mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 10:49:33 +00:00
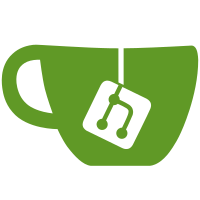
Changes from v1: * exported mpi_sub and mpi_mul, otherwise the build fails when RSA is a module The kernel RSA ASN.1 private key parser already supports only private keys with additional values to be used with the Chinese Remainder Theorem [1], but these values are currently not used. This rudimentary CRT implementation speeds up RSA private key operations for the following Go benchmark up to ~3x. This implementation also tries to minimise the allocation of additional MPIs, so existing MPIs are reused as much as possible (hence the variable names are a bit weird). The benchmark used: ``` package keyring_test import ( "crypto" "crypto/rand" "crypto/rsa" "crypto/x509" "io" "syscall" "testing" "unsafe" ) type KeySerial int32 type Keyring int32 const ( KEY_SPEC_PROCESS_KEYRING Keyring = -2 KEYCTL_PKEY_SIGN = 27 ) var ( keyTypeAsym = []byte("asymmetric\x00") sha256pkcs1 = []byte("enc=pkcs1 hash=sha256\x00") ) func (keyring Keyring) LoadAsym(desc string, payload []byte) (KeySerial, error) { cdesc := []byte(desc + "\x00") serial, _, errno := syscall.Syscall6(syscall.SYS_ADD_KEY, uintptr(unsafe.Pointer(&keyTypeAsym[0])), uintptr(unsafe.Pointer(&cdesc[0])), uintptr(unsafe.Pointer(&payload[0])), uintptr(len(payload)), uintptr(keyring), uintptr(0)) if errno == 0 { return KeySerial(serial), nil } return KeySerial(serial), errno } type pkeyParams struct { key_id KeySerial in_len uint32 out_or_in2_len uint32 __spare [7]uint32 } // the output signature buffer is an input parameter here, because we want to // avoid Go buffer allocation leaking into our benchmarks func (key KeySerial) Sign(info, digest, out []byte) error { var params pkeyParams params.key_id = key params.in_len = uint32(len(digest)) params.out_or_in2_len = uint32(len(out)) _, _, errno := syscall.Syscall6(syscall.SYS_KEYCTL, KEYCTL_PKEY_SIGN, uintptr(unsafe.Pointer(¶ms)), uintptr(unsafe.Pointer(&info[0])), uintptr(unsafe.Pointer(&digest[0])), uintptr(unsafe.Pointer(&out[0])), uintptr(0)) if errno == 0 { return nil } return errno } func BenchmarkSign(b *testing.B) { priv, err := rsa.GenerateKey(rand.Reader, 2048) if err != nil { b.Fatalf("failed to generate private key: %v", err) } pkcs8, err := x509.MarshalPKCS8PrivateKey(priv) if err != nil { b.Fatalf("failed to serialize the private key to PKCS8 blob: %v", err) } serial, err := KEY_SPEC_PROCESS_KEYRING.LoadAsym("test rsa key", pkcs8) if err != nil { b.Fatalf("failed to load the private key into the keyring: %v", err) } b.Logf("loaded test rsa key: %v", serial) digest := make([]byte, 32) _, err = io.ReadFull(rand.Reader, digest) if err != nil { b.Fatalf("failed to generate a random digest: %v", err) } sig := make([]byte, 256) for n := 0; n < b.N; n++ { err = serial.Sign(sha256pkcs1, digest, sig) if err != nil { b.Fatalf("failed to sign the digest: %v", err) } } err = rsa.VerifyPKCS1v15(&priv.PublicKey, crypto.SHA256, digest, sig) if err != nil { b.Fatalf("failed to verify the signature: %v", err) } } ``` [1]: https://en.wikipedia.org/wiki/RSA_(cryptosystem)#Using_the_Chinese_remainder_algorithm Signed-off-by: Ignat Korchagin <ignat@cloudflare.com> Reported-by: kernel test robot <lkp@intel.com> Signed-off-by: Herbert Xu <herbert@gondor.apana.org.au>
155 lines
3.4 KiB
C
155 lines
3.4 KiB
C
/* mpi-add.c - MPI functions
|
|
* Copyright (C) 1994, 1996, 1998, 2001, 2002,
|
|
* 2003 Free Software Foundation, Inc.
|
|
*
|
|
* This file is part of Libgcrypt.
|
|
*
|
|
* Note: This code is heavily based on the GNU MP Library.
|
|
* Actually it's the same code with only minor changes in the
|
|
* way the data is stored; this is to support the abstraction
|
|
* of an optional secure memory allocation which may be used
|
|
* to avoid revealing of sensitive data due to paging etc.
|
|
*/
|
|
|
|
#include "mpi-internal.h"
|
|
|
|
/****************
|
|
* Add the unsigned integer V to the mpi-integer U and store the
|
|
* result in W. U and V may be the same.
|
|
*/
|
|
void mpi_add_ui(MPI w, MPI u, unsigned long v)
|
|
{
|
|
mpi_ptr_t wp, up;
|
|
mpi_size_t usize, wsize;
|
|
int usign, wsign;
|
|
|
|
usize = u->nlimbs;
|
|
usign = u->sign;
|
|
wsign = 0;
|
|
|
|
/* If not space for W (and possible carry), increase space. */
|
|
wsize = usize + 1;
|
|
if (w->alloced < wsize)
|
|
mpi_resize(w, wsize);
|
|
|
|
/* These must be after realloc (U may be the same as W). */
|
|
up = u->d;
|
|
wp = w->d;
|
|
|
|
if (!usize) { /* simple */
|
|
wp[0] = v;
|
|
wsize = v ? 1:0;
|
|
} else if (!usign) { /* mpi is not negative */
|
|
mpi_limb_t cy;
|
|
cy = mpihelp_add_1(wp, up, usize, v);
|
|
wp[usize] = cy;
|
|
wsize = usize + cy;
|
|
} else {
|
|
/* The signs are different. Need exact comparison to determine
|
|
* which operand to subtract from which.
|
|
*/
|
|
if (usize == 1 && up[0] < v) {
|
|
wp[0] = v - up[0];
|
|
wsize = 1;
|
|
} else {
|
|
mpihelp_sub_1(wp, up, usize, v);
|
|
/* Size can decrease with at most one limb. */
|
|
wsize = usize - (wp[usize-1] == 0);
|
|
wsign = 1;
|
|
}
|
|
}
|
|
|
|
w->nlimbs = wsize;
|
|
w->sign = wsign;
|
|
}
|
|
|
|
|
|
void mpi_add(MPI w, MPI u, MPI v)
|
|
{
|
|
mpi_ptr_t wp, up, vp;
|
|
mpi_size_t usize, vsize, wsize;
|
|
int usign, vsign, wsign;
|
|
|
|
if (u->nlimbs < v->nlimbs) { /* Swap U and V. */
|
|
usize = v->nlimbs;
|
|
usign = v->sign;
|
|
vsize = u->nlimbs;
|
|
vsign = u->sign;
|
|
wsize = usize + 1;
|
|
RESIZE_IF_NEEDED(w, wsize);
|
|
/* These must be after realloc (u or v may be the same as w). */
|
|
up = v->d;
|
|
vp = u->d;
|
|
} else {
|
|
usize = u->nlimbs;
|
|
usign = u->sign;
|
|
vsize = v->nlimbs;
|
|
vsign = v->sign;
|
|
wsize = usize + 1;
|
|
RESIZE_IF_NEEDED(w, wsize);
|
|
/* These must be after realloc (u or v may be the same as w). */
|
|
up = u->d;
|
|
vp = v->d;
|
|
}
|
|
wp = w->d;
|
|
wsign = 0;
|
|
|
|
if (!vsize) { /* simple */
|
|
MPN_COPY(wp, up, usize);
|
|
wsize = usize;
|
|
wsign = usign;
|
|
} else if (usign != vsign) { /* different sign */
|
|
/* This test is right since USIZE >= VSIZE */
|
|
if (usize != vsize) {
|
|
mpihelp_sub(wp, up, usize, vp, vsize);
|
|
wsize = usize;
|
|
MPN_NORMALIZE(wp, wsize);
|
|
wsign = usign;
|
|
} else if (mpihelp_cmp(up, vp, usize) < 0) {
|
|
mpihelp_sub_n(wp, vp, up, usize);
|
|
wsize = usize;
|
|
MPN_NORMALIZE(wp, wsize);
|
|
if (!usign)
|
|
wsign = 1;
|
|
} else {
|
|
mpihelp_sub_n(wp, up, vp, usize);
|
|
wsize = usize;
|
|
MPN_NORMALIZE(wp, wsize);
|
|
if (usign)
|
|
wsign = 1;
|
|
}
|
|
} else { /* U and V have same sign. Add them. */
|
|
mpi_limb_t cy = mpihelp_add(wp, up, usize, vp, vsize);
|
|
wp[usize] = cy;
|
|
wsize = usize + cy;
|
|
if (usign)
|
|
wsign = 1;
|
|
}
|
|
|
|
w->nlimbs = wsize;
|
|
w->sign = wsign;
|
|
}
|
|
EXPORT_SYMBOL_GPL(mpi_add);
|
|
|
|
void mpi_sub(MPI w, MPI u, MPI v)
|
|
{
|
|
MPI vv = mpi_copy(v);
|
|
vv->sign = !vv->sign;
|
|
mpi_add(w, u, vv);
|
|
mpi_free(vv);
|
|
}
|
|
EXPORT_SYMBOL_GPL(mpi_sub);
|
|
|
|
void mpi_addm(MPI w, MPI u, MPI v, MPI m)
|
|
{
|
|
mpi_add(w, u, v);
|
|
mpi_mod(w, w, m);
|
|
}
|
|
EXPORT_SYMBOL_GPL(mpi_addm);
|
|
|
|
void mpi_subm(MPI w, MPI u, MPI v, MPI m)
|
|
{
|
|
mpi_sub(w, u, v);
|
|
mpi_mod(w, w, m);
|
|
}
|
|
EXPORT_SYMBOL_GPL(mpi_subm);
|