mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-29 04:09:39 +00:00
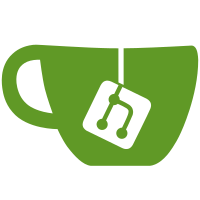
kernel.h is being used as a dump for all kinds of stuff for a long time. Here is the attempt to start cleaning it up by splitting out mathematical helpers. At the same time convert users in header and lib folder to use new header. Though for time being include new header back to kernel.h to avoid twisted indirected includes for existing users. [sfr@canb.auug.org.au: fix powerpc build] Link: https://lkml.kernel.org/r/20201029150809.13059608@canb.auug.org.au Link: https://lkml.kernel.org/r/20201028173212.41768-1-andriy.shevchenko@linux.intel.com Signed-off-by: Andy Shevchenko <andriy.shevchenko@linux.intel.com> Cc: "Paul E. McKenney" <paulmck@kernel.org> Cc: Trond Myklebust <trond.myklebust@hammerspace.com> Cc: Jeff Layton <jlayton@kernel.org> Cc: Rasmus Villemoes <linux@rasmusvillemoes.dk> Signed-off-by: Andrew Morton <akpm@linux-foundation.org> Signed-off-by: Linus Torvalds <torvalds@linux-foundation.org>
92 lines
3.7 KiB
C
92 lines
3.7 KiB
C
/* SPDX-License-Identifier: GPL-2.0+ */
|
|
/*
|
|
* RCU node combining tree definitions. These are used to compute
|
|
* global attributes while avoiding common-case global contention. A key
|
|
* property that these computations rely on is a tournament-style approach
|
|
* where only one of the tasks contending a lower level in the tree need
|
|
* advance to the next higher level. If properly configured, this allows
|
|
* unlimited scalability while maintaining a constant level of contention
|
|
* on the root node.
|
|
*
|
|
* This seemingly RCU-private file must be available to SRCU users
|
|
* because the size of the TREE SRCU srcu_struct structure depends
|
|
* on these definitions.
|
|
*
|
|
* Copyright IBM Corporation, 2017
|
|
*
|
|
* Author: Paul E. McKenney <paulmck@linux.ibm.com>
|
|
*/
|
|
|
|
#ifndef __LINUX_RCU_NODE_TREE_H
|
|
#define __LINUX_RCU_NODE_TREE_H
|
|
|
|
#include <linux/math.h>
|
|
|
|
/*
|
|
* Define shape of hierarchy based on NR_CPUS, CONFIG_RCU_FANOUT, and
|
|
* CONFIG_RCU_FANOUT_LEAF.
|
|
* In theory, it should be possible to add more levels straightforwardly.
|
|
* In practice, this did work well going from three levels to four.
|
|
* Of course, your mileage may vary.
|
|
*/
|
|
|
|
#ifdef CONFIG_RCU_FANOUT
|
|
#define RCU_FANOUT CONFIG_RCU_FANOUT
|
|
#else /* #ifdef CONFIG_RCU_FANOUT */
|
|
# ifdef CONFIG_64BIT
|
|
# define RCU_FANOUT 64
|
|
# else
|
|
# define RCU_FANOUT 32
|
|
# endif
|
|
#endif /* #else #ifdef CONFIG_RCU_FANOUT */
|
|
|
|
#ifdef CONFIG_RCU_FANOUT_LEAF
|
|
#define RCU_FANOUT_LEAF CONFIG_RCU_FANOUT_LEAF
|
|
#else /* #ifdef CONFIG_RCU_FANOUT_LEAF */
|
|
#define RCU_FANOUT_LEAF 16
|
|
#endif /* #else #ifdef CONFIG_RCU_FANOUT_LEAF */
|
|
|
|
#define RCU_FANOUT_1 (RCU_FANOUT_LEAF)
|
|
#define RCU_FANOUT_2 (RCU_FANOUT_1 * RCU_FANOUT)
|
|
#define RCU_FANOUT_3 (RCU_FANOUT_2 * RCU_FANOUT)
|
|
#define RCU_FANOUT_4 (RCU_FANOUT_3 * RCU_FANOUT)
|
|
|
|
#if NR_CPUS <= RCU_FANOUT_1
|
|
# define RCU_NUM_LVLS 1
|
|
# define NUM_RCU_LVL_0 1
|
|
# define NUM_RCU_NODES NUM_RCU_LVL_0
|
|
# define NUM_RCU_LVL_INIT { NUM_RCU_LVL_0 }
|
|
# define RCU_NODE_NAME_INIT { "rcu_node_0" }
|
|
# define RCU_FQS_NAME_INIT { "rcu_node_fqs_0" }
|
|
#elif NR_CPUS <= RCU_FANOUT_2
|
|
# define RCU_NUM_LVLS 2
|
|
# define NUM_RCU_LVL_0 1
|
|
# define NUM_RCU_LVL_1 DIV_ROUND_UP(NR_CPUS, RCU_FANOUT_1)
|
|
# define NUM_RCU_NODES (NUM_RCU_LVL_0 + NUM_RCU_LVL_1)
|
|
# define NUM_RCU_LVL_INIT { NUM_RCU_LVL_0, NUM_RCU_LVL_1 }
|
|
# define RCU_NODE_NAME_INIT { "rcu_node_0", "rcu_node_1" }
|
|
# define RCU_FQS_NAME_INIT { "rcu_node_fqs_0", "rcu_node_fqs_1" }
|
|
#elif NR_CPUS <= RCU_FANOUT_3
|
|
# define RCU_NUM_LVLS 3
|
|
# define NUM_RCU_LVL_0 1
|
|
# define NUM_RCU_LVL_1 DIV_ROUND_UP(NR_CPUS, RCU_FANOUT_2)
|
|
# define NUM_RCU_LVL_2 DIV_ROUND_UP(NR_CPUS, RCU_FANOUT_1)
|
|
# define NUM_RCU_NODES (NUM_RCU_LVL_0 + NUM_RCU_LVL_1 + NUM_RCU_LVL_2)
|
|
# define NUM_RCU_LVL_INIT { NUM_RCU_LVL_0, NUM_RCU_LVL_1, NUM_RCU_LVL_2 }
|
|
# define RCU_NODE_NAME_INIT { "rcu_node_0", "rcu_node_1", "rcu_node_2" }
|
|
# define RCU_FQS_NAME_INIT { "rcu_node_fqs_0", "rcu_node_fqs_1", "rcu_node_fqs_2" }
|
|
#elif NR_CPUS <= RCU_FANOUT_4
|
|
# define RCU_NUM_LVLS 4
|
|
# define NUM_RCU_LVL_0 1
|
|
# define NUM_RCU_LVL_1 DIV_ROUND_UP(NR_CPUS, RCU_FANOUT_3)
|
|
# define NUM_RCU_LVL_2 DIV_ROUND_UP(NR_CPUS, RCU_FANOUT_2)
|
|
# define NUM_RCU_LVL_3 DIV_ROUND_UP(NR_CPUS, RCU_FANOUT_1)
|
|
# define NUM_RCU_NODES (NUM_RCU_LVL_0 + NUM_RCU_LVL_1 + NUM_RCU_LVL_2 + NUM_RCU_LVL_3)
|
|
# define NUM_RCU_LVL_INIT { NUM_RCU_LVL_0, NUM_RCU_LVL_1, NUM_RCU_LVL_2, NUM_RCU_LVL_3 }
|
|
# define RCU_NODE_NAME_INIT { "rcu_node_0", "rcu_node_1", "rcu_node_2", "rcu_node_3" }
|
|
# define RCU_FQS_NAME_INIT { "rcu_node_fqs_0", "rcu_node_fqs_1", "rcu_node_fqs_2", "rcu_node_fqs_3" }
|
|
#else
|
|
# error "CONFIG_RCU_FANOUT insufficient for NR_CPUS"
|
|
#endif /* #if (NR_CPUS) <= RCU_FANOUT_1 */
|
|
|
|
#endif /* __LINUX_RCU_NODE_TREE_H */
|