mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-29 05:44:11 +00:00
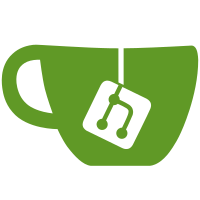
BPF object files are, in a way, the final artifact produced as part of the ahead-of-time compilation process. That makes them somewhat special compared to "regular" object files, which are a intermediate build artifacts that can typically be removed safely. As such, it can make sense to name them differently to make it easier to spot this difference at a glance. Among others, libbpf-bootstrap [0] has established the extension .bpf.o for BPF object files. It seems reasonable to follow this example and establish the same denomination for selftest build artifacts. To that end, this change adjusts the corresponding part of the build system and the test programs loading BPF object files to work with .bpf.o files. [0] https://github.com/libbpf/libbpf-bootstrap Suggested-by: Andrii Nakryiko <andrii@kernel.org> Signed-off-by: Daniel Müller <deso@posteo.net> Signed-off-by: Daniel Borkmann <daniel@iogearbox.net> Link: https://lore.kernel.org/bpf/20220901222253.1199242-1-deso@posteo.net
85 lines
2 KiB
C
85 lines
2 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/* Copyright (c) 2017 Facebook
|
|
*/
|
|
|
|
#include <stdio.h>
|
|
#include <stdlib.h>
|
|
#include <string.h>
|
|
#include <errno.h>
|
|
#include <assert.h>
|
|
#include <sys/time.h>
|
|
|
|
#include <linux/bpf.h>
|
|
#include <bpf/bpf.h>
|
|
#include <bpf/libbpf.h>
|
|
|
|
#include "cgroup_helpers.h"
|
|
#include "testing_helpers.h"
|
|
|
|
#define DEV_CGROUP_PROG "./dev_cgroup.bpf.o"
|
|
|
|
#define TEST_CGROUP "/test-bpf-based-device-cgroup/"
|
|
|
|
int main(int argc, char **argv)
|
|
{
|
|
struct bpf_object *obj;
|
|
int error = EXIT_FAILURE;
|
|
int prog_fd, cgroup_fd;
|
|
__u32 prog_cnt;
|
|
|
|
/* Use libbpf 1.0 API mode */
|
|
libbpf_set_strict_mode(LIBBPF_STRICT_ALL);
|
|
|
|
if (bpf_prog_test_load(DEV_CGROUP_PROG, BPF_PROG_TYPE_CGROUP_DEVICE,
|
|
&obj, &prog_fd)) {
|
|
printf("Failed to load DEV_CGROUP program\n");
|
|
goto out;
|
|
}
|
|
|
|
cgroup_fd = cgroup_setup_and_join(TEST_CGROUP);
|
|
if (cgroup_fd < 0) {
|
|
printf("Failed to create test cgroup\n");
|
|
goto out;
|
|
}
|
|
|
|
/* Attach bpf program */
|
|
if (bpf_prog_attach(prog_fd, cgroup_fd, BPF_CGROUP_DEVICE, 0)) {
|
|
printf("Failed to attach DEV_CGROUP program");
|
|
goto err;
|
|
}
|
|
|
|
if (bpf_prog_query(cgroup_fd, BPF_CGROUP_DEVICE, 0, NULL, NULL,
|
|
&prog_cnt)) {
|
|
printf("Failed to query attached programs");
|
|
goto err;
|
|
}
|
|
|
|
/* All operations with /dev/zero and and /dev/urandom are allowed,
|
|
* everything else is forbidden.
|
|
*/
|
|
assert(system("rm -f /tmp/test_dev_cgroup_null") == 0);
|
|
assert(system("mknod /tmp/test_dev_cgroup_null c 1 3"));
|
|
assert(system("rm -f /tmp/test_dev_cgroup_null") == 0);
|
|
|
|
/* /dev/zero is whitelisted */
|
|
assert(system("rm -f /tmp/test_dev_cgroup_zero") == 0);
|
|
assert(system("mknod /tmp/test_dev_cgroup_zero c 1 5") == 0);
|
|
assert(system("rm -f /tmp/test_dev_cgroup_zero") == 0);
|
|
|
|
assert(system("dd if=/dev/urandom of=/dev/zero count=64") == 0);
|
|
|
|
/* src is allowed, target is forbidden */
|
|
assert(system("dd if=/dev/urandom of=/dev/full count=64"));
|
|
|
|
/* src is forbidden, target is allowed */
|
|
assert(system("dd if=/dev/random of=/dev/zero count=64"));
|
|
|
|
error = 0;
|
|
printf("test_dev_cgroup:PASS\n");
|
|
|
|
err:
|
|
cleanup_cgroup_environment();
|
|
|
|
out:
|
|
return error;
|
|
}
|