) and kfree(entry) inside free_msi_irqs() do
happen (MSI descriptors are allocated before pci_msi_setup_msi_irqs()
is called and need to be cleaned up if that fails).
Fixes: 6b2fd7efeb
("PCI/MSI/PPC: Remove arch_msi_check_device()")
Cc: stable@vger.kernel.org # v3.18+
Signed-off-by: Radu Rendec <radu.rendec@gmail.com>
Signed-off-by: Michael Ellerman <mpe@ellerman.id.au>
43 lines
1.2 KiB
C
43 lines
1.2 KiB
C
/*
|
|
* Copyright 2006-2007, Michael Ellerman, IBM Corporation.
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License
|
|
* as published by the Free Software Foundation; either version
|
|
* 2 of the License, or (at your option) any later version.
|
|
*/
|
|
|
|
#include <linux/kernel.h>
|
|
#include <linux/msi.h>
|
|
#include <linux/pci.h>
|
|
|
|
#include <asm/machdep.h>
|
|
|
|
int arch_setup_msi_irqs(struct pci_dev *dev, int nvec, int type)
|
|
{
|
|
struct pci_controller *phb = pci_bus_to_host(dev->bus);
|
|
|
|
if (!phb->controller_ops.setup_msi_irqs ||
|
|
!phb->controller_ops.teardown_msi_irqs) {
|
|
pr_debug("msi: Platform doesn't provide MSI callbacks.\n");
|
|
return -ENOSYS;
|
|
}
|
|
|
|
/* PowerPC doesn't support multiple MSI yet */
|
|
if (type == PCI_CAP_ID_MSI && nvec > 1)
|
|
return 1;
|
|
|
|
return phb->controller_ops.setup_msi_irqs(dev, nvec, type);
|
|
}
|
|
|
|
void arch_teardown_msi_irqs(struct pci_dev *dev)
|
|
{
|
|
struct pci_controller *phb = pci_bus_to_host(dev->bus);
|
|
|
|
/*
|
|
* We can be called even when arch_setup_msi_irqs() returns -ENOSYS,
|
|
* so check the pointer again.
|
|
*/
|
|
if (phb->controller_ops.teardown_msi_irqs)
|
|
phb->controller_ops.teardown_msi_irqs(dev);
|
|
}
|