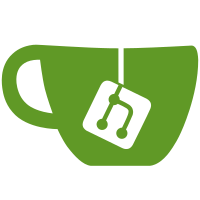
* Add a new lint rule to the Makefile Signed-off-by: Kenfe-Mickael Laventure <mickael.laventure@gmail.com> * Fix linter errors Signed-off-by: Kenfe-Mickael Laventure <mickael.laventure@gmail.com> * Allow replacing the default apt mirror Signed-off-by: Kenfe-Mickael Laventure <mickael.laventure@gmail.com>
28 lines
497 B
Go
28 lines
497 B
Go
package supervisor
|
|
|
|
import "os"
|
|
|
|
// SignalTask holds needed parameters to signal a container
|
|
type SignalTask struct {
|
|
baseTask
|
|
ID string
|
|
PID string
|
|
Signal os.Signal
|
|
}
|
|
|
|
func (s *Supervisor) signal(t *SignalTask) error {
|
|
i, ok := s.containers[t.ID]
|
|
if !ok {
|
|
return ErrContainerNotFound
|
|
}
|
|
processes, err := i.container.Processes()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
for _, p := range processes {
|
|
if p.ID() == t.PID {
|
|
return p.Signal(t.Signal)
|
|
}
|
|
}
|
|
return ErrProcessNotFound
|
|
}
|