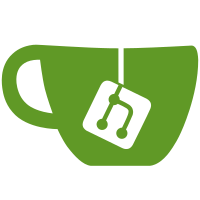
This currently depends on a runc PR: https://github.com/opencontainers/runc/pull/703 We need this pr because we have to SIGKILL runc and the container root dir will still be left around. As for the containerd changes this adds a flag to containerd so that you can configure the timeout without any more code changes. It also adds better handling in the error cases and will kill the containerd-shim and runc ( as well as the user process if it exists ) if the timeout is hit. Signed-off-by: Michael Crosby <crosbymichael@gmail.com>
54 lines
1.1 KiB
Go
54 lines
1.1 KiB
Go
package supervisor
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/docker/containerd/runtime"
|
|
)
|
|
|
|
type StartTask struct {
|
|
baseTask
|
|
platformStartTask
|
|
ID string
|
|
BundlePath string
|
|
Stdout string
|
|
Stderr string
|
|
Stdin string
|
|
StartResponse chan StartResponse
|
|
Labels []string
|
|
NoPivotRoot bool
|
|
}
|
|
|
|
func (s *Supervisor) start(t *StartTask) error {
|
|
start := time.Now()
|
|
container, err := runtime.New(runtime.ContainerOpts{
|
|
Root: s.stateDir,
|
|
ID: t.ID,
|
|
Bundle: t.BundlePath,
|
|
Runtime: s.runtime,
|
|
RuntimeArgs: s.runtimeArgs,
|
|
Labels: t.Labels,
|
|
NoPivotRoot: t.NoPivotRoot,
|
|
Timeout: s.timeout,
|
|
})
|
|
if err != nil {
|
|
return err
|
|
}
|
|
s.containers[t.ID] = &containerInfo{
|
|
container: container,
|
|
}
|
|
ContainersCounter.Inc(1)
|
|
task := &startTask{
|
|
Err: t.ErrorCh(),
|
|
Container: container,
|
|
StartResponse: t.StartResponse,
|
|
Stdin: t.Stdin,
|
|
Stdout: t.Stdout,
|
|
Stderr: t.Stderr,
|
|
}
|
|
task.setTaskCheckpoint(t)
|
|
|
|
s.startTasks <- task
|
|
ContainerCreateTimer.UpdateSince(start)
|
|
return errDeferredResponse
|
|
}
|