mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
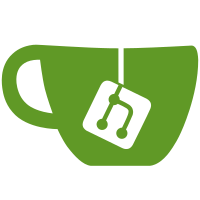
When building ARCH=mips 32r2el_defconfig with CONFIG_UBSAN_ALIGNMENT: ld.lld: error: undefined symbol: __ubsan_handle_alignment_assumption referenced by slab.h:557 (include/linux/slab.h:557) main.o:(do_initcalls) in archive init/built-in.a referenced by slab.h:448 (include/linux/slab.h:448) do_mounts_rd.o:(rd_load_image) in archive init/built-in.a referenced by slab.h:448 (include/linux/slab.h:448) do_mounts_rd.o:(identify_ramdisk_image) in archive init/built-in.a referenced 1579 more times Implement this for the kernel based on LLVM's handleAlignmentAssumptionImpl because the kernel is not linked against the compiler runtime. Link: https://github.com/ClangBuiltLinux/linux/issues/1245 Link: https://github.com/llvm/llvm-project/blob/llvmorg-11.0.1/compiler-rt/lib/ubsan/ubsan_handlers.cpp#L151-L190 Link: https://lkml.kernel.org/r/20210127224451.2587372-1-nathan@kernel.org Signed-off-by: Nathan Chancellor <nathan@kernel.org> Acked-by: Kees Cook <keescook@chromium.org> Reviewed-by: Nick Desaulniers <ndesaulniers@google.com> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
95 lines
1.8 KiB
C
95 lines
1.8 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _LIB_UBSAN_H
|
|
#define _LIB_UBSAN_H
|
|
|
|
enum {
|
|
type_kind_int = 0,
|
|
type_kind_float = 1,
|
|
type_unknown = 0xffff
|
|
};
|
|
|
|
struct type_descriptor {
|
|
u16 type_kind;
|
|
u16 type_info;
|
|
char type_name[1];
|
|
};
|
|
|
|
struct source_location {
|
|
const char *file_name;
|
|
union {
|
|
unsigned long reported;
|
|
struct {
|
|
u32 line;
|
|
u32 column;
|
|
};
|
|
};
|
|
};
|
|
|
|
struct overflow_data {
|
|
struct source_location location;
|
|
struct type_descriptor *type;
|
|
};
|
|
|
|
struct type_mismatch_data {
|
|
struct source_location location;
|
|
struct type_descriptor *type;
|
|
unsigned long alignment;
|
|
unsigned char type_check_kind;
|
|
};
|
|
|
|
struct type_mismatch_data_v1 {
|
|
struct source_location location;
|
|
struct type_descriptor *type;
|
|
unsigned char log_alignment;
|
|
unsigned char type_check_kind;
|
|
};
|
|
|
|
struct type_mismatch_data_common {
|
|
struct source_location *location;
|
|
struct type_descriptor *type;
|
|
unsigned long alignment;
|
|
unsigned char type_check_kind;
|
|
};
|
|
|
|
struct nonnull_arg_data {
|
|
struct source_location location;
|
|
struct source_location attr_location;
|
|
int arg_index;
|
|
};
|
|
|
|
struct out_of_bounds_data {
|
|
struct source_location location;
|
|
struct type_descriptor *array_type;
|
|
struct type_descriptor *index_type;
|
|
};
|
|
|
|
struct shift_out_of_bounds_data {
|
|
struct source_location location;
|
|
struct type_descriptor *lhs_type;
|
|
struct type_descriptor *rhs_type;
|
|
};
|
|
|
|
struct unreachable_data {
|
|
struct source_location location;
|
|
};
|
|
|
|
struct invalid_value_data {
|
|
struct source_location location;
|
|
struct type_descriptor *type;
|
|
};
|
|
|
|
struct alignment_assumption_data {
|
|
struct source_location location;
|
|
struct source_location assumption_location;
|
|
struct type_descriptor *type;
|
|
};
|
|
|
|
#if defined(CONFIG_ARCH_SUPPORTS_INT128)
|
|
typedef __int128 s_max;
|
|
typedef unsigned __int128 u_max;
|
|
#else
|
|
typedef s64 s_max;
|
|
typedef u64 u_max;
|
|
#endif
|
|
|
|
#endif
|