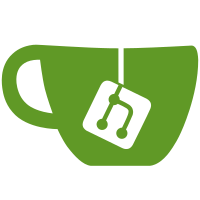
Initial vendor list validated with empty $GOPATH and only master checked out; followed by `make` and verified that all binaries build properly. Updates require github.com/LK4D4/vndr tool. Signed-off-by: Phil Estes <estesp@linux.vnet.ibm.com>
2794 lines
63 KiB
Go
2794 lines
63 KiB
Go
// Code generated by protoc-gen-gogo.
|
|
// source: protocol.proto
|
|
// DO NOT EDIT!
|
|
|
|
/*
|
|
Package pb is a generated protocol buffer package.
|
|
|
|
It is generated from these files:
|
|
protocol.proto
|
|
|
|
It has these top-level messages:
|
|
PubMsg
|
|
PubAck
|
|
MsgProto
|
|
Ack
|
|
ConnectRequest
|
|
ConnectResponse
|
|
SubscriptionRequest
|
|
SubscriptionResponse
|
|
UnsubscribeRequest
|
|
CloseRequest
|
|
CloseResponse
|
|
*/
|
|
package pb
|
|
|
|
import proto "github.com/gogo/protobuf/proto"
|
|
import fmt "fmt"
|
|
import math "math"
|
|
import _ "github.com/gogo/protobuf/gogoproto"
|
|
|
|
import io "io"
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ = proto.Marshal
|
|
var _ = fmt.Errorf
|
|
var _ = math.Inf
|
|
|
|
// Enum for start position type.
|
|
type StartPosition int32
|
|
|
|
const (
|
|
StartPosition_NewOnly StartPosition = 0
|
|
StartPosition_LastReceived StartPosition = 1
|
|
StartPosition_TimeDeltaStart StartPosition = 2
|
|
StartPosition_SequenceStart StartPosition = 3
|
|
StartPosition_First StartPosition = 4
|
|
)
|
|
|
|
var StartPosition_name = map[int32]string{
|
|
0: "NewOnly",
|
|
1: "LastReceived",
|
|
2: "TimeDeltaStart",
|
|
3: "SequenceStart",
|
|
4: "First",
|
|
}
|
|
var StartPosition_value = map[string]int32{
|
|
"NewOnly": 0,
|
|
"LastReceived": 1,
|
|
"TimeDeltaStart": 2,
|
|
"SequenceStart": 3,
|
|
"First": 4,
|
|
}
|
|
|
|
func (x StartPosition) String() string {
|
|
return proto.EnumName(StartPosition_name, int32(x))
|
|
}
|
|
|
|
// How messages are delivered to the STAN cluster
|
|
type PubMsg struct {
|
|
ClientID string `protobuf:"bytes,1,opt,name=clientID,proto3" json:"clientID,omitempty"`
|
|
Guid string `protobuf:"bytes,2,opt,name=guid,proto3" json:"guid,omitempty"`
|
|
Subject string `protobuf:"bytes,3,opt,name=subject,proto3" json:"subject,omitempty"`
|
|
Reply string `protobuf:"bytes,4,opt,name=reply,proto3" json:"reply,omitempty"`
|
|
Data []byte `protobuf:"bytes,5,opt,name=data,proto3" json:"data,omitempty"`
|
|
Sha256 []byte `protobuf:"bytes,10,opt,name=sha256,proto3" json:"sha256,omitempty"`
|
|
}
|
|
|
|
func (m *PubMsg) Reset() { *m = PubMsg{} }
|
|
func (m *PubMsg) String() string { return proto.CompactTextString(m) }
|
|
func (*PubMsg) ProtoMessage() {}
|
|
|
|
// Used to ACK to publishers
|
|
type PubAck struct {
|
|
Guid string `protobuf:"bytes,1,opt,name=guid,proto3" json:"guid,omitempty"`
|
|
Error string `protobuf:"bytes,2,opt,name=error,proto3" json:"error,omitempty"`
|
|
}
|
|
|
|
func (m *PubAck) Reset() { *m = PubAck{} }
|
|
func (m *PubAck) String() string { return proto.CompactTextString(m) }
|
|
func (*PubAck) ProtoMessage() {}
|
|
|
|
// Msg struct. Sequence is assigned for global ordering by
|
|
// the cluster after the publisher has been acknowledged.
|
|
type MsgProto struct {
|
|
Sequence uint64 `protobuf:"varint,1,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
Subject string `protobuf:"bytes,2,opt,name=subject,proto3" json:"subject,omitempty"`
|
|
Reply string `protobuf:"bytes,3,opt,name=reply,proto3" json:"reply,omitempty"`
|
|
Data []byte `protobuf:"bytes,4,opt,name=data,proto3" json:"data,omitempty"`
|
|
Timestamp int64 `protobuf:"varint,5,opt,name=timestamp,proto3" json:"timestamp,omitempty"`
|
|
Redelivered bool `protobuf:"varint,6,opt,name=redelivered,proto3" json:"redelivered,omitempty"`
|
|
CRC32 uint32 `protobuf:"varint,10,opt,name=CRC32,proto3" json:"CRC32,omitempty"`
|
|
}
|
|
|
|
func (m *MsgProto) Reset() { *m = MsgProto{} }
|
|
func (m *MsgProto) String() string { return proto.CompactTextString(m) }
|
|
func (*MsgProto) ProtoMessage() {}
|
|
|
|
// Ack will deliver an ack for a delivered msg.
|
|
type Ack struct {
|
|
Subject string `protobuf:"bytes,1,opt,name=subject,proto3" json:"subject,omitempty"`
|
|
Sequence uint64 `protobuf:"varint,2,opt,name=sequence,proto3" json:"sequence,omitempty"`
|
|
}
|
|
|
|
func (m *Ack) Reset() { *m = Ack{} }
|
|
func (m *Ack) String() string { return proto.CompactTextString(m) }
|
|
func (*Ack) ProtoMessage() {}
|
|
|
|
// Connection Request
|
|
type ConnectRequest struct {
|
|
ClientID string `protobuf:"bytes,1,opt,name=clientID,proto3" json:"clientID,omitempty"`
|
|
HeartbeatInbox string `protobuf:"bytes,2,opt,name=heartbeatInbox,proto3" json:"heartbeatInbox,omitempty"`
|
|
}
|
|
|
|
func (m *ConnectRequest) Reset() { *m = ConnectRequest{} }
|
|
func (m *ConnectRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*ConnectRequest) ProtoMessage() {}
|
|
|
|
// Response to a client connect
|
|
type ConnectResponse struct {
|
|
PubPrefix string `protobuf:"bytes,1,opt,name=pubPrefix,proto3" json:"pubPrefix,omitempty"`
|
|
SubRequests string `protobuf:"bytes,2,opt,name=subRequests,proto3" json:"subRequests,omitempty"`
|
|
UnsubRequests string `protobuf:"bytes,3,opt,name=unsubRequests,proto3" json:"unsubRequests,omitempty"`
|
|
CloseRequests string `protobuf:"bytes,4,opt,name=closeRequests,proto3" json:"closeRequests,omitempty"`
|
|
Error string `protobuf:"bytes,5,opt,name=error,proto3" json:"error,omitempty"`
|
|
SubCloseRequests string `protobuf:"bytes,6,opt,name=subCloseRequests,proto3" json:"subCloseRequests,omitempty"`
|
|
PublicKey string `protobuf:"bytes,100,opt,name=publicKey,proto3" json:"publicKey,omitempty"`
|
|
}
|
|
|
|
func (m *ConnectResponse) Reset() { *m = ConnectResponse{} }
|
|
func (m *ConnectResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*ConnectResponse) ProtoMessage() {}
|
|
|
|
// Protocol for a client to subscribe
|
|
type SubscriptionRequest struct {
|
|
ClientID string `protobuf:"bytes,1,opt,name=clientID,proto3" json:"clientID,omitempty"`
|
|
Subject string `protobuf:"bytes,2,opt,name=subject,proto3" json:"subject,omitempty"`
|
|
QGroup string `protobuf:"bytes,3,opt,name=qGroup,proto3" json:"qGroup,omitempty"`
|
|
Inbox string `protobuf:"bytes,4,opt,name=inbox,proto3" json:"inbox,omitempty"`
|
|
MaxInFlight int32 `protobuf:"varint,5,opt,name=maxInFlight,proto3" json:"maxInFlight,omitempty"`
|
|
AckWaitInSecs int32 `protobuf:"varint,6,opt,name=ackWaitInSecs,proto3" json:"ackWaitInSecs,omitempty"`
|
|
DurableName string `protobuf:"bytes,7,opt,name=durableName,proto3" json:"durableName,omitempty"`
|
|
StartPosition StartPosition `protobuf:"varint,10,opt,name=startPosition,proto3,enum=pb.StartPosition" json:"startPosition,omitempty"`
|
|
StartSequence uint64 `protobuf:"varint,11,opt,name=startSequence,proto3" json:"startSequence,omitempty"`
|
|
StartTimeDelta int64 `protobuf:"varint,12,opt,name=startTimeDelta,proto3" json:"startTimeDelta,omitempty"`
|
|
}
|
|
|
|
func (m *SubscriptionRequest) Reset() { *m = SubscriptionRequest{} }
|
|
func (m *SubscriptionRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*SubscriptionRequest) ProtoMessage() {}
|
|
|
|
// Response for SubscriptionRequest and UnsubscribeRequests
|
|
type SubscriptionResponse struct {
|
|
AckInbox string `protobuf:"bytes,2,opt,name=ackInbox,proto3" json:"ackInbox,omitempty"`
|
|
Error string `protobuf:"bytes,3,opt,name=error,proto3" json:"error,omitempty"`
|
|
}
|
|
|
|
func (m *SubscriptionResponse) Reset() { *m = SubscriptionResponse{} }
|
|
func (m *SubscriptionResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*SubscriptionResponse) ProtoMessage() {}
|
|
|
|
// Protocol for a clients to unsubscribe. Will return a SubscriptionResponse
|
|
type UnsubscribeRequest struct {
|
|
ClientID string `protobuf:"bytes,1,opt,name=clientID,proto3" json:"clientID,omitempty"`
|
|
Subject string `protobuf:"bytes,2,opt,name=subject,proto3" json:"subject,omitempty"`
|
|
Inbox string `protobuf:"bytes,3,opt,name=inbox,proto3" json:"inbox,omitempty"`
|
|
DurableName string `protobuf:"bytes,4,opt,name=durableName,proto3" json:"durableName,omitempty"`
|
|
}
|
|
|
|
func (m *UnsubscribeRequest) Reset() { *m = UnsubscribeRequest{} }
|
|
func (m *UnsubscribeRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*UnsubscribeRequest) ProtoMessage() {}
|
|
|
|
// Protocol for a client to close a connection
|
|
type CloseRequest struct {
|
|
ClientID string `protobuf:"bytes,1,opt,name=clientID,proto3" json:"clientID,omitempty"`
|
|
}
|
|
|
|
func (m *CloseRequest) Reset() { *m = CloseRequest{} }
|
|
func (m *CloseRequest) String() string { return proto.CompactTextString(m) }
|
|
func (*CloseRequest) ProtoMessage() {}
|
|
|
|
// Response for CloseRequest
|
|
type CloseResponse struct {
|
|
Error string `protobuf:"bytes,1,opt,name=error,proto3" json:"error,omitempty"`
|
|
}
|
|
|
|
func (m *CloseResponse) Reset() { *m = CloseResponse{} }
|
|
func (m *CloseResponse) String() string { return proto.CompactTextString(m) }
|
|
func (*CloseResponse) ProtoMessage() {}
|
|
|
|
func init() {
|
|
proto.RegisterType((*PubMsg)(nil), "pb.PubMsg")
|
|
proto.RegisterType((*PubAck)(nil), "pb.PubAck")
|
|
proto.RegisterType((*MsgProto)(nil), "pb.MsgProto")
|
|
proto.RegisterType((*Ack)(nil), "pb.Ack")
|
|
proto.RegisterType((*ConnectRequest)(nil), "pb.ConnectRequest")
|
|
proto.RegisterType((*ConnectResponse)(nil), "pb.ConnectResponse")
|
|
proto.RegisterType((*SubscriptionRequest)(nil), "pb.SubscriptionRequest")
|
|
proto.RegisterType((*SubscriptionResponse)(nil), "pb.SubscriptionResponse")
|
|
proto.RegisterType((*UnsubscribeRequest)(nil), "pb.UnsubscribeRequest")
|
|
proto.RegisterType((*CloseRequest)(nil), "pb.CloseRequest")
|
|
proto.RegisterType((*CloseResponse)(nil), "pb.CloseResponse")
|
|
proto.RegisterEnum("pb.StartPosition", StartPosition_name, StartPosition_value)
|
|
}
|
|
func (m *PubMsg) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *PubMsg) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ClientID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.ClientID)))
|
|
i += copy(data[i:], m.ClientID)
|
|
}
|
|
if len(m.Guid) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Guid)))
|
|
i += copy(data[i:], m.Guid)
|
|
}
|
|
if len(m.Subject) > 0 {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Subject)))
|
|
i += copy(data[i:], m.Subject)
|
|
}
|
|
if len(m.Reply) > 0 {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Reply)))
|
|
i += copy(data[i:], m.Reply)
|
|
}
|
|
if m.Data != nil {
|
|
if len(m.Data) > 0 {
|
|
data[i] = 0x2a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Data)))
|
|
i += copy(data[i:], m.Data)
|
|
}
|
|
}
|
|
if m.Sha256 != nil {
|
|
if len(m.Sha256) > 0 {
|
|
data[i] = 0x52
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Sha256)))
|
|
i += copy(data[i:], m.Sha256)
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *PubAck) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *PubAck) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.Guid) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Guid)))
|
|
i += copy(data[i:], m.Guid)
|
|
}
|
|
if len(m.Error) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Error)))
|
|
i += copy(data[i:], m.Error)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *MsgProto) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *MsgProto) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.Sequence != 0 {
|
|
data[i] = 0x8
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.Sequence))
|
|
}
|
|
if len(m.Subject) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Subject)))
|
|
i += copy(data[i:], m.Subject)
|
|
}
|
|
if len(m.Reply) > 0 {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Reply)))
|
|
i += copy(data[i:], m.Reply)
|
|
}
|
|
if m.Data != nil {
|
|
if len(m.Data) > 0 {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Data)))
|
|
i += copy(data[i:], m.Data)
|
|
}
|
|
}
|
|
if m.Timestamp != 0 {
|
|
data[i] = 0x28
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.Timestamp))
|
|
}
|
|
if m.Redelivered {
|
|
data[i] = 0x30
|
|
i++
|
|
if m.Redelivered {
|
|
data[i] = 1
|
|
} else {
|
|
data[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
if m.CRC32 != 0 {
|
|
data[i] = 0x50
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.CRC32))
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Ack) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *Ack) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.Subject) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Subject)))
|
|
i += copy(data[i:], m.Subject)
|
|
}
|
|
if m.Sequence != 0 {
|
|
data[i] = 0x10
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.Sequence))
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *ConnectRequest) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *ConnectRequest) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ClientID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.ClientID)))
|
|
i += copy(data[i:], m.ClientID)
|
|
}
|
|
if len(m.HeartbeatInbox) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.HeartbeatInbox)))
|
|
i += copy(data[i:], m.HeartbeatInbox)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *ConnectResponse) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *ConnectResponse) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.PubPrefix) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.PubPrefix)))
|
|
i += copy(data[i:], m.PubPrefix)
|
|
}
|
|
if len(m.SubRequests) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.SubRequests)))
|
|
i += copy(data[i:], m.SubRequests)
|
|
}
|
|
if len(m.UnsubRequests) > 0 {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.UnsubRequests)))
|
|
i += copy(data[i:], m.UnsubRequests)
|
|
}
|
|
if len(m.CloseRequests) > 0 {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.CloseRequests)))
|
|
i += copy(data[i:], m.CloseRequests)
|
|
}
|
|
if len(m.Error) > 0 {
|
|
data[i] = 0x2a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Error)))
|
|
i += copy(data[i:], m.Error)
|
|
}
|
|
if len(m.SubCloseRequests) > 0 {
|
|
data[i] = 0x32
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.SubCloseRequests)))
|
|
i += copy(data[i:], m.SubCloseRequests)
|
|
}
|
|
if len(m.PublicKey) > 0 {
|
|
data[i] = 0xa2
|
|
i++
|
|
data[i] = 0x6
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.PublicKey)))
|
|
i += copy(data[i:], m.PublicKey)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *SubscriptionRequest) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *SubscriptionRequest) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ClientID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.ClientID)))
|
|
i += copy(data[i:], m.ClientID)
|
|
}
|
|
if len(m.Subject) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Subject)))
|
|
i += copy(data[i:], m.Subject)
|
|
}
|
|
if len(m.QGroup) > 0 {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.QGroup)))
|
|
i += copy(data[i:], m.QGroup)
|
|
}
|
|
if len(m.Inbox) > 0 {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Inbox)))
|
|
i += copy(data[i:], m.Inbox)
|
|
}
|
|
if m.MaxInFlight != 0 {
|
|
data[i] = 0x28
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.MaxInFlight))
|
|
}
|
|
if m.AckWaitInSecs != 0 {
|
|
data[i] = 0x30
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.AckWaitInSecs))
|
|
}
|
|
if len(m.DurableName) > 0 {
|
|
data[i] = 0x3a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.DurableName)))
|
|
i += copy(data[i:], m.DurableName)
|
|
}
|
|
if m.StartPosition != 0 {
|
|
data[i] = 0x50
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.StartPosition))
|
|
}
|
|
if m.StartSequence != 0 {
|
|
data[i] = 0x58
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.StartSequence))
|
|
}
|
|
if m.StartTimeDelta != 0 {
|
|
data[i] = 0x60
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(m.StartTimeDelta))
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *SubscriptionResponse) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *SubscriptionResponse) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.AckInbox) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.AckInbox)))
|
|
i += copy(data[i:], m.AckInbox)
|
|
}
|
|
if len(m.Error) > 0 {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Error)))
|
|
i += copy(data[i:], m.Error)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *UnsubscribeRequest) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *UnsubscribeRequest) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ClientID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.ClientID)))
|
|
i += copy(data[i:], m.ClientID)
|
|
}
|
|
if len(m.Subject) > 0 {
|
|
data[i] = 0x12
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Subject)))
|
|
i += copy(data[i:], m.Subject)
|
|
}
|
|
if len(m.Inbox) > 0 {
|
|
data[i] = 0x1a
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Inbox)))
|
|
i += copy(data[i:], m.Inbox)
|
|
}
|
|
if len(m.DurableName) > 0 {
|
|
data[i] = 0x22
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.DurableName)))
|
|
i += copy(data[i:], m.DurableName)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *CloseRequest) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *CloseRequest) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ClientID) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.ClientID)))
|
|
i += copy(data[i:], m.ClientID)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *CloseResponse) Marshal() (data []byte, err error) {
|
|
size := m.Size()
|
|
data = make([]byte, size)
|
|
n, err := m.MarshalTo(data)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return data[:n], nil
|
|
}
|
|
|
|
func (m *CloseResponse) MarshalTo(data []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.Error) > 0 {
|
|
data[i] = 0xa
|
|
i++
|
|
i = encodeVarintProtocol(data, i, uint64(len(m.Error)))
|
|
i += copy(data[i:], m.Error)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func encodeFixed64Protocol(data []byte, offset int, v uint64) int {
|
|
data[offset] = uint8(v)
|
|
data[offset+1] = uint8(v >> 8)
|
|
data[offset+2] = uint8(v >> 16)
|
|
data[offset+3] = uint8(v >> 24)
|
|
data[offset+4] = uint8(v >> 32)
|
|
data[offset+5] = uint8(v >> 40)
|
|
data[offset+6] = uint8(v >> 48)
|
|
data[offset+7] = uint8(v >> 56)
|
|
return offset + 8
|
|
}
|
|
func encodeFixed32Protocol(data []byte, offset int, v uint32) int {
|
|
data[offset] = uint8(v)
|
|
data[offset+1] = uint8(v >> 8)
|
|
data[offset+2] = uint8(v >> 16)
|
|
data[offset+3] = uint8(v >> 24)
|
|
return offset + 4
|
|
}
|
|
func encodeVarintProtocol(data []byte, offset int, v uint64) int {
|
|
for v >= 1<<7 {
|
|
data[offset] = uint8(v&0x7f | 0x80)
|
|
v >>= 7
|
|
offset++
|
|
}
|
|
data[offset] = uint8(v)
|
|
return offset + 1
|
|
}
|
|
func (m *PubMsg) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ClientID)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Guid)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Subject)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Reply)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
if m.Data != nil {
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
}
|
|
if m.Sha256 != nil {
|
|
l = len(m.Sha256)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *PubAck) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.Guid)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Error)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *MsgProto) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if m.Sequence != 0 {
|
|
n += 1 + sovProtocol(uint64(m.Sequence))
|
|
}
|
|
l = len(m.Subject)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Reply)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
if m.Data != nil {
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
}
|
|
if m.Timestamp != 0 {
|
|
n += 1 + sovProtocol(uint64(m.Timestamp))
|
|
}
|
|
if m.Redelivered {
|
|
n += 2
|
|
}
|
|
if m.CRC32 != 0 {
|
|
n += 1 + sovProtocol(uint64(m.CRC32))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Ack) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.Subject)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
if m.Sequence != 0 {
|
|
n += 1 + sovProtocol(uint64(m.Sequence))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *ConnectRequest) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ClientID)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.HeartbeatInbox)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *ConnectResponse) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.PubPrefix)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.SubRequests)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.UnsubRequests)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.CloseRequests)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Error)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.SubCloseRequests)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.PublicKey)
|
|
if l > 0 {
|
|
n += 2 + l + sovProtocol(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *SubscriptionRequest) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ClientID)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Subject)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.QGroup)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Inbox)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
if m.MaxInFlight != 0 {
|
|
n += 1 + sovProtocol(uint64(m.MaxInFlight))
|
|
}
|
|
if m.AckWaitInSecs != 0 {
|
|
n += 1 + sovProtocol(uint64(m.AckWaitInSecs))
|
|
}
|
|
l = len(m.DurableName)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
if m.StartPosition != 0 {
|
|
n += 1 + sovProtocol(uint64(m.StartPosition))
|
|
}
|
|
if m.StartSequence != 0 {
|
|
n += 1 + sovProtocol(uint64(m.StartSequence))
|
|
}
|
|
if m.StartTimeDelta != 0 {
|
|
n += 1 + sovProtocol(uint64(m.StartTimeDelta))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *SubscriptionResponse) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.AckInbox)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Error)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *UnsubscribeRequest) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ClientID)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Subject)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.Inbox)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
l = len(m.DurableName)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *CloseRequest) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ClientID)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *CloseResponse) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.Error)
|
|
if l > 0 {
|
|
n += 1 + l + sovProtocol(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func sovProtocol(x uint64) (n int) {
|
|
for {
|
|
n++
|
|
x >>= 7
|
|
if x == 0 {
|
|
break
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
func sozProtocol(x uint64) (n int) {
|
|
return sovProtocol(uint64((x << 1) ^ uint64((int64(x) >> 63))))
|
|
}
|
|
func (m *PubMsg) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: PubMsg: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: PubMsg: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ClientID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ClientID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Guid", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Guid = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Subject", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Subject = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Reply", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Reply = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
byteLen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], data[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Sha256", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
byteLen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Sha256 = append(m.Sha256[:0], data[iNdEx:postIndex]...)
|
|
if m.Sha256 == nil {
|
|
m.Sha256 = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *PubAck) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: PubAck: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: PubAck: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Guid", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Guid = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Error", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Error = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *MsgProto) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: MsgProto: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: MsgProto: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Sequence", wireType)
|
|
}
|
|
m.Sequence = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.Sequence |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Subject", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Subject = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Reply", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Reply = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
byteLen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], data[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Timestamp", wireType)
|
|
}
|
|
m.Timestamp = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.Timestamp |= (int64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 6:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Redelivered", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Redelivered = bool(v != 0)
|
|
case 10:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CRC32", wireType)
|
|
}
|
|
m.CRC32 = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.CRC32 |= (uint32(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Ack) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Ack: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Ack: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Subject", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Subject = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Sequence", wireType)
|
|
}
|
|
m.Sequence = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.Sequence |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ConnectRequest) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ConnectRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ConnectRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ClientID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ClientID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field HeartbeatInbox", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.HeartbeatInbox = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ConnectResponse) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ConnectResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ConnectResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field PubPrefix", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.PubPrefix = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SubRequests", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.SubRequests = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field UnsubRequests", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.UnsubRequests = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CloseRequests", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.CloseRequests = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Error", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Error = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SubCloseRequests", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.SubCloseRequests = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 100:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field PublicKey", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.PublicKey = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SubscriptionRequest) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SubscriptionRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SubscriptionRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ClientID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ClientID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Subject", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Subject = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field QGroup", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.QGroup = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Inbox", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Inbox = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field MaxInFlight", wireType)
|
|
}
|
|
m.MaxInFlight = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.MaxInFlight |= (int32(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 6:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AckWaitInSecs", wireType)
|
|
}
|
|
m.AckWaitInSecs = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.AckWaitInSecs |= (int32(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DurableName", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.DurableName = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field StartPosition", wireType)
|
|
}
|
|
m.StartPosition = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.StartPosition |= (StartPosition(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 11:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field StartSequence", wireType)
|
|
}
|
|
m.StartSequence = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.StartSequence |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 12:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field StartTimeDelta", wireType)
|
|
}
|
|
m.StartTimeDelta = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
m.StartTimeDelta |= (int64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SubscriptionResponse) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SubscriptionResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SubscriptionResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AckInbox", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.AckInbox = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Error", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Error = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *UnsubscribeRequest) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: UnsubscribeRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: UnsubscribeRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ClientID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ClientID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Subject", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Subject = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Inbox", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Inbox = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DurableName", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.DurableName = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *CloseRequest) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: CloseRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: CloseRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ClientID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ClientID = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *CloseResponse) Unmarshal(data []byte) error {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: CloseResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: CloseResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Error", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Error = string(data[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipProtocol(data[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthProtocol
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func skipProtocol(data []byte) (n int, err error) {
|
|
l := len(data)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
wireType := int(wire & 0x7)
|
|
switch wireType {
|
|
case 0:
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx++
|
|
if data[iNdEx-1] < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
return iNdEx, nil
|
|
case 1:
|
|
iNdEx += 8
|
|
return iNdEx, nil
|
|
case 2:
|
|
var length int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
length |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
iNdEx += length
|
|
if length < 0 {
|
|
return 0, ErrInvalidLengthProtocol
|
|
}
|
|
return iNdEx, nil
|
|
case 3:
|
|
for {
|
|
var innerWire uint64
|
|
var start int = iNdEx
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowProtocol
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := data[iNdEx]
|
|
iNdEx++
|
|
innerWire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
innerWireType := int(innerWire & 0x7)
|
|
if innerWireType == 4 {
|
|
break
|
|
}
|
|
next, err := skipProtocol(data[start:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
iNdEx = start + next
|
|
}
|
|
return iNdEx, nil
|
|
case 4:
|
|
return iNdEx, nil
|
|
case 5:
|
|
iNdEx += 4
|
|
return iNdEx, nil
|
|
default:
|
|
return 0, fmt.Errorf("proto: illegal wireType %d", wireType)
|
|
}
|
|
}
|
|
panic("unreachable")
|
|
}
|
|
|
|
var (
|
|
ErrInvalidLengthProtocol = fmt.Errorf("proto: negative length found during unmarshaling")
|
|
ErrIntOverflowProtocol = fmt.Errorf("proto: integer overflow")
|
|
)
|