mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
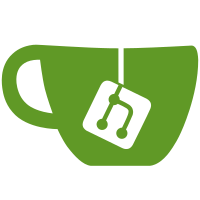
Previously, alloc_ila_locks() and bucket_table_alloc() call spin_lock_init() separately, therefore they have two different lock names and lock class keys. However, after commitb893281715
("ila: Call library function alloc_bucket_locks") they both call helper alloc_bucket_spinlocks() which now only has one lock name and lock class key. This causes a few bogus lockdep warnings as reported by syzbot. Fix this by making alloc_bucket_locks() a macro and pass declaration name as lock name and a static lock class key inside the macro. Fixes:b893281715
("ila: Call library function alloc_bucket_locks") Reported-by: <syzbot+b66a5a554991a8ed027c@syzkaller.appspotmail.com> Cc: Tom Herbert <tom@quantonium.net> Signed-off-by: Cong Wang <xiyou.wangcong@gmail.com> Signed-off-by: David S. Miller <davem@davemloft.net>
54 lines
1.4 KiB
C
54 lines
1.4 KiB
C
#include <linux/export.h>
|
|
#include <linux/kernel.h>
|
|
#include <linux/mm.h>
|
|
#include <linux/slab.h>
|
|
#include <linux/vmalloc.h>
|
|
|
|
/* Allocate an array of spinlocks to be accessed by a hash. Two arguments
|
|
* indicate the number of elements to allocate in the array. max_size
|
|
* gives the maximum number of elements to allocate. cpu_mult gives
|
|
* the number of locks per CPU to allocate. The size is rounded up
|
|
* to a power of 2 to be suitable as a hash table.
|
|
*/
|
|
|
|
int __alloc_bucket_spinlocks(spinlock_t **locks, unsigned int *locks_mask,
|
|
size_t max_size, unsigned int cpu_mult, gfp_t gfp,
|
|
const char *name, struct lock_class_key *key)
|
|
{
|
|
spinlock_t *tlocks = NULL;
|
|
unsigned int i, size;
|
|
#if defined(CONFIG_PROVE_LOCKING)
|
|
unsigned int nr_pcpus = 2;
|
|
#else
|
|
unsigned int nr_pcpus = num_possible_cpus();
|
|
#endif
|
|
|
|
if (cpu_mult) {
|
|
nr_pcpus = min_t(unsigned int, nr_pcpus, 64UL);
|
|
size = min_t(unsigned int, nr_pcpus * cpu_mult, max_size);
|
|
} else {
|
|
size = max_size;
|
|
}
|
|
|
|
if (sizeof(spinlock_t) != 0) {
|
|
tlocks = kvmalloc_array(size, sizeof(spinlock_t), gfp);
|
|
if (!tlocks)
|
|
return -ENOMEM;
|
|
for (i = 0; i < size; i++) {
|
|
spin_lock_init(&tlocks[i]);
|
|
lockdep_init_map(&tlocks[i].dep_map, name, key, 0);
|
|
}
|
|
}
|
|
|
|
*locks = tlocks;
|
|
*locks_mask = size - 1;
|
|
|
|
return 0;
|
|
}
|
|
EXPORT_SYMBOL(__alloc_bucket_spinlocks);
|
|
|
|
void free_bucket_spinlocks(spinlock_t *locks)
|
|
{
|
|
kvfree(locks);
|
|
}
|
|
EXPORT_SYMBOL(free_bucket_spinlocks);
|