mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-09-12 13:55:32 +00:00
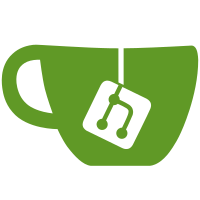
get_current_ioprio() is used to initialize IO priority of various requests. As such it should be returning the effective IO priority of the task (i.e., reflecting the fact that unset IO priority should get set based on task's CPU priority) so that the conversion is concentrated in one place. Reviewed-by: Damien Le Moal <damien.lemoal@opensource.wdc.com> Tested-by: Damien Le Moal <damien.lemoal@opensource.wdc.com> Signed-off-by: Jan Kara <jack@suse.cz> Reviewed-by: Christoph Hellwig <hch@lst.de> Link: https://lore.kernel.org/r/20220623074840.5960-2-jack@suse.cz Signed-off-by: Jens Axboe <axboe@kernel.dk>
85 lines
1.9 KiB
C
85 lines
1.9 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef IOPRIO_H
|
|
#define IOPRIO_H
|
|
|
|
#include <linux/sched.h>
|
|
#include <linux/sched/rt.h>
|
|
#include <linux/iocontext.h>
|
|
|
|
#include <uapi/linux/ioprio.h>
|
|
|
|
/*
|
|
* Default IO priority.
|
|
*/
|
|
#define IOPRIO_DEFAULT IOPRIO_PRIO_VALUE(IOPRIO_CLASS_NONE, 0)
|
|
|
|
/*
|
|
* Check that a priority value has a valid class.
|
|
*/
|
|
static inline bool ioprio_valid(unsigned short ioprio)
|
|
{
|
|
unsigned short class = IOPRIO_PRIO_CLASS(ioprio);
|
|
|
|
return class > IOPRIO_CLASS_NONE && class <= IOPRIO_CLASS_IDLE;
|
|
}
|
|
|
|
/*
|
|
* if process has set io priority explicitly, use that. if not, convert
|
|
* the cpu scheduler nice value to an io priority
|
|
*/
|
|
static inline int task_nice_ioprio(struct task_struct *task)
|
|
{
|
|
return (task_nice(task) + 20) / 5;
|
|
}
|
|
|
|
/*
|
|
* This is for the case where the task hasn't asked for a specific IO class.
|
|
* Check for idle and rt task process, and return appropriate IO class.
|
|
*/
|
|
static inline int task_nice_ioclass(struct task_struct *task)
|
|
{
|
|
if (task->policy == SCHED_IDLE)
|
|
return IOPRIO_CLASS_IDLE;
|
|
else if (task_is_realtime(task))
|
|
return IOPRIO_CLASS_RT;
|
|
else
|
|
return IOPRIO_CLASS_BE;
|
|
}
|
|
|
|
/*
|
|
* If the calling process has set an I/O priority, use that. Otherwise, return
|
|
* the default I/O priority.
|
|
*/
|
|
static inline int get_current_ioprio(void)
|
|
{
|
|
struct io_context *ioc = current->io_context;
|
|
int prio;
|
|
|
|
if (ioc)
|
|
prio = ioc->ioprio;
|
|
else
|
|
prio = IOPRIO_DEFAULT;
|
|
|
|
if (IOPRIO_PRIO_CLASS(prio) == IOPRIO_CLASS_NONE)
|
|
prio = IOPRIO_PRIO_VALUE(task_nice_ioclass(current),
|
|
task_nice_ioprio(current));
|
|
return prio;
|
|
}
|
|
|
|
/*
|
|
* For inheritance, return the highest of the two given priorities
|
|
*/
|
|
extern int ioprio_best(unsigned short aprio, unsigned short bprio);
|
|
|
|
extern int set_task_ioprio(struct task_struct *task, int ioprio);
|
|
|
|
#ifdef CONFIG_BLOCK
|
|
extern int ioprio_check_cap(int ioprio);
|
|
#else
|
|
static inline int ioprio_check_cap(int ioprio)
|
|
{
|
|
return -ENOTBLK;
|
|
}
|
|
#endif /* CONFIG_BLOCK */
|
|
|
|
#endif
|