mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-27 19:29:37 +00:00
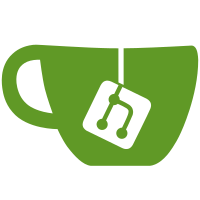
In relocation, we need to locate all parent tree leaves referring to one data extent, thus we have a complex mechanism to iterate throught extent tree and subvolume trees to locate the related leaves. However this is already done in backref.c, we have btrfs_find_all_leafs(), which can return a ulist containing all leaves referring to that data extent. Use btrfs_find_all_leafs() to replace find_data_references(). There is a special handling for v1 space cache data extents, where we need to delete the v1 space cache data extents, to avoid those data extents to hang the data relocation. In this patch, the special handling is done by re-iterating the root tree leaf. Although it's a little less efficient than the old handling, considering we can reuse a lot of code, it should be acceptable. Signed-off-by: Qu Wenruo <wqu@suse.com> Reviewed-by: David Sterba <dsterba@suse.com> Signed-off-by: David Sterba <dsterba@suse.com>
81 lines
2.5 KiB
C
81 lines
2.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
/*
|
|
* Copyright (C) 2011 STRATO. All rights reserved.
|
|
*/
|
|
|
|
#ifndef BTRFS_BACKREF_H
|
|
#define BTRFS_BACKREF_H
|
|
|
|
#include <linux/btrfs.h>
|
|
#include "ulist.h"
|
|
#include "extent_io.h"
|
|
|
|
struct inode_fs_paths {
|
|
struct btrfs_path *btrfs_path;
|
|
struct btrfs_root *fs_root;
|
|
struct btrfs_data_container *fspath;
|
|
};
|
|
|
|
typedef int (iterate_extent_inodes_t)(u64 inum, u64 offset, u64 root,
|
|
void *ctx);
|
|
|
|
int extent_from_logical(struct btrfs_fs_info *fs_info, u64 logical,
|
|
struct btrfs_path *path, struct btrfs_key *found_key,
|
|
u64 *flags);
|
|
|
|
int tree_backref_for_extent(unsigned long *ptr, struct extent_buffer *eb,
|
|
struct btrfs_key *key, struct btrfs_extent_item *ei,
|
|
u32 item_size, u64 *out_root, u8 *out_level);
|
|
|
|
int iterate_extent_inodes(struct btrfs_fs_info *fs_info,
|
|
u64 extent_item_objectid,
|
|
u64 extent_offset, int search_commit_root,
|
|
iterate_extent_inodes_t *iterate, void *ctx,
|
|
bool ignore_offset);
|
|
|
|
int iterate_inodes_from_logical(u64 logical, struct btrfs_fs_info *fs_info,
|
|
struct btrfs_path *path,
|
|
iterate_extent_inodes_t *iterate, void *ctx,
|
|
bool ignore_offset);
|
|
|
|
int paths_from_inode(u64 inum, struct inode_fs_paths *ipath);
|
|
|
|
int btrfs_find_all_leafs(struct btrfs_trans_handle *trans,
|
|
struct btrfs_fs_info *fs_info, u64 bytenr,
|
|
u64 time_seq, struct ulist **leafs,
|
|
const u64 *extent_item_pos, bool ignore_offset);
|
|
int btrfs_find_all_roots(struct btrfs_trans_handle *trans,
|
|
struct btrfs_fs_info *fs_info, u64 bytenr,
|
|
u64 time_seq, struct ulist **roots, bool ignore_offset);
|
|
char *btrfs_ref_to_path(struct btrfs_root *fs_root, struct btrfs_path *path,
|
|
u32 name_len, unsigned long name_off,
|
|
struct extent_buffer *eb_in, u64 parent,
|
|
char *dest, u32 size);
|
|
|
|
struct btrfs_data_container *init_data_container(u32 total_bytes);
|
|
struct inode_fs_paths *init_ipath(s32 total_bytes, struct btrfs_root *fs_root,
|
|
struct btrfs_path *path);
|
|
void free_ipath(struct inode_fs_paths *ipath);
|
|
|
|
int btrfs_find_one_extref(struct btrfs_root *root, u64 inode_objectid,
|
|
u64 start_off, struct btrfs_path *path,
|
|
struct btrfs_inode_extref **ret_extref,
|
|
u64 *found_off);
|
|
int btrfs_check_shared(struct btrfs_root *root, u64 inum, u64 bytenr,
|
|
struct ulist *roots, struct ulist *tmp_ulist);
|
|
|
|
int __init btrfs_prelim_ref_init(void);
|
|
void __cold btrfs_prelim_ref_exit(void);
|
|
|
|
struct prelim_ref {
|
|
struct rb_node rbnode;
|
|
u64 root_id;
|
|
struct btrfs_key key_for_search;
|
|
int level;
|
|
int count;
|
|
struct extent_inode_elem *inode_list;
|
|
u64 parent;
|
|
u64 wanted_disk_byte;
|
|
};
|
|
|
|
#endif
|