mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-22 17:01:14 +00:00
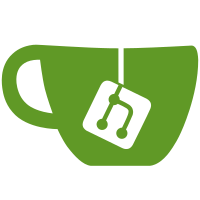
If the probe of an fb driver has been deferred due to missing dependencies, and the probe is later ran when a module is loaded, the fbdev framework will try to find a logo to use. However, the logos are __initdata, and have already been freed. This causes sometimes page faults, if the logo memory is not mapped, sometimes other random crashes as the logo data is invalid, and sometimes nothing, if the fbdev decides to reject the logo (e.g. the random value depicting the logo's height is too big). This patch adds a late_initcall function to mark the logos as freed. In reality the logos are freed later, and fbdev probe may be ran between this late_initcall and the freeing of the logos. In that case we will miss drawing the logo, even if it would be possible. Signed-off-by: Tomi Valkeinen <tomi.valkeinen@ti.com> Cc: stable@vger.kernel.org
117 lines
2.6 KiB
C
117 lines
2.6 KiB
C
|
|
/*
|
|
* Linux logo to be displayed on boot
|
|
*
|
|
* Copyright (C) 1996 Larry Ewing (lewing@isc.tamu.edu)
|
|
* Copyright (C) 1996,1998 Jakub Jelinek (jj@sunsite.mff.cuni.cz)
|
|
* Copyright (C) 2001 Greg Banks <gnb@alphalink.com.au>
|
|
* Copyright (C) 2001 Jan-Benedict Glaw <jbglaw@lug-owl.de>
|
|
* Copyright (C) 2003 Geert Uytterhoeven <geert@linux-m68k.org>
|
|
*/
|
|
|
|
#include <linux/linux_logo.h>
|
|
#include <linux/stddef.h>
|
|
#include <linux/module.h>
|
|
|
|
#ifdef CONFIG_M68K
|
|
#include <asm/setup.h>
|
|
#endif
|
|
|
|
static bool nologo;
|
|
module_param(nologo, bool, 0);
|
|
MODULE_PARM_DESC(nologo, "Disables startup logo");
|
|
|
|
/*
|
|
* Logos are located in the initdata, and will be freed in kernel_init.
|
|
* Use late_init to mark the logos as freed to prevent any further use.
|
|
*/
|
|
|
|
static bool logos_freed;
|
|
|
|
static int __init fb_logo_late_init(void)
|
|
{
|
|
logos_freed = true;
|
|
return 0;
|
|
}
|
|
|
|
late_initcall(fb_logo_late_init);
|
|
|
|
/* logo's are marked __initdata. Use __init_refok to tell
|
|
* modpost that it is intended that this function uses data
|
|
* marked __initdata.
|
|
*/
|
|
const struct linux_logo * __init_refok fb_find_logo(int depth)
|
|
{
|
|
const struct linux_logo *logo = NULL;
|
|
|
|
if (nologo || logos_freed)
|
|
return NULL;
|
|
|
|
if (depth >= 1) {
|
|
#ifdef CONFIG_LOGO_LINUX_MONO
|
|
/* Generic Linux logo */
|
|
logo = &logo_linux_mono;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_SUPERH_MONO
|
|
/* SuperH Linux logo */
|
|
logo = &logo_superh_mono;
|
|
#endif
|
|
}
|
|
|
|
if (depth >= 4) {
|
|
#ifdef CONFIG_LOGO_LINUX_VGA16
|
|
/* Generic Linux logo */
|
|
logo = &logo_linux_vga16;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_BLACKFIN_VGA16
|
|
/* Blackfin processor logo */
|
|
logo = &logo_blackfin_vga16;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_SUPERH_VGA16
|
|
/* SuperH Linux logo */
|
|
logo = &logo_superh_vga16;
|
|
#endif
|
|
}
|
|
|
|
if (depth >= 8) {
|
|
#ifdef CONFIG_LOGO_LINUX_CLUT224
|
|
/* Generic Linux logo */
|
|
logo = &logo_linux_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_BLACKFIN_CLUT224
|
|
/* Blackfin Linux logo */
|
|
logo = &logo_blackfin_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_DEC_CLUT224
|
|
/* DEC Linux logo on MIPS/MIPS64 or ALPHA */
|
|
logo = &logo_dec_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_MAC_CLUT224
|
|
/* Macintosh Linux logo on m68k */
|
|
if (MACH_IS_MAC)
|
|
logo = &logo_mac_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_PARISC_CLUT224
|
|
/* PA-RISC Linux logo */
|
|
logo = &logo_parisc_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_SGI_CLUT224
|
|
/* SGI Linux logo on MIPS/MIPS64 */
|
|
logo = &logo_sgi_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_SUN_CLUT224
|
|
/* Sun Linux logo */
|
|
logo = &logo_sun_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_SUPERH_CLUT224
|
|
/* SuperH Linux logo */
|
|
logo = &logo_superh_clut224;
|
|
#endif
|
|
#ifdef CONFIG_LOGO_M32R_CLUT224
|
|
/* M32R Linux logo */
|
|
logo = &logo_m32r_clut224;
|
|
#endif
|
|
}
|
|
return logo;
|
|
}
|
|
EXPORT_SYMBOL_GPL(fb_find_logo);
|