mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-23 09:19:51 +00:00
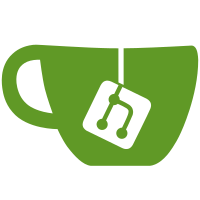
The userspace governor is still in use on production systems and the
deprecating warning is scary.
Even if we want to get rid of the userspace governor, it is too soon
yet as the alternatives are not yet adopted.
Change the deprecated warning by an information message suggesting to
switch to the netlink thermal events.
Fixes: 0275c9fb0e
("thermal/core: Make the userspace governor deprecated")
Signed-off-by: Daniel Lezcano <daniel.lezcano@linaro.org>
Signed-off-by: Rafael J. Wysocki <rafael.j.wysocki@intel.com>
55 lines
1.5 KiB
C
55 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0-only
|
|
/*
|
|
* user_space.c - A simple user space Thermal events notifier
|
|
*
|
|
* Copyright (C) 2012 Intel Corp
|
|
* Copyright (C) 2012 Durgadoss R <durgadoss.r@intel.com>
|
|
*
|
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
*
|
|
* ~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~~
|
|
*/
|
|
|
|
#include <linux/slab.h>
|
|
#include <linux/thermal.h>
|
|
|
|
#include "thermal_core.h"
|
|
|
|
static int user_space_bind(struct thermal_zone_device *tz)
|
|
{
|
|
pr_info_once("Consider using thermal netlink events interface\n");
|
|
|
|
return 0;
|
|
}
|
|
|
|
/**
|
|
* notify_user_space - Notifies user space about thermal events
|
|
* @tz: thermal_zone_device
|
|
* @trip: trip point index
|
|
*
|
|
* This function notifies the user space through UEvents.
|
|
*/
|
|
static int notify_user_space(struct thermal_zone_device *tz, int trip)
|
|
{
|
|
char *thermal_prop[5];
|
|
int i;
|
|
|
|
mutex_lock(&tz->lock);
|
|
thermal_prop[0] = kasprintf(GFP_KERNEL, "NAME=%s", tz->type);
|
|
thermal_prop[1] = kasprintf(GFP_KERNEL, "TEMP=%d", tz->temperature);
|
|
thermal_prop[2] = kasprintf(GFP_KERNEL, "TRIP=%d", trip);
|
|
thermal_prop[3] = kasprintf(GFP_KERNEL, "EVENT=%d", tz->notify_event);
|
|
thermal_prop[4] = NULL;
|
|
kobject_uevent_env(&tz->device.kobj, KOBJ_CHANGE, thermal_prop);
|
|
for (i = 0; i < 4; ++i)
|
|
kfree(thermal_prop[i]);
|
|
mutex_unlock(&tz->lock);
|
|
return 0;
|
|
}
|
|
|
|
static struct thermal_governor thermal_gov_user_space = {
|
|
.name = "user_space",
|
|
.throttle = notify_user_space,
|
|
.bind_to_tz = user_space_bind,
|
|
};
|
|
THERMAL_GOVERNOR_DECLARE(thermal_gov_user_space);
|