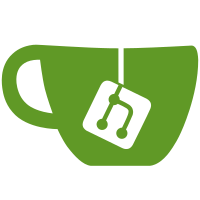
This changeset adds the simple apply command. It consumes a tar layer and applies that layer to the specified directory. For the most part, it is a direct call into Docker's `pkg/archive.ApplyLayer`. The following demonstrates unpacking the wordpress rootfs into a local directory `wordpress`: ``` $ ./dist fetch docker.io/library/wordpress 4.5 mediatype:application/vnd.docker.distribution.manifest.v2+json | \ jq -r '.layers[] | "sudo ./dist apply ./wordpress < $(./dist path -n "+.digest+")"' | xargs -I{} -n1 sh -c "{}" ``` Note that you should have fetched the layers into the local content store before running the above. Alternatively, you can just read the manifest from the content store, rather than fetching it. We use fetch above to avoid having to lookup the manifest digest for our demo. This tool has a long way to go. We still need to incorporate snapshotting, as well as the ability to calculate the `ChainID` under subsequent unpacking. Once we have some tools to play around with snapshotting, we'll be able to incorporate our `rootfs.ApplyLayer` algorithm that will get us a lot closer to a production worthy system. Signed-off-by: Stephen J Day <stephen.day@docker.com>
50 lines
855 B
Go
50 lines
855 B
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
|
|
"github.com/Sirupsen/logrus"
|
|
"github.com/docker/containerd"
|
|
"github.com/urfave/cli"
|
|
)
|
|
|
|
func main() {
|
|
app := cli.NewApp()
|
|
app.Name = "dist"
|
|
app.Version = containerd.Version
|
|
app.Usage = `
|
|
___ __
|
|
____/ (_)____/ /_
|
|
/ __ / / ___/ __/
|
|
/ /_/ / (__ ) /_
|
|
\__,_/_/____/\__/
|
|
|
|
distribution tool
|
|
`
|
|
app.Flags = []cli.Flag{
|
|
cli.BoolFlag{
|
|
Name: "debug",
|
|
Usage: "enable debug output in logs",
|
|
},
|
|
}
|
|
app.Commands = []cli.Command{
|
|
fetchCommand,
|
|
ingestCommand,
|
|
activeCommand,
|
|
pathCommand,
|
|
deleteCommand,
|
|
listCommand,
|
|
applyCommand,
|
|
}
|
|
app.Before = func(context *cli.Context) error {
|
|
if context.GlobalBool("debug") {
|
|
logrus.SetLevel(logrus.DebugLevel)
|
|
}
|
|
return nil
|
|
}
|
|
if err := app.Run(os.Args); err != nil {
|
|
fmt.Fprintf(os.Stderr, "dist: %s\n", err)
|
|
os.Exit(1)
|
|
}
|
|
}
|