mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 00:48:50 +00:00
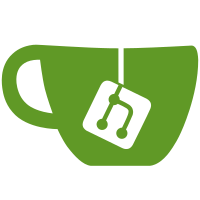
tso_count_descs() is a small function doing simple calculation, and tso_count_descs() is used in fast path, so inline it to reduce the overhead of calls. Signed-off-by: Yunsheng Lin <linyunsheng@huawei.com> Link: https://lore.kernel.org/r/20221212032426.16050-1-linyunsheng@huawei.com Signed-off-by: Jakub Kicinski <kuba@kernel.org>
31 lines
721 B
C
31 lines
721 B
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef _TSO_H
|
|
#define _TSO_H
|
|
|
|
#include <linux/skbuff.h>
|
|
#include <net/ip.h>
|
|
|
|
#define TSO_HEADER_SIZE 256
|
|
|
|
struct tso_t {
|
|
int next_frag_idx;
|
|
int size;
|
|
void *data;
|
|
u16 ip_id;
|
|
u8 tlen; /* transport header len */
|
|
bool ipv6;
|
|
u32 tcp_seq;
|
|
};
|
|
|
|
/* Calculate the worst case buffer count */
|
|
static inline int tso_count_descs(const struct sk_buff *skb)
|
|
{
|
|
return skb_shinfo(skb)->gso_segs * 2 + skb_shinfo(skb)->nr_frags;
|
|
}
|
|
|
|
void tso_build_hdr(const struct sk_buff *skb, char *hdr, struct tso_t *tso,
|
|
int size, bool is_last);
|
|
void tso_build_data(const struct sk_buff *skb, struct tso_t *tso, int size);
|
|
int tso_start(struct sk_buff *skb, struct tso_t *tso);
|
|
|
|
#endif /* _TSO_H */
|