mirror of
https://git.kernel.org/pub/scm/linux/kernel/git/stable/linux.git
synced 2024-08-22 17:01:14 +00:00
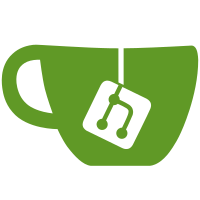
Now that the scheduler can deal with migrate disable properly, there is no real compelling reason to make it only available for RT. There are quite some code pathes which needlessly disable preemption in order to prevent migration and some constructs like kmap_atomic() enforce it implicitly. Making it available independent of RT allows to provide a preemptible variant of kmap_atomic() and makes the code more consistent in general. Signed-off-by: Thomas Gleixner <tglx@linutronix.de> Grudgingly-Acked-by: Peter Zijlstra (Intel) <peterz@infradead.org> Link: https://lore.kernel.org/r/20201118204007.269943012@linutronix.de
72 lines
1.5 KiB
C
72 lines
1.5 KiB
C
// SPDX-License-Identifier: GPL-2.0
|
|
/*
|
|
* lib/smp_processor_id.c
|
|
*
|
|
* DEBUG_PREEMPT variant of smp_processor_id().
|
|
*/
|
|
#include <linux/export.h>
|
|
#include <linux/kprobes.h>
|
|
#include <linux/sched.h>
|
|
|
|
noinstr static
|
|
unsigned int check_preemption_disabled(const char *what1, const char *what2)
|
|
{
|
|
int this_cpu = raw_smp_processor_id();
|
|
|
|
if (likely(preempt_count()))
|
|
goto out;
|
|
|
|
if (irqs_disabled())
|
|
goto out;
|
|
|
|
/*
|
|
* Kernel threads bound to a single CPU can safely use
|
|
* smp_processor_id():
|
|
*/
|
|
if (current->nr_cpus_allowed == 1)
|
|
goto out;
|
|
|
|
#ifdef CONFIG_SMP
|
|
if (current->migration_disabled)
|
|
goto out;
|
|
#endif
|
|
|
|
/*
|
|
* It is valid to assume CPU-locality during early bootup:
|
|
*/
|
|
if (system_state < SYSTEM_SCHEDULING)
|
|
goto out;
|
|
|
|
/*
|
|
* Avoid recursion:
|
|
*/
|
|
preempt_disable_notrace();
|
|
|
|
instrumentation_begin();
|
|
if (!printk_ratelimit())
|
|
goto out_enable;
|
|
|
|
printk(KERN_ERR "BUG: using %s%s() in preemptible [%08x] code: %s/%d\n",
|
|
what1, what2, preempt_count() - 1, current->comm, current->pid);
|
|
|
|
printk("caller is %pS\n", __builtin_return_address(0));
|
|
dump_stack();
|
|
instrumentation_end();
|
|
|
|
out_enable:
|
|
preempt_enable_no_resched_notrace();
|
|
out:
|
|
return this_cpu;
|
|
}
|
|
|
|
noinstr unsigned int debug_smp_processor_id(void)
|
|
{
|
|
return check_preemption_disabled("smp_processor_id", "");
|
|
}
|
|
EXPORT_SYMBOL(debug_smp_processor_id);
|
|
|
|
noinstr void __this_cpu_preempt_check(const char *op)
|
|
{
|
|
check_preemption_disabled("__this_cpu_", op);
|
|
}
|
|
EXPORT_SYMBOL(__this_cpu_preempt_check);
|