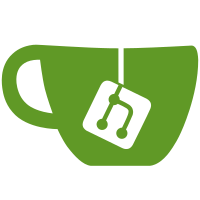
After implementing pull, a few changes are required to the content store interface to make sure that the implementation works smoothly. Specifically, we work to make sure the predeclaration path for digests works the same between remote and local writers. Before, we were hesitent to require the the size and digest up front, but it became clear that having this provided significant benefit. There are also several cleanups related to naming. We now call the expected digest `Expected` consistently across the board and `Total` is used to mark the expected size. This whole effort comes together to provide a very smooth status reporting workflow for image pull and push. This will be more obvious when the bulk of pull code lands. There are a few other changes to make `content.WriteBlob` more broadly useful. In accordance with addition for predeclaring expected size when getting a `Writer`, `WriteBlob` now supports this fully. It will also resume downloads if provided an `io.Seeker` or `io.ReaderAt`. Coupled with the `httpReadSeeker` from `docker/distribution`, we should only be a lines of code away from resumable downloads. Signed-off-by: Stephen J Day <stephen.day@docker.com>
41 lines
1.5 KiB
Go
41 lines
1.5 KiB
Go
package remotes
|
|
|
|
import (
|
|
"context"
|
|
"io"
|
|
)
|
|
|
|
type Fetcher interface {
|
|
// Fetch the resource identified by id. The id is opaque to the remote, but
|
|
// may typically be a tag or a digest.
|
|
//
|
|
// Hints are provided to give instruction on how the resource may be
|
|
// fetched. They may provide information about the expected type or size.
|
|
// They may be protocol specific or help a protocol to identify the most
|
|
// efficient fetch methodology.
|
|
//
|
|
// Hints are the format of `<type>:<content>` where `<type>` is the type
|
|
// of the hint and `<content>` can be pretty much anything. For example, a
|
|
// media type hint would be the following:
|
|
//
|
|
// mediatype:application/vnd.docker.distribution.manifest.v2+json
|
|
//
|
|
// The following hint names are must be honored across all remote
|
|
// implementations:
|
|
//
|
|
// size: specify the expected size in bytes
|
|
// mediatype: specify the expected mediatype
|
|
//
|
|
// The caller should never expect the hints to be honored and should
|
|
// validate that returned content is as expected. They are only provided to
|
|
// help the remote retrieve the content.
|
|
Fetch(ctx context.Context, id string, hints ...string) (io.ReadCloser, error)
|
|
}
|
|
|
|
// FetcherFunc allows package users to implement a Fetcher with just a
|
|
// function.
|
|
type FetcherFunc func(context.Context, string, ...string) (io.ReadCloser, error)
|
|
|
|
func (fn FetcherFunc) Fetch(ctx context.Context, object string, hints ...string) (io.ReadCloser, error) {
|
|
return fn(ctx, object, hints...)
|
|
}
|