mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
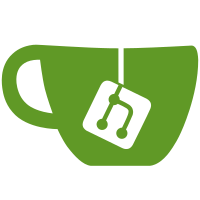
Today, Kubernetes is still operating on cgroups v1, however, it is possible to retrieve the task's classid based on 'current' out of connect(), sendmsg(), recvmsg() and bind-related hooks for orchestrators which attach to the root cgroup v2 hook in a mixed env like in case of Cilium, for example, in order to then correlate certain pod traffic and use it as part of the key for BPF map lookups. Signed-off-by: Daniel Borkmann <daniel@iogearbox.net> Signed-off-by: Alexei Starovoitov <ast@kernel.org> Link: https://lore.kernel.org/bpf/555e1c69db7376c0947007b4951c260e1074efc3.1585323121.git.daniel@iogearbox.net
88 lines
2 KiB
C
88 lines
2 KiB
C
/* SPDX-License-Identifier: GPL-2.0-or-later */
|
|
/*
|
|
* cls_cgroup.h Control Group Classifier
|
|
*
|
|
* Authors: Thomas Graf <tgraf@suug.ch>
|
|
*/
|
|
|
|
#ifndef _NET_CLS_CGROUP_H
|
|
#define _NET_CLS_CGROUP_H
|
|
|
|
#include <linux/cgroup.h>
|
|
#include <linux/hardirq.h>
|
|
#include <linux/rcupdate.h>
|
|
#include <net/sock.h>
|
|
#include <net/inet_sock.h>
|
|
|
|
#ifdef CONFIG_CGROUP_NET_CLASSID
|
|
struct cgroup_cls_state {
|
|
struct cgroup_subsys_state css;
|
|
u32 classid;
|
|
};
|
|
|
|
struct cgroup_cls_state *task_cls_state(struct task_struct *p);
|
|
|
|
static inline u32 task_cls_classid(struct task_struct *p)
|
|
{
|
|
u32 classid;
|
|
|
|
if (in_interrupt())
|
|
return 0;
|
|
|
|
rcu_read_lock();
|
|
classid = container_of(task_css(p, net_cls_cgrp_id),
|
|
struct cgroup_cls_state, css)->classid;
|
|
rcu_read_unlock();
|
|
|
|
return classid;
|
|
}
|
|
|
|
static inline void sock_update_classid(struct sock_cgroup_data *skcd)
|
|
{
|
|
u32 classid;
|
|
|
|
classid = task_cls_classid(current);
|
|
sock_cgroup_set_classid(skcd, classid);
|
|
}
|
|
|
|
static inline u32 __task_get_classid(struct task_struct *task)
|
|
{
|
|
return task_cls_state(task)->classid;
|
|
}
|
|
|
|
static inline u32 task_get_classid(const struct sk_buff *skb)
|
|
{
|
|
u32 classid = __task_get_classid(current);
|
|
|
|
/* Due to the nature of the classifier it is required to ignore all
|
|
* packets originating from softirq context as accessing `current'
|
|
* would lead to false results.
|
|
*
|
|
* This test assumes that all callers of dev_queue_xmit() explicitly
|
|
* disable bh. Knowing this, it is possible to detect softirq based
|
|
* calls by looking at the number of nested bh disable calls because
|
|
* softirqs always disables bh.
|
|
*/
|
|
if (in_serving_softirq()) {
|
|
struct sock *sk = skb_to_full_sk(skb);
|
|
|
|
/* If there is an sock_cgroup_classid we'll use that. */
|
|
if (!sk || !sk_fullsock(sk))
|
|
return 0;
|
|
|
|
classid = sock_cgroup_classid(&sk->sk_cgrp_data);
|
|
}
|
|
|
|
return classid;
|
|
}
|
|
#else /* !CONFIG_CGROUP_NET_CLASSID */
|
|
static inline void sock_update_classid(struct sock_cgroup_data *skcd)
|
|
{
|
|
}
|
|
|
|
static inline u32 task_get_classid(const struct sk_buff *skb)
|
|
{
|
|
return 0;
|
|
}
|
|
#endif /* CONFIG_CGROUP_NET_CLASSID */
|
|
#endif /* _NET_CLS_CGROUP_H */
|