mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-10-14 04:26:45 +00:00
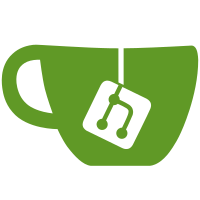
Implement the service call for configuring a shared structure between a VCPU and the hypervisor in which the hypervisor can write the time stolen from the VCPU's execution time by other tasks on the host. User space allocates memory which is placed at an IPA also chosen by user space. The hypervisor then updates the shared structure using kvm_put_guest() to ensure single copy atomicity of the 64-bit value reporting the stolen time in nanoseconds. Whenever stolen time is enabled by the guest, the stolen time counter is reset. The stolen time itself is retrieved from the sched_info structure maintained by the Linux scheduler code. We enable SCHEDSTATS when selecting KVM Kconfig to ensure this value is meaningful. Signed-off-by: Steven Price <steven.price@arm.com> Signed-off-by: Marc Zyngier <maz@kernel.org>
71 lines
1.5 KiB
C
71 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
// Copyright (C) 2019 Arm Ltd.
|
|
|
|
#include <linux/arm-smccc.h>
|
|
#include <linux/kvm_host.h>
|
|
|
|
#include <asm/kvm_emulate.h>
|
|
|
|
#include <kvm/arm_hypercalls.h>
|
|
#include <kvm/arm_psci.h>
|
|
|
|
int kvm_hvc_call_handler(struct kvm_vcpu *vcpu)
|
|
{
|
|
u32 func_id = smccc_get_function(vcpu);
|
|
long val = SMCCC_RET_NOT_SUPPORTED;
|
|
u32 feature;
|
|
gpa_t gpa;
|
|
|
|
switch (func_id) {
|
|
case ARM_SMCCC_VERSION_FUNC_ID:
|
|
val = ARM_SMCCC_VERSION_1_1;
|
|
break;
|
|
case ARM_SMCCC_ARCH_FEATURES_FUNC_ID:
|
|
feature = smccc_get_arg1(vcpu);
|
|
switch (feature) {
|
|
case ARM_SMCCC_ARCH_WORKAROUND_1:
|
|
switch (kvm_arm_harden_branch_predictor()) {
|
|
case KVM_BP_HARDEN_UNKNOWN:
|
|
break;
|
|
case KVM_BP_HARDEN_WA_NEEDED:
|
|
val = SMCCC_RET_SUCCESS;
|
|
break;
|
|
case KVM_BP_HARDEN_NOT_REQUIRED:
|
|
val = SMCCC_RET_NOT_REQUIRED;
|
|
break;
|
|
}
|
|
break;
|
|
case ARM_SMCCC_ARCH_WORKAROUND_2:
|
|
switch (kvm_arm_have_ssbd()) {
|
|
case KVM_SSBD_FORCE_DISABLE:
|
|
case KVM_SSBD_UNKNOWN:
|
|
break;
|
|
case KVM_SSBD_KERNEL:
|
|
val = SMCCC_RET_SUCCESS;
|
|
break;
|
|
case KVM_SSBD_FORCE_ENABLE:
|
|
case KVM_SSBD_MITIGATED:
|
|
val = SMCCC_RET_NOT_REQUIRED;
|
|
break;
|
|
}
|
|
break;
|
|
case ARM_SMCCC_HV_PV_TIME_FEATURES:
|
|
val = SMCCC_RET_SUCCESS;
|
|
break;
|
|
}
|
|
break;
|
|
case ARM_SMCCC_HV_PV_TIME_FEATURES:
|
|
val = kvm_hypercall_pv_features(vcpu);
|
|
break;
|
|
case ARM_SMCCC_HV_PV_TIME_ST:
|
|
gpa = kvm_init_stolen_time(vcpu);
|
|
if (gpa != GPA_INVALID)
|
|
val = gpa;
|
|
break;
|
|
default:
|
|
return kvm_psci_call(vcpu);
|
|
}
|
|
|
|
smccc_set_retval(vcpu, val, 0, 0, 0);
|
|
return 1;
|
|
}
|