mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-29 04:09:39 +00:00
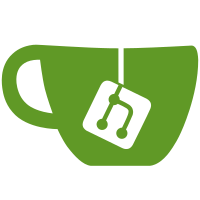
cpumap needs to set, clear, and test the lowest bit in skb pointer in various places. To make these checks less noisy, add pointer friendly bitop macros that also do some typechecking to sanitize the argument. These wrap the non-atomic bitops __set_bit, __clear_bit, and test_bit but for pointer arguments. Pointer's address has to be passed in and it is treated as an unsigned long *, since width and representation of pointer and unsigned long match on targets Linux supports. They are prefixed with double underscore to indicate lack of atomicity. Signed-off-by: Kumar Kartikeya Dwivedi <memxor@gmail.com> Signed-off-by: Alexei Starovoitov <ast@kernel.org> Reviewed-by: Toke Høiland-Jørgensen <toke@redhat.com> Link: https://lore.kernel.org/bpf/20210702111825.491065-3-memxor@gmail.com
34 lines
782 B
C
34 lines
782 B
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef TYPECHECK_H_INCLUDED
|
|
#define TYPECHECK_H_INCLUDED
|
|
|
|
/*
|
|
* Check at compile time that something is of a particular type.
|
|
* Always evaluates to 1 so you may use it easily in comparisons.
|
|
*/
|
|
#define typecheck(type,x) \
|
|
({ type __dummy; \
|
|
typeof(x) __dummy2; \
|
|
(void)(&__dummy == &__dummy2); \
|
|
1; \
|
|
})
|
|
|
|
/*
|
|
* Check at compile time that 'function' is a certain type, or is a pointer
|
|
* to that type (needs to use typedef for the function type.)
|
|
*/
|
|
#define typecheck_fn(type,function) \
|
|
({ typeof(type) __tmp = function; \
|
|
(void)__tmp; \
|
|
})
|
|
|
|
/*
|
|
* Check at compile time that something is a pointer type.
|
|
*/
|
|
#define typecheck_pointer(x) \
|
|
({ typeof(x) __dummy; \
|
|
(void)sizeof(*__dummy); \
|
|
1; \
|
|
})
|
|
|
|
#endif /* TYPECHECK_H_INCLUDED */
|