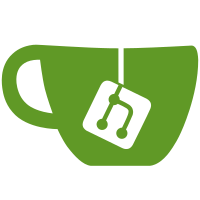
The storage library uses github.com/pkg/errors to wrap errors that it returns from many of its functions, so when passing them to os.IsNotExist() or comparing them to specific errors defined in the storage library, unwrap them using errors.Cause(). Signed-off-by: Nalin Dahyabhai <nalin@redhat.com>
53 lines
1.4 KiB
Go
53 lines
1.4 KiB
Go
package server
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
"github.com/Sirupsen/logrus"
|
|
"github.com/containers/storage"
|
|
"github.com/pkg/errors"
|
|
"golang.org/x/net/context"
|
|
pb "k8s.io/kubernetes/pkg/kubelet/api/v1alpha1/runtime"
|
|
)
|
|
|
|
// ImageStatus returns the status of the image.
|
|
func (s *Server) ImageStatus(ctx context.Context, req *pb.ImageStatusRequest) (*pb.ImageStatusResponse, error) {
|
|
logrus.Debugf("ImageStatusRequest: %+v", req)
|
|
image := ""
|
|
img := req.GetImage()
|
|
if img != nil {
|
|
image = img.Image
|
|
}
|
|
if image == "" {
|
|
return nil, fmt.Errorf("no image specified")
|
|
}
|
|
images, err := s.StorageImageServer().ResolveNames(image)
|
|
if err != nil {
|
|
// This means we got an image ID
|
|
if strings.Contains(err.Error(), "cannot specify 64-byte hexadecimal strings") {
|
|
images = append(images, image)
|
|
} else {
|
|
return nil, err
|
|
}
|
|
}
|
|
// match just the first registry as that's what kube meant
|
|
image = images[0]
|
|
status, err := s.StorageImageServer().ImageStatus(s.ImageContext(), image)
|
|
if err != nil {
|
|
if errors.Cause(err) == storage.ErrImageUnknown {
|
|
return &pb.ImageStatusResponse{}, nil
|
|
}
|
|
return nil, err
|
|
}
|
|
resp := &pb.ImageStatusResponse{
|
|
Image: &pb.Image{
|
|
Id: status.ID,
|
|
RepoTags: status.Names,
|
|
Size_: *status.Size,
|
|
// TODO: https://github.com/kubernetes-incubator/cri-o/issues/531
|
|
},
|
|
}
|
|
logrus.Debugf("ImageStatusResponse: %+v", resp)
|
|
return resp, nil
|
|
}
|