mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
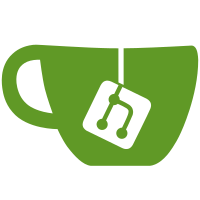
David Rientjes has reported the following memory corruption while the
oom reaper tries to unmap the victims address space
BUG: Bad page map in process oom_reaper pte:6353826300000000 pmd:00000000
addr:00007f50cab1d000 vm_flags:08100073 anon_vma:ffff9eea335603f0 mapping: (null) index:7f50cab1d
file: (null) fault: (null) mmap: (null) readpage: (null)
CPU: 2 PID: 1001 Comm: oom_reaper
Call Trace:
unmap_page_range+0x1068/0x1130
__oom_reap_task_mm+0xd5/0x16b
oom_reaper+0xff/0x14c
kthread+0xc1/0xe0
Tetsuo Handa has noticed that the synchronization inside exit_mmap is
insufficient. We only synchronize with the oom reaper if
tsk_is_oom_victim which is not true if the final __mmput is called from
a different context than the oom victim exit path. This can trivially
happen from context of any task which has grabbed mm reference (e.g. to
read /proc/<pid>/ file which requires mm etc.).
The race would look like this
oom_reaper oom_victim task
mmget_not_zero
do_exit
mmput
__oom_reap_task_mm mmput
__mmput
exit_mmap
remove_vma
unmap_page_range
Fix this issue by providing a new mm_is_oom_victim() helper which
operates on the mm struct rather than a task. Any context which
operates on a remote mm struct should use this helper in place of
tsk_is_oom_victim. The flag is set in mark_oom_victim and never cleared
so it is stable in the exit_mmap path.
Debugged by Tetsuo Handa.
Link: http://lkml.kernel.org/r/20171210095130.17110-1-mhocko@kernel.org
Fixes: 2129258024
("mm: oom: let oom_reap_task and exit_mmap run concurrently")
Signed-off-by: Michal Hocko <mhocko@suse.com>
Reported-by: David Rientjes <rientjes@google.com>
Acked-by: David Rientjes <rientjes@google.com>
Cc: Tetsuo Handa <penguin-kernel@I-love.SAKURA.ne.jp>
Cc: Andrea Argangeli <andrea@kernel.org>
Cc: <stable@vger.kernel.org> [4.14]
Signed-off-by: Andrew Morton <akpm@linux-foundation.org>
Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
118 lines
3.1 KiB
C
118 lines
3.1 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __INCLUDE_LINUX_OOM_H
|
|
#define __INCLUDE_LINUX_OOM_H
|
|
|
|
|
|
#include <linux/sched/signal.h>
|
|
#include <linux/types.h>
|
|
#include <linux/nodemask.h>
|
|
#include <uapi/linux/oom.h>
|
|
#include <linux/sched/coredump.h> /* MMF_* */
|
|
#include <linux/mm.h> /* VM_FAULT* */
|
|
|
|
struct zonelist;
|
|
struct notifier_block;
|
|
struct mem_cgroup;
|
|
struct task_struct;
|
|
|
|
/*
|
|
* Details of the page allocation that triggered the oom killer that are used to
|
|
* determine what should be killed.
|
|
*/
|
|
struct oom_control {
|
|
/* Used to determine cpuset */
|
|
struct zonelist *zonelist;
|
|
|
|
/* Used to determine mempolicy */
|
|
nodemask_t *nodemask;
|
|
|
|
/* Memory cgroup in which oom is invoked, or NULL for global oom */
|
|
struct mem_cgroup *memcg;
|
|
|
|
/* Used to determine cpuset and node locality requirement */
|
|
const gfp_t gfp_mask;
|
|
|
|
/*
|
|
* order == -1 means the oom kill is required by sysrq, otherwise only
|
|
* for display purposes.
|
|
*/
|
|
const int order;
|
|
|
|
/* Used by oom implementation, do not set */
|
|
unsigned long totalpages;
|
|
struct task_struct *chosen;
|
|
unsigned long chosen_points;
|
|
};
|
|
|
|
extern struct mutex oom_lock;
|
|
|
|
static inline void set_current_oom_origin(void)
|
|
{
|
|
current->signal->oom_flag_origin = true;
|
|
}
|
|
|
|
static inline void clear_current_oom_origin(void)
|
|
{
|
|
current->signal->oom_flag_origin = false;
|
|
}
|
|
|
|
static inline bool oom_task_origin(const struct task_struct *p)
|
|
{
|
|
return p->signal->oom_flag_origin;
|
|
}
|
|
|
|
static inline bool tsk_is_oom_victim(struct task_struct * tsk)
|
|
{
|
|
return tsk->signal->oom_mm;
|
|
}
|
|
|
|
/*
|
|
* Use this helper if tsk->mm != mm and the victim mm needs a special
|
|
* handling. This is guaranteed to stay true after once set.
|
|
*/
|
|
static inline bool mm_is_oom_victim(struct mm_struct *mm)
|
|
{
|
|
return test_bit(MMF_OOM_VICTIM, &mm->flags);
|
|
}
|
|
|
|
/*
|
|
* Checks whether a page fault on the given mm is still reliable.
|
|
* This is no longer true if the oom reaper started to reap the
|
|
* address space which is reflected by MMF_UNSTABLE flag set in
|
|
* the mm. At that moment any !shared mapping would lose the content
|
|
* and could cause a memory corruption (zero pages instead of the
|
|
* original content).
|
|
*
|
|
* User should call this before establishing a page table entry for
|
|
* a !shared mapping and under the proper page table lock.
|
|
*
|
|
* Return 0 when the PF is safe VM_FAULT_SIGBUS otherwise.
|
|
*/
|
|
static inline int check_stable_address_space(struct mm_struct *mm)
|
|
{
|
|
if (unlikely(test_bit(MMF_UNSTABLE, &mm->flags)))
|
|
return VM_FAULT_SIGBUS;
|
|
return 0;
|
|
}
|
|
|
|
extern unsigned long oom_badness(struct task_struct *p,
|
|
struct mem_cgroup *memcg, const nodemask_t *nodemask,
|
|
unsigned long totalpages);
|
|
|
|
extern bool out_of_memory(struct oom_control *oc);
|
|
|
|
extern void exit_oom_victim(void);
|
|
|
|
extern int register_oom_notifier(struct notifier_block *nb);
|
|
extern int unregister_oom_notifier(struct notifier_block *nb);
|
|
|
|
extern bool oom_killer_disable(signed long timeout);
|
|
extern void oom_killer_enable(void);
|
|
|
|
extern struct task_struct *find_lock_task_mm(struct task_struct *p);
|
|
|
|
/* sysctls */
|
|
extern int sysctl_oom_dump_tasks;
|
|
extern int sysctl_oom_kill_allocating_task;
|
|
extern int sysctl_panic_on_oom;
|
|
#endif /* _INCLUDE_LINUX_OOM_H */
|