mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
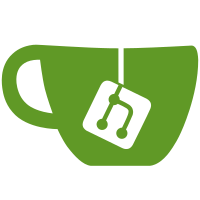
Next record calucaltion can be reduced to a much more tivial ALIGN operation as follows: 1. Splitting 5 into 2 + 3 we get next = ((be16_to_cpu(rec->len) + 2 + 3) & ~3) - 2 (1) 2. Using ALIGN macro we reduce (1) to: ALIGN(be16_to_cpu(rec->len) + 2, 4) - 2 (2) 3. Subsituting 'next' in original next record calucation we get: (void *)&rec->data[ALIGN(be16_to_cpu(rec->len) + 2, 4) - 2] (3) 4. Converting array index to pointer arithmetic we convert (3) into: (void *)rec + sizeof(*rec) + ALIGN(be16_to_cpu(rec->len) + 2, 4) - 2 (4) 5. Subsituting sizeof(*rec) with its value, 6, and substracting 2, in (4) we get: (void *)rec + ALIGN(be16_to_cpu(rec->len) + 2, 4) + 4 (5) 6. Since ALIGN(X, 4) + 4 == ALIGN(X + 4, 4), (5) can be converted to: (void *)rec + ALIGN(be16_to_cpu(rec->len) + 6, 4) (6) 5. Subsituting 6 in (6) to sizeof(*rec) we get: (void *)rec + ALIGN(be16_to_cpu(rec->len) + sizeof(*rec), 4) (7) Using expression (7) should make it more clear that next record is located by adding full size of the current record (payload + auxiliary data) aligned to 4 bytes, to the location of the current one. No functional change intended. Cc: Chris Healy <cphealy@gmail.com> Cc: Kyle McMartin <kyle@kernel.org> Cc: Andrew Morton <akpm@linux-foundation.org> Cc: Masahiro Yamada <yamada.masahiro@socionext.com> Cc: David Woodhouse <dwmw2@infradead.org> Cc: Greg Kroah-Hartman <gregkh@linuxfoundation.org> Cc: linux-kernel <linux-kernel@vger.kernel.org> Signed-off-by: Andrey Smirnov <andrew.smirnov@gmail.com> Signed-off-by: Greg Kroah-Hartman <gregkh@linuxfoundation.org>
84 lines
2.2 KiB
C
84 lines
2.2 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* Compact binary representation of ihex records. Some devices need their
|
|
* firmware loaded in strange orders rather than a single big blob, but
|
|
* actually parsing ihex-as-text within the kernel seems silly. Thus,...
|
|
*/
|
|
|
|
#ifndef __LINUX_IHEX_H__
|
|
#define __LINUX_IHEX_H__
|
|
|
|
#include <linux/types.h>
|
|
#include <linux/firmware.h>
|
|
#include <linux/device.h>
|
|
|
|
/* Intel HEX files actually limit the length to 256 bytes, but we have
|
|
drivers which would benefit from using separate records which are
|
|
longer than that, so we extend to 16 bits of length */
|
|
struct ihex_binrec {
|
|
__be32 addr;
|
|
__be16 len;
|
|
uint8_t data[0];
|
|
} __attribute__((packed));
|
|
|
|
static inline uint16_t ihex_binrec_size(const struct ihex_binrec *p)
|
|
{
|
|
return be16_to_cpu(p->len) + sizeof(*p);
|
|
}
|
|
|
|
/* Find the next record, taking into account the 4-byte alignment */
|
|
static inline const struct ihex_binrec *
|
|
__ihex_next_binrec(const struct ihex_binrec *rec)
|
|
{
|
|
const void *p = rec;
|
|
|
|
return p + ALIGN(ihex_binrec_size(rec), 4);
|
|
}
|
|
|
|
static inline const struct ihex_binrec *
|
|
ihex_next_binrec(const struct ihex_binrec *rec)
|
|
{
|
|
rec = __ihex_next_binrec(rec);
|
|
|
|
return be16_to_cpu(rec->len) ? rec : NULL;
|
|
}
|
|
|
|
/* Check that ihex_next_binrec() won't take us off the end of the image... */
|
|
static inline int ihex_validate_fw(const struct firmware *fw)
|
|
{
|
|
const struct ihex_binrec *end, *rec;
|
|
|
|
rec = (const void *)fw->data;
|
|
end = (const void *)&fw->data[fw->size - sizeof(*end)];
|
|
|
|
for (; rec <= end; rec = __ihex_next_binrec(rec)) {
|
|
/* Zero length marks end of records */
|
|
if (rec == end && !be16_to_cpu(rec->len))
|
|
return 0;
|
|
}
|
|
return -EINVAL;
|
|
}
|
|
|
|
/* Request firmware and validate it so that we can trust we won't
|
|
* run off the end while reading records... */
|
|
static inline int request_ihex_firmware(const struct firmware **fw,
|
|
const char *fw_name,
|
|
struct device *dev)
|
|
{
|
|
const struct firmware *lfw;
|
|
int ret;
|
|
|
|
ret = request_firmware(&lfw, fw_name, dev);
|
|
if (ret)
|
|
return ret;
|
|
ret = ihex_validate_fw(lfw);
|
|
if (ret) {
|
|
dev_err(dev, "Firmware \"%s\" not valid IHEX records\n",
|
|
fw_name);
|
|
release_firmware(lfw);
|
|
return ret;
|
|
}
|
|
*fw = lfw;
|
|
return 0;
|
|
}
|
|
#endif /* __LINUX_IHEX_H__ */
|