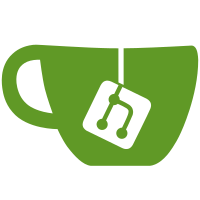
With the rename of fetch to fetch-object, we now introduce the `fetch` command. It will fetch all of the resources required for an image into the content store. We'll still need to follow this up with metadata registration but this is a good start. Signed-off-by: Stephen J Day <stephen.day@docker.com>
25 lines
568 B
Go
25 lines
568 B
Go
package progress
|
|
|
|
import (
|
|
"fmt"
|
|
"time"
|
|
|
|
units "github.com/docker/go-units"
|
|
)
|
|
|
|
// Bytes converts a regular int64 to human readable type.
|
|
type Bytes int64
|
|
|
|
func (b Bytes) String() string {
|
|
return units.CustomSize("%02.1f %s", float64(b), 1024.0, []string{"B", "KiB", "MiB", "GiB", "TiB", "PiB", "EiB", "ZiB", "YiB"})
|
|
}
|
|
|
|
type BytesPerSecond int64
|
|
|
|
func NewBytesPerSecond(n int64, duration time.Duration) BytesPerSecond {
|
|
return BytesPerSecond(float64(n) / duration.Seconds())
|
|
}
|
|
|
|
func (bps BytesPerSecond) String() string {
|
|
return fmt.Sprintf("%v/s", Bytes(bps))
|
|
}
|