mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 05:48:40 +00:00
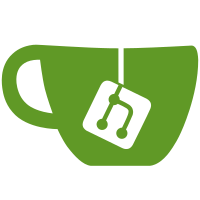
Add a simple helper to check if a file exists based on kernel space file name and switch the early init code over to it. Note that this theoretically changes behavior as it always is based on the effective permissions. But during early init that doesn't make a difference. Signed-off-by: Christoph Hellwig <hch@lst.de>
133 lines
2.9 KiB
C
133 lines
2.9 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* Routines that mimic syscalls, but don't use the user address space or file
|
|
* descriptors. Only for init/ and related early init code.
|
|
*/
|
|
#include <linux/init.h>
|
|
#include <linux/mount.h>
|
|
#include <linux/namei.h>
|
|
#include <linux/fs.h>
|
|
#include <linux/fs_struct.h>
|
|
#include <linux/init_syscalls.h>
|
|
#include <linux/security.h>
|
|
#include "internal.h"
|
|
|
|
int __init init_mount(const char *dev_name, const char *dir_name,
|
|
const char *type_page, unsigned long flags, void *data_page)
|
|
{
|
|
struct path path;
|
|
int ret;
|
|
|
|
ret = kern_path(dir_name, LOOKUP_FOLLOW, &path);
|
|
if (ret)
|
|
return ret;
|
|
ret = path_mount(dev_name, &path, type_page, flags, data_page);
|
|
path_put(&path);
|
|
return ret;
|
|
}
|
|
|
|
int __init init_umount(const char *name, int flags)
|
|
{
|
|
int lookup_flags = LOOKUP_MOUNTPOINT;
|
|
struct path path;
|
|
int ret;
|
|
|
|
if (!(flags & UMOUNT_NOFOLLOW))
|
|
lookup_flags |= LOOKUP_FOLLOW;
|
|
ret = kern_path(name, lookup_flags, &path);
|
|
if (ret)
|
|
return ret;
|
|
return path_umount(&path, flags);
|
|
}
|
|
|
|
int __init init_chdir(const char *filename)
|
|
{
|
|
struct path path;
|
|
int error;
|
|
|
|
error = kern_path(filename, LOOKUP_FOLLOW | LOOKUP_DIRECTORY, &path);
|
|
if (error)
|
|
return error;
|
|
error = inode_permission(path.dentry->d_inode, MAY_EXEC | MAY_CHDIR);
|
|
if (!error)
|
|
set_fs_pwd(current->fs, &path);
|
|
path_put(&path);
|
|
return error;
|
|
}
|
|
|
|
int __init init_chroot(const char *filename)
|
|
{
|
|
struct path path;
|
|
int error;
|
|
|
|
error = kern_path(filename, LOOKUP_FOLLOW | LOOKUP_DIRECTORY, &path);
|
|
if (error)
|
|
return error;
|
|
error = inode_permission(path.dentry->d_inode, MAY_EXEC | MAY_CHDIR);
|
|
if (error)
|
|
goto dput_and_out;
|
|
error = -EPERM;
|
|
if (!ns_capable(current_user_ns(), CAP_SYS_CHROOT))
|
|
goto dput_and_out;
|
|
error = security_path_chroot(&path);
|
|
if (error)
|
|
goto dput_and_out;
|
|
set_fs_root(current->fs, &path);
|
|
dput_and_out:
|
|
path_put(&path);
|
|
return error;
|
|
}
|
|
|
|
int __init init_chown(const char *filename, uid_t user, gid_t group, int flags)
|
|
{
|
|
int lookup_flags = (flags & AT_SYMLINK_NOFOLLOW) ? 0 : LOOKUP_FOLLOW;
|
|
struct path path;
|
|
int error;
|
|
|
|
error = kern_path(filename, lookup_flags, &path);
|
|
if (error)
|
|
return error;
|
|
error = mnt_want_write(path.mnt);
|
|
if (!error) {
|
|
error = chown_common(&path, user, group);
|
|
mnt_drop_write(path.mnt);
|
|
}
|
|
path_put(&path);
|
|
return error;
|
|
}
|
|
|
|
int __init init_chmod(const char *filename, umode_t mode)
|
|
{
|
|
struct path path;
|
|
int error;
|
|
|
|
error = kern_path(filename, LOOKUP_FOLLOW, &path);
|
|
if (error)
|
|
return error;
|
|
error = chmod_common(&path, mode);
|
|
path_put(&path);
|
|
return error;
|
|
}
|
|
|
|
int __init init_eaccess(const char *filename)
|
|
{
|
|
struct path path;
|
|
int error;
|
|
|
|
error = kern_path(filename, LOOKUP_FOLLOW, &path);
|
|
if (error)
|
|
return error;
|
|
error = inode_permission(d_inode(path.dentry), MAY_ACCESS);
|
|
path_put(&path);
|
|
return error;
|
|
}
|
|
|
|
int __init init_unlink(const char *pathname)
|
|
{
|
|
return do_unlinkat(AT_FDCWD, getname_kernel(pathname));
|
|
}
|
|
|
|
int __init init_rmdir(const char *pathname)
|
|
{
|
|
return do_rmdir(AT_FDCWD, getname_kernel(pathname));
|
|
}
|