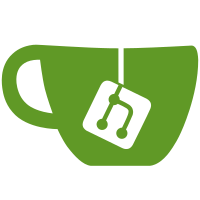
The oom control file may not exist if the container is short lived and exits before we can open it. Signed-off-by: Michael Crosby <crosbymichael@gmail.com>
74 lines
1.6 KiB
Go
74 lines
1.6 KiB
Go
package supervisor
|
|
|
|
import (
|
|
"sync"
|
|
"time"
|
|
|
|
"github.com/Sirupsen/logrus"
|
|
"github.com/docker/containerd/runtime"
|
|
)
|
|
|
|
type Worker interface {
|
|
Start()
|
|
}
|
|
|
|
type startTask struct {
|
|
Container runtime.Container
|
|
Checkpoint string
|
|
Stdin string
|
|
Stdout string
|
|
Stderr string
|
|
Err chan error
|
|
StartResponse chan StartResponse
|
|
}
|
|
|
|
func NewWorker(s *Supervisor, wg *sync.WaitGroup) Worker {
|
|
return &worker{
|
|
s: s,
|
|
wg: wg,
|
|
}
|
|
}
|
|
|
|
type worker struct {
|
|
wg *sync.WaitGroup
|
|
s *Supervisor
|
|
}
|
|
|
|
func (w *worker) Start() {
|
|
defer w.wg.Done()
|
|
for t := range w.s.startTasks {
|
|
started := time.Now()
|
|
process, err := t.Container.Start(t.Checkpoint, runtime.NewStdio(t.Stdin, t.Stdout, t.Stderr))
|
|
if err != nil {
|
|
logrus.WithFields(logrus.Fields{
|
|
"error": err,
|
|
"id": t.Container.ID(),
|
|
}).Error("containerd: start container")
|
|
t.Err <- err
|
|
evt := &DeleteTask{
|
|
ID: t.Container.ID(),
|
|
NoEvent: true,
|
|
}
|
|
w.s.SendTask(evt)
|
|
continue
|
|
}
|
|
if err := w.s.monitor.MonitorOOM(t.Container); err != nil && err != runtime.ErrContainerExited {
|
|
if process.State() != runtime.Stopped {
|
|
logrus.WithField("error", err).Error("containerd: notify OOM events")
|
|
}
|
|
}
|
|
if err := w.s.monitorProcess(process); err != nil {
|
|
logrus.WithField("error", err).Error("containerd: add process to monitor")
|
|
}
|
|
ContainerStartTimer.UpdateSince(started)
|
|
t.Err <- nil
|
|
t.StartResponse <- StartResponse{
|
|
Container: t.Container,
|
|
}
|
|
w.s.notifySubscribers(Event{
|
|
Timestamp: time.Now(),
|
|
ID: t.Container.ID(),
|
|
Type: "start-container",
|
|
})
|
|
}
|
|
}
|