mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-11-01 17:08:10 +00:00
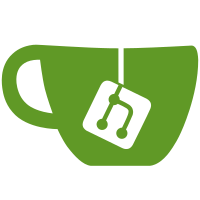
Store flags per struct pollfd *entries object in a bitmap of int size. Implement fdarray_flag__nonfilterable flag to skip object from counting by fdarray__filter(). Fixed fdarray test issue reported by kernel test robot. Reported-by: kernel test robot <rong.a.chen@intel.com> Signed-off-by: Alexey Budankov <alexey.budankov@linux.intel.com> Acked-by: Jiri Olsa <jolsa@redhat.com> Acked-by: Namhyung Kim <namhyung@kernel.org> Cc: Alexander Shishkin <alexander.shishkin@linux.intel.com> Cc: Andi Kleen <ak@linux.intel.com> Cc: Peter Zijlstra <peterz@infradead.org> Link: http://lore.kernel.org/lkml/6b7d43ff-0801-d5dd-4e90-fcd86b17c1c8@linux.intel.com Signed-off-by: Arnaldo Carvalho de Melo <acme@redhat.com>
57 lines
1.5 KiB
C
57 lines
1.5 KiB
C
/* SPDX-License-Identifier: GPL-2.0 */
|
|
#ifndef __API_FD_ARRAY__
|
|
#define __API_FD_ARRAY__
|
|
|
|
#include <stdio.h>
|
|
|
|
struct pollfd;
|
|
|
|
/**
|
|
* struct fdarray: Array of file descriptors
|
|
*
|
|
* @priv: Per array entry priv area, users should access just its contents,
|
|
* not set it to anything, as it is kept in synch with @entries, being
|
|
* realloc'ed, * for instance, in fdarray__{grow,filter}.
|
|
*
|
|
* I.e. using 'fda->priv[N].idx = * value' where N < fda->nr is ok,
|
|
* but doing 'fda->priv = malloc(M)' is not allowed.
|
|
*/
|
|
struct fdarray {
|
|
int nr;
|
|
int nr_alloc;
|
|
int nr_autogrow;
|
|
struct pollfd *entries;
|
|
struct priv {
|
|
union {
|
|
int idx;
|
|
void *ptr;
|
|
};
|
|
unsigned int flags;
|
|
} *priv;
|
|
};
|
|
|
|
enum fdarray_flags {
|
|
fdarray_flag__default = 0x00000000,
|
|
fdarray_flag__nonfilterable = 0x00000001
|
|
};
|
|
|
|
void fdarray__init(struct fdarray *fda, int nr_autogrow);
|
|
void fdarray__exit(struct fdarray *fda);
|
|
|
|
struct fdarray *fdarray__new(int nr_alloc, int nr_autogrow);
|
|
void fdarray__delete(struct fdarray *fda);
|
|
|
|
int fdarray__add(struct fdarray *fda, int fd, short revents, enum fdarray_flags flags);
|
|
int fdarray__poll(struct fdarray *fda, int timeout);
|
|
int fdarray__filter(struct fdarray *fda, short revents,
|
|
void (*entry_destructor)(struct fdarray *fda, int fd, void *arg),
|
|
void *arg);
|
|
int fdarray__grow(struct fdarray *fda, int extra);
|
|
int fdarray__fprintf(struct fdarray *fda, FILE *fp);
|
|
|
|
static inline int fdarray__available_entries(struct fdarray *fda)
|
|
{
|
|
return fda->nr_alloc - fda->nr;
|
|
}
|
|
|
|
#endif /* __API_FD_ARRAY__ */
|