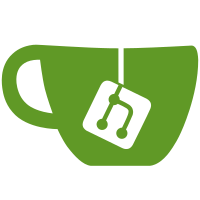
Signed-off-by: Jacek J. Łakis <jacek.lakis@intel.com> Signed-off-by: Samuel Ortiz <sameo@linux.intel.com>
212 lines
7.5 KiB
Protocol Buffer
212 lines
7.5 KiB
Protocol Buffer
/*
|
|
Copyright 2017 The Kubernetes Authors.
|
|
|
|
Licensed under the Apache License, Version 2.0 (the "License");
|
|
you may not use this file except in compliance with the License.
|
|
You may obtain a copy of the License at
|
|
|
|
http://www.apache.org/licenses/LICENSE-2.0
|
|
|
|
Unless required by applicable law or agreed to in writing, software
|
|
distributed under the License is distributed on an "AS IS" BASIS,
|
|
WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
See the License for the specific language governing permissions and
|
|
limitations under the License.
|
|
*/
|
|
|
|
|
|
// This file was autogenerated by go-to-protobuf. Do not edit it manually!
|
|
|
|
syntax = 'proto2';
|
|
|
|
package k8s.io.apiserver.pkg.apis.example.v1;
|
|
|
|
import "k8s.io/apimachinery/pkg/apis/meta/v1/generated.proto";
|
|
import "k8s.io/apimachinery/pkg/runtime/generated.proto";
|
|
import "k8s.io/apimachinery/pkg/runtime/schema/generated.proto";
|
|
import "k8s.io/apimachinery/pkg/util/intstr/generated.proto";
|
|
|
|
// Package-wide variables from generator "generated".
|
|
option go_package = "v1";
|
|
|
|
// Pod is a collection of containers, used as either input (create, update) or as output (list, get).
|
|
message Pod {
|
|
// Standard object's metadata.
|
|
// More info: http://releases.k8s.io/HEAD/docs/devel/api-conventions.md#metadata
|
|
// +optional
|
|
optional k8s.io.apimachinery.pkg.apis.meta.v1.ObjectMeta metadata = 1;
|
|
|
|
// Specification of the desired behavior of the pod.
|
|
// More info: http://releases.k8s.io/HEAD/docs/devel/api-conventions.md#spec-and-status
|
|
// +optional
|
|
optional PodSpec spec = 2;
|
|
|
|
// Most recently observed status of the pod.
|
|
// This data may not be up to date.
|
|
// Populated by the system.
|
|
// Read-only.
|
|
// More info: http://releases.k8s.io/HEAD/docs/devel/api-conventions.md#spec-and-status
|
|
// +optional
|
|
optional PodStatus status = 3;
|
|
}
|
|
|
|
message PodCondition {
|
|
// Type is the type of the condition.
|
|
// Currently only Ready.
|
|
// More info: http://kubernetes.io/docs/user-guide/pod-states#pod-conditions
|
|
optional string type = 1;
|
|
|
|
// Status is the status of the condition.
|
|
// Can be True, False, Unknown.
|
|
// More info: http://kubernetes.io/docs/user-guide/pod-states#pod-conditions
|
|
optional string status = 2;
|
|
|
|
// Last time we probed the condition.
|
|
// +optional
|
|
optional k8s.io.apimachinery.pkg.apis.meta.v1.Time lastProbeTime = 3;
|
|
|
|
// Last time the condition transitioned from one status to another.
|
|
// +optional
|
|
optional k8s.io.apimachinery.pkg.apis.meta.v1.Time lastTransitionTime = 4;
|
|
|
|
// Unique, one-word, CamelCase reason for the condition's last transition.
|
|
// +optional
|
|
optional string reason = 5;
|
|
|
|
// Human-readable message indicating details about last transition.
|
|
// +optional
|
|
optional string message = 6;
|
|
}
|
|
|
|
// PodList is a list of Pods.
|
|
message PodList {
|
|
// Standard list metadata.
|
|
// More info: http://releases.k8s.io/HEAD/docs/devel/api-conventions.md#types-kinds
|
|
// +optional
|
|
optional k8s.io.apimachinery.pkg.apis.meta.v1.ListMeta metadata = 1;
|
|
|
|
// List of pods.
|
|
// More info: http://kubernetes.io/docs/user-guide/pods
|
|
repeated Pod items = 2;
|
|
}
|
|
|
|
// PodSpec is a description of a pod
|
|
message PodSpec {
|
|
// Restart policy for all containers within the pod.
|
|
// One of Always, OnFailure, Never.
|
|
// Default to Always.
|
|
// More info: http://kubernetes.io/docs/user-guide/pod-states#restartpolicy
|
|
// +optional
|
|
optional string restartPolicy = 3;
|
|
|
|
// Optional duration in seconds the pod needs to terminate gracefully. May be decreased in delete request.
|
|
// Value must be non-negative integer. The value zero indicates delete immediately.
|
|
// If this value is nil, the default grace period will be used instead.
|
|
// The grace period is the duration in seconds after the processes running in the pod are sent
|
|
// a termination signal and the time when the processes are forcibly halted with a kill signal.
|
|
// Set this value longer than the expected cleanup time for your process.
|
|
// Defaults to 30 seconds.
|
|
// +optional
|
|
optional int64 terminationGracePeriodSeconds = 4;
|
|
|
|
// Optional duration in seconds the pod may be active on the node relative to
|
|
// StartTime before the system will actively try to mark it failed and kill associated containers.
|
|
// Value must be a positive integer.
|
|
// +optional
|
|
optional int64 activeDeadlineSeconds = 5;
|
|
|
|
// NodeSelector is a selector which must be true for the pod to fit on a node.
|
|
// Selector which must match a node's labels for the pod to be scheduled on that node.
|
|
// More info: http://kubernetes.io/docs/user-guide/node-selection/README
|
|
// +optional
|
|
map<string, string> nodeSelector = 7;
|
|
|
|
// ServiceAccountName is the name of the ServiceAccount to use to run this pod.
|
|
// More info: http://releases.k8s.io/HEAD/docs/design/service_accounts.md
|
|
// +optional
|
|
optional string serviceAccountName = 8;
|
|
|
|
// DeprecatedServiceAccount is a depreciated alias for ServiceAccountName.
|
|
// Deprecated: Use serviceAccountName instead.
|
|
// +k8s:conversion-gen=false
|
|
// +optional
|
|
optional string serviceAccount = 9;
|
|
|
|
// NodeName is a request to schedule this pod onto a specific node. If it is non-empty,
|
|
// the scheduler simply schedules this pod onto that node, assuming that it fits resource
|
|
// requirements.
|
|
// +optional
|
|
optional string nodeName = 10;
|
|
|
|
// Host networking requested for this pod. Use the host's network namespace.
|
|
// If this option is set, the ports that will be used must be specified.
|
|
// Default to false.
|
|
// +k8s:conversion-gen=false
|
|
// +optional
|
|
optional bool hostNetwork = 11;
|
|
|
|
// Use the host's pid namespace.
|
|
// Optional: Default to false.
|
|
// +k8s:conversion-gen=false
|
|
// +optional
|
|
optional bool hostPID = 12;
|
|
|
|
// Use the host's ipc namespace.
|
|
// Optional: Default to false.
|
|
// +k8s:conversion-gen=false
|
|
// +optional
|
|
optional bool hostIPC = 13;
|
|
|
|
// Specifies the hostname of the Pod
|
|
// If not specified, the pod's hostname will be set to a system-defined value.
|
|
// +optional
|
|
optional string hostname = 16;
|
|
|
|
// If specified, the fully qualified Pod hostname will be "<hostname>.<subdomain>.<pod namespace>.svc.<cluster domain>".
|
|
// If not specified, the pod will not have a domainname at all.
|
|
// +optional
|
|
optional string subdomain = 17;
|
|
|
|
// If specified, the pod will be dispatched by specified scheduler.
|
|
// If not specified, the pod will be dispatched by default scheduler.
|
|
// +optional
|
|
optional string schedulername = 19;
|
|
}
|
|
|
|
// PodStatus represents information about the status of a pod. Status may trail the actual
|
|
// state of a system.
|
|
message PodStatus {
|
|
// Current condition of the pod.
|
|
// More info: http://kubernetes.io/docs/user-guide/pod-states#pod-phase
|
|
// +optional
|
|
optional string phase = 1;
|
|
|
|
// Current service state of pod.
|
|
// More info: http://kubernetes.io/docs/user-guide/pod-states#pod-conditions
|
|
// +optional
|
|
repeated PodCondition conditions = 2;
|
|
|
|
// A human readable message indicating details about why the pod is in this condition.
|
|
// +optional
|
|
optional string message = 3;
|
|
|
|
// A brief CamelCase message indicating details about why the pod is in this state.
|
|
// e.g. 'OutOfDisk'
|
|
// +optional
|
|
optional string reason = 4;
|
|
|
|
// IP address of the host to which the pod is assigned. Empty if not yet scheduled.
|
|
// +optional
|
|
optional string hostIP = 5;
|
|
|
|
// IP address allocated to the pod. Routable at least within the cluster.
|
|
// Empty if not yet allocated.
|
|
// +optional
|
|
optional string podIP = 6;
|
|
|
|
// RFC 3339 date and time at which the object was acknowledged by the Kubelet.
|
|
// This is before the Kubelet pulled the container image(s) for the pod.
|
|
// +optional
|
|
optional k8s.io.apimachinery.pkg.apis.meta.v1.Time startTime = 7;
|
|
}
|
|
|