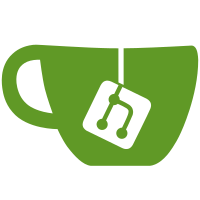
I came in like a wrecking ball.... I think the grpc server and rpc configuration for the server should be handled outside of this package. There are many ways to configure it and we need more flexability on start and shutdown for grpc services. Signal handling should be in the caller.
40 lines
802 B
Go
40 lines
802 B
Go
package element
|
|
|
|
import (
|
|
"encoding/json"
|
|
"time"
|
|
)
|
|
|
|
// PeerAgent is the peer information for an agent in the cluster including name and GRPC address
|
|
type PeerAgent struct {
|
|
Name string
|
|
Addr string
|
|
Updated time.Time
|
|
}
|
|
|
|
// Peers returns all known peers in the cluster
|
|
func (a *Agent) Peers() ([]*PeerAgent, error) {
|
|
self := a.members.LocalNode()
|
|
var (
|
|
peerAgents map[string]*PeerAgent
|
|
peers []*PeerAgent
|
|
)
|
|
if err := json.Unmarshal(self.Meta, &peerAgents); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
for _, p := range peerAgents {
|
|
peers = append(peers, p)
|
|
}
|
|
|
|
return peers, nil
|
|
}
|
|
|
|
// LocalNode returns local node peer info
|
|
func (a *Agent) LocalNode() (*PeerAgent, error) {
|
|
return &PeerAgent{
|
|
Name: a.config.NodeName,
|
|
Addr: a.config.Address,
|
|
Updated: time.Now(),
|
|
}, nil
|
|
}
|