mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 17:30:02 +00:00
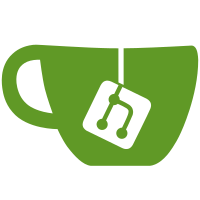
The UAF bug occurred because we were putting DFS root sessions in cifs_umount() while DFS cache refresher was being executed. Make DFS root sessions have same lifetime as DFS tcons so we can avoid the use-after-free bug is DFS cache refresher and other places that require IPCs to get new DFS referrals on. Also, get rid of mount group handling in DFS cache as we no longer need it. This fixes below use-after-free bug catched by KASAN [ 379.946955] BUG: KASAN: use-after-free in __refresh_tcon.isra.0+0x10b/0xc10 [cifs] [ 379.947642] Read of size 8 at addr ffff888018f57030 by task kworker/u4:3/56 [ 379.948096] [ 379.948208] CPU: 0 PID: 56 Comm: kworker/u4:3 Not tainted 6.2.0-rc7-lku #23 [ 379.948661] Hardware name: QEMU Standard PC (Q35 + ICH9, 2009), BIOS rel-1.16.0-0-gd239552-rebuilt.opensuse.org 04/01/2014 [ 379.949368] Workqueue: cifs-dfscache refresh_cache_worker [cifs] [ 379.949942] Call Trace: [ 379.950113] <TASK> [ 379.950260] dump_stack_lvl+0x50/0x67 [ 379.950510] print_report+0x16a/0x48e [ 379.950759] ? __virt_addr_valid+0xd8/0x160 [ 379.951040] ? __phys_addr+0x41/0x80 [ 379.951285] kasan_report+0xdb/0x110 [ 379.951533] ? __refresh_tcon.isra.0+0x10b/0xc10 [cifs] [ 379.952056] ? __refresh_tcon.isra.0+0x10b/0xc10 [cifs] [ 379.952585] __refresh_tcon.isra.0+0x10b/0xc10 [cifs] [ 379.953096] ? __pfx___refresh_tcon.isra.0+0x10/0x10 [cifs] [ 379.953637] ? __pfx___mutex_lock+0x10/0x10 [ 379.953915] ? lock_release+0xb6/0x720 [ 379.954167] ? __pfx_lock_acquire+0x10/0x10 [ 379.954443] ? refresh_cache_worker+0x34e/0x6d0 [cifs] [ 379.954960] ? __pfx_wb_workfn+0x10/0x10 [ 379.955239] refresh_cache_worker+0x4ad/0x6d0 [cifs] [ 379.955755] ? __pfx_refresh_cache_worker+0x10/0x10 [cifs] [ 379.956323] ? __pfx_lock_acquired+0x10/0x10 [ 379.956615] ? read_word_at_a_time+0xe/0x20 [ 379.956898] ? lockdep_hardirqs_on_prepare+0x12/0x220 [ 379.957235] process_one_work+0x535/0x990 [ 379.957509] ? __pfx_process_one_work+0x10/0x10 [ 379.957812] ? lock_acquired+0xb7/0x5f0 [ 379.958069] ? __list_add_valid+0x37/0xd0 [ 379.958341] ? __list_add_valid+0x37/0xd0 [ 379.958611] worker_thread+0x8e/0x630 [ 379.958861] ? __pfx_worker_thread+0x10/0x10 [ 379.959148] kthread+0x17d/0x1b0 [ 379.959369] ? __pfx_kthread+0x10/0x10 [ 379.959630] ret_from_fork+0x2c/0x50 [ 379.959879] </TASK> Signed-off-by: Paulo Alcantara (SUSE) <pc@manguebit.com> Cc: stable@vger.kernel.org # 6.2 Signed-off-by: Steve French <stfrench@microsoft.com>
63 lines
1.7 KiB
C
63 lines
1.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* Copyright (c) 2022 Paulo Alcantara <palcantara@suse.de>
|
|
*/
|
|
|
|
#ifndef _CIFS_DFS_H
|
|
#define _CIFS_DFS_H
|
|
|
|
#include "cifsglob.h"
|
|
#include "fs_context.h"
|
|
#include "cifs_unicode.h"
|
|
|
|
struct dfs_root_ses {
|
|
struct list_head list;
|
|
struct cifs_ses *ses;
|
|
};
|
|
|
|
int dfs_parse_target_referral(const char *full_path, const struct dfs_info3_param *ref,
|
|
struct smb3_fs_context *ctx);
|
|
int dfs_mount_share(struct cifs_mount_ctx *mnt_ctx, bool *isdfs);
|
|
|
|
static inline char *dfs_get_path(struct cifs_sb_info *cifs_sb, const char *path)
|
|
{
|
|
return dfs_cache_canonical_path(path, cifs_sb->local_nls, cifs_remap(cifs_sb));
|
|
}
|
|
|
|
static inline int dfs_get_referral(struct cifs_mount_ctx *mnt_ctx, const char *path,
|
|
struct dfs_info3_param *ref, struct dfs_cache_tgt_list *tl)
|
|
{
|
|
struct smb3_fs_context *ctx = mnt_ctx->fs_ctx;
|
|
struct cifs_sb_info *cifs_sb = mnt_ctx->cifs_sb;
|
|
|
|
return dfs_cache_find(mnt_ctx->xid, ctx->dfs_root_ses, cifs_sb->local_nls,
|
|
cifs_remap(cifs_sb), path, ref, tl);
|
|
}
|
|
|
|
static inline char *dfs_get_automount_devname(struct dentry *dentry, void *page)
|
|
{
|
|
struct cifs_sb_info *cifs_sb = CIFS_SB(dentry->d_sb);
|
|
struct cifs_tcon *tcon = cifs_sb_master_tcon(cifs_sb);
|
|
struct TCP_Server_Info *server = tcon->ses->server;
|
|
|
|
if (unlikely(!server->origin_fullpath))
|
|
return ERR_PTR(-EREMOTE);
|
|
|
|
return __build_path_from_dentry_optional_prefix(dentry, page,
|
|
server->origin_fullpath,
|
|
strlen(server->origin_fullpath),
|
|
true);
|
|
}
|
|
|
|
static inline void dfs_put_root_smb_sessions(struct list_head *head)
|
|
{
|
|
struct dfs_root_ses *root, *tmp;
|
|
|
|
list_for_each_entry_safe(root, tmp, head, list) {
|
|
list_del_init(&root->list);
|
|
cifs_put_smb_ses(root->ses);
|
|
kfree(root);
|
|
}
|
|
}
|
|
|
|
#endif /* _CIFS_DFS_H */
|