mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
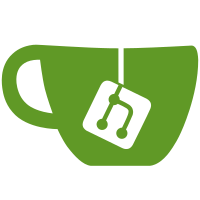
Allow loading of a binary containing i2c scaling sequences to be provided to the wkup_m3 firmware in order to properly scale voltage rails on the PMIC during low power modes like DeepSleep0. Proper binary format is determined by the FW in use. Code expects firmware to have 0x0C57 present as the first two bytes followed by one byte defining offset to sleep sequence followed by one byte defining offset to wake sequence and then lastly both sequences. Each sequence is a series of I2C transfers in the form: u8 length | u8 chip address | u8 byte0/reg address | u8 byte1 | u8 byteN .. The length indicates the number of bytes to transfer, including the register address. The length of each transfer is limited by the I2C buffer size of 32 bytes. Based on previous work by Russ Dill. [dfustini: replace FW_ACTION_HOTPLUG with FW_ACTION_UEVENT] Signed-off-by: Dave Gerlach <d-gerlach@ti.com> Signed-off-by: Keerthy <j-keerthy@ti.com> [dfustini: add NULL argument to rproc_da_to_va() call] Signed-off-by: Drew Fustini <dfustini@baylibre.com> Signed-off-by: Nishanth Menon <nm@ti.com> Link: https://lore.kernel.org/r/20220426200741.712842-3-dfustini@baylibre.com
75 lines
1.9 KiB
C
75 lines
1.9 KiB
C
/*
|
|
* TI Wakeup M3 for AMx3 SoCs Power Management Routines
|
|
*
|
|
* Copyright (C) 2015 Texas Instruments Incorporated - https://www.ti.com/
|
|
* Dave Gerlach <d-gerlach@ti.com>
|
|
*
|
|
* This program is free software; you can redistribute it and/or
|
|
* modify it under the terms of the GNU General Public License as
|
|
* published by the Free Software Foundation version 2.
|
|
*
|
|
* This program is distributed "as is" WITHOUT ANY WARRANTY of any
|
|
* kind, whether express or implied; without even the implied warranty
|
|
* of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
|
|
* GNU General Public License for more details.
|
|
*/
|
|
|
|
#ifndef _LINUX_WKUP_M3_IPC_H
|
|
#define _LINUX_WKUP_M3_IPC_H
|
|
|
|
#define WKUP_M3_DEEPSLEEP 1
|
|
#define WKUP_M3_STANDBY 2
|
|
#define WKUP_M3_IDLE 3
|
|
|
|
#include <linux/mailbox_client.h>
|
|
|
|
struct wkup_m3_ipc_ops;
|
|
|
|
struct wkup_m3_ipc {
|
|
struct rproc *rproc;
|
|
|
|
void __iomem *ipc_mem_base;
|
|
struct device *dev;
|
|
|
|
int mem_type;
|
|
unsigned long resume_addr;
|
|
int vtt_conf;
|
|
int isolation_conf;
|
|
int state;
|
|
|
|
unsigned long volt_scale_offsets;
|
|
const char *sd_fw_name;
|
|
|
|
struct completion sync_complete;
|
|
struct mbox_client mbox_client;
|
|
struct mbox_chan *mbox;
|
|
|
|
struct wkup_m3_ipc_ops *ops;
|
|
int is_rtc_only;
|
|
};
|
|
|
|
struct wkup_m3_wakeup_src {
|
|
int irq_nr;
|
|
char src[10];
|
|
};
|
|
|
|
struct wkup_m3_scale_data_header {
|
|
u16 magic;
|
|
u8 sleep_offset;
|
|
u8 wake_offset;
|
|
} __packed;
|
|
|
|
struct wkup_m3_ipc_ops {
|
|
void (*set_mem_type)(struct wkup_m3_ipc *m3_ipc, int mem_type);
|
|
void (*set_resume_address)(struct wkup_m3_ipc *m3_ipc, void *addr);
|
|
int (*prepare_low_power)(struct wkup_m3_ipc *m3_ipc, int state);
|
|
int (*finish_low_power)(struct wkup_m3_ipc *m3_ipc);
|
|
int (*request_pm_status)(struct wkup_m3_ipc *m3_ipc);
|
|
const char *(*request_wake_src)(struct wkup_m3_ipc *m3_ipc);
|
|
void (*set_rtc_only)(struct wkup_m3_ipc *m3_ipc);
|
|
};
|
|
|
|
struct wkup_m3_ipc *wkup_m3_ipc_get(void);
|
|
void wkup_m3_ipc_put(struct wkup_m3_ipc *m3_ipc);
|
|
void wkup_m3_set_rtc_only_mode(void);
|
|
#endif /* _LINUX_WKUP_M3_IPC_H */
|