mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-26 02:39:48 +00:00
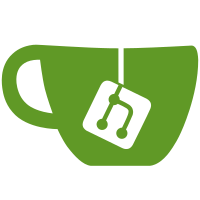
Changes from v1: * exported mpi_sub and mpi_mul, otherwise the build fails when RSA is a module The kernel RSA ASN.1 private key parser already supports only private keys with additional values to be used with the Chinese Remainder Theorem [1], but these values are currently not used. This rudimentary CRT implementation speeds up RSA private key operations for the following Go benchmark up to ~3x. This implementation also tries to minimise the allocation of additional MPIs, so existing MPIs are reused as much as possible (hence the variable names are a bit weird). The benchmark used: ``` package keyring_test import ( "crypto" "crypto/rand" "crypto/rsa" "crypto/x509" "io" "syscall" "testing" "unsafe" ) type KeySerial int32 type Keyring int32 const ( KEY_SPEC_PROCESS_KEYRING Keyring = -2 KEYCTL_PKEY_SIGN = 27 ) var ( keyTypeAsym = []byte("asymmetric\x00") sha256pkcs1 = []byte("enc=pkcs1 hash=sha256\x00") ) func (keyring Keyring) LoadAsym(desc string, payload []byte) (KeySerial, error) { cdesc := []byte(desc + "\x00") serial, _, errno := syscall.Syscall6(syscall.SYS_ADD_KEY, uintptr(unsafe.Pointer(&keyTypeAsym[0])), uintptr(unsafe.Pointer(&cdesc[0])), uintptr(unsafe.Pointer(&payload[0])), uintptr(len(payload)), uintptr(keyring), uintptr(0)) if errno == 0 { return KeySerial(serial), nil } return KeySerial(serial), errno } type pkeyParams struct { key_id KeySerial in_len uint32 out_or_in2_len uint32 __spare [7]uint32 } // the output signature buffer is an input parameter here, because we want to // avoid Go buffer allocation leaking into our benchmarks func (key KeySerial) Sign(info, digest, out []byte) error { var params pkeyParams params.key_id = key params.in_len = uint32(len(digest)) params.out_or_in2_len = uint32(len(out)) _, _, errno := syscall.Syscall6(syscall.SYS_KEYCTL, KEYCTL_PKEY_SIGN, uintptr(unsafe.Pointer(¶ms)), uintptr(unsafe.Pointer(&info[0])), uintptr(unsafe.Pointer(&digest[0])), uintptr(unsafe.Pointer(&out[0])), uintptr(0)) if errno == 0 { return nil } return errno } func BenchmarkSign(b *testing.B) { priv, err := rsa.GenerateKey(rand.Reader, 2048) if err != nil { b.Fatalf("failed to generate private key: %v", err) } pkcs8, err := x509.MarshalPKCS8PrivateKey(priv) if err != nil { b.Fatalf("failed to serialize the private key to PKCS8 blob: %v", err) } serial, err := KEY_SPEC_PROCESS_KEYRING.LoadAsym("test rsa key", pkcs8) if err != nil { b.Fatalf("failed to load the private key into the keyring: %v", err) } b.Logf("loaded test rsa key: %v", serial) digest := make([]byte, 32) _, err = io.ReadFull(rand.Reader, digest) if err != nil { b.Fatalf("failed to generate a random digest: %v", err) } sig := make([]byte, 256) for n := 0; n < b.N; n++ { err = serial.Sign(sha256pkcs1, digest, sig) if err != nil { b.Fatalf("failed to sign the digest: %v", err) } } err = rsa.VerifyPKCS1v15(&priv.PublicKey, crypto.SHA256, digest, sig) if err != nil { b.Fatalf("failed to verify the signature: %v", err) } } ``` [1]: https://en.wikipedia.org/wiki/RSA_(cryptosystem)#Using_the_Chinese_remainder_algorithm Signed-off-by: Ignat Korchagin <ignat@cloudflare.com> Reported-by: kernel test robot <lkp@intel.com> Signed-off-by: Herbert Xu <herbert@gondor.apana.org.au>
92 lines
2.2 KiB
C
92 lines
2.2 KiB
C
/* mpi-mul.c - MPI functions
|
|
* Copyright (C) 1994, 1996, 1998, 2001, 2002,
|
|
* 2003 Free Software Foundation, Inc.
|
|
*
|
|
* This file is part of Libgcrypt.
|
|
*
|
|
* Note: This code is heavily based on the GNU MP Library.
|
|
* Actually it's the same code with only minor changes in the
|
|
* way the data is stored; this is to support the abstraction
|
|
* of an optional secure memory allocation which may be used
|
|
* to avoid revealing of sensitive data due to paging etc.
|
|
*/
|
|
|
|
#include "mpi-internal.h"
|
|
|
|
void mpi_mul(MPI w, MPI u, MPI v)
|
|
{
|
|
mpi_size_t usize, vsize, wsize;
|
|
mpi_ptr_t up, vp, wp;
|
|
mpi_limb_t cy;
|
|
int usign, vsign, sign_product;
|
|
int assign_wp = 0;
|
|
mpi_ptr_t tmp_limb = NULL;
|
|
|
|
if (u->nlimbs < v->nlimbs) {
|
|
/* Swap U and V. */
|
|
usize = v->nlimbs;
|
|
usign = v->sign;
|
|
up = v->d;
|
|
vsize = u->nlimbs;
|
|
vsign = u->sign;
|
|
vp = u->d;
|
|
} else {
|
|
usize = u->nlimbs;
|
|
usign = u->sign;
|
|
up = u->d;
|
|
vsize = v->nlimbs;
|
|
vsign = v->sign;
|
|
vp = v->d;
|
|
}
|
|
sign_product = usign ^ vsign;
|
|
wp = w->d;
|
|
|
|
/* Ensure W has space enough to store the result. */
|
|
wsize = usize + vsize;
|
|
if (w->alloced < wsize) {
|
|
if (wp == up || wp == vp) {
|
|
wp = mpi_alloc_limb_space(wsize);
|
|
assign_wp = 1;
|
|
} else {
|
|
mpi_resize(w, wsize);
|
|
wp = w->d;
|
|
}
|
|
} else { /* Make U and V not overlap with W. */
|
|
if (wp == up) {
|
|
/* W and U are identical. Allocate temporary space for U. */
|
|
up = tmp_limb = mpi_alloc_limb_space(usize);
|
|
/* Is V identical too? Keep it identical with U. */
|
|
if (wp == vp)
|
|
vp = up;
|
|
/* Copy to the temporary space. */
|
|
MPN_COPY(up, wp, usize);
|
|
} else if (wp == vp) {
|
|
/* W and V are identical. Allocate temporary space for V. */
|
|
vp = tmp_limb = mpi_alloc_limb_space(vsize);
|
|
/* Copy to the temporary space. */
|
|
MPN_COPY(vp, wp, vsize);
|
|
}
|
|
}
|
|
|
|
if (!vsize)
|
|
wsize = 0;
|
|
else {
|
|
mpihelp_mul(wp, up, usize, vp, vsize, &cy);
|
|
wsize -= cy ? 0:1;
|
|
}
|
|
|
|
if (assign_wp)
|
|
mpi_assign_limb_space(w, wp, wsize);
|
|
w->nlimbs = wsize;
|
|
w->sign = sign_product;
|
|
if (tmp_limb)
|
|
mpi_free_limb_space(tmp_limb);
|
|
}
|
|
EXPORT_SYMBOL_GPL(mpi_mul);
|
|
|
|
void mpi_mulm(MPI w, MPI u, MPI v, MPI m)
|
|
{
|
|
mpi_mul(w, u, v);
|
|
mpi_tdiv_r(w, w, m);
|
|
}
|
|
EXPORT_SYMBOL_GPL(mpi_mulm);
|