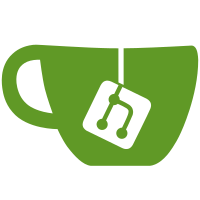
Signed-off-by: John Howard <jhoward@microsoft.com> Move process sorter to new file Signed-off-by: Michael Crosby <crosbymichael@gmail.com> Sort containers by id This will not be the most accurate sorting but atleast the list will be consistent inbetween calls. Signed-off-by: Michael Crosby <crosbymichael@gmail.com> Allow runtime to be configurable via daemon start This allows people to pass an alternate name or location to the runtime binary to start containers. Signed-off-by: Michael Crosby <crosbymichael@gmail.com> Fix state output for containers Return the proper state/status for a container by checking if the pid is still alive. Also fix the cleanup handling in the shim to make sure containers are not left behind. Signed-off-by: Michael Crosby <crosbymichael@gmail.com> Properly wait for container start Signed-off-by: Michael Crosby <crosbymichael@gmail.com>
44 lines
899 B
Go
44 lines
899 B
Go
package supervisor
|
|
|
|
import (
|
|
"time"
|
|
|
|
"github.com/docker/containerd/runtime"
|
|
)
|
|
|
|
type StartTask struct {
|
|
baseTask
|
|
platformStartTask
|
|
ID string
|
|
BundlePath string
|
|
Stdout string
|
|
Stderr string
|
|
Stdin string
|
|
StartResponse chan StartResponse
|
|
Labels []string
|
|
}
|
|
|
|
func (s *Supervisor) start(t *StartTask) error {
|
|
start := time.Now()
|
|
container, err := runtime.New(s.stateDir, t.ID, t.BundlePath, s.runtime, t.Labels)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
s.containers[t.ID] = &containerInfo{
|
|
container: container,
|
|
}
|
|
ContainersCounter.Inc(1)
|
|
task := &startTask{
|
|
Err: t.ErrorCh(),
|
|
Container: container,
|
|
StartResponse: t.StartResponse,
|
|
Stdin: t.Stdin,
|
|
Stdout: t.Stdout,
|
|
Stderr: t.Stderr,
|
|
}
|
|
task.setTaskCheckpoint(t)
|
|
|
|
s.startTasks <- task
|
|
ContainerCreateTimer.UpdateSince(start)
|
|
return errDeferedResponse
|
|
}
|