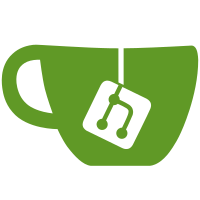
Also set default level of logging to errors, we should not see info messages in the kpod command line. While adding this patch, I found missing options in kpod command line and bash completions, so I added them in. Also fixed some sorting issues in the way commands are displayer in help or in bash completions. Finally fixed the error message to be output on failure using logrus.Errorf, so we don't get the stack any longer. Also updated README.md with missing kpod commands. Signed-off-by: Daniel J Walsh <dwalsh@redhat.com>
317 lines
5.5 KiB
Bash
317 lines
5.5 KiB
Bash
#! /bin/bash
|
|
|
|
: ${PROG:=$(basename ${BASH_SOURCE})}
|
|
|
|
__kpod_list_images() {
|
|
COMPREPLY=($(compgen -W "$(kpod images -q)" -- $cur))
|
|
}
|
|
|
|
_kpod_history() {
|
|
local options_with_args="
|
|
--format
|
|
"
|
|
local boolean_options="
|
|
--human -H
|
|
--no-trunc
|
|
--quiet -q
|
|
--json
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=($(compgen -W "$boolean_options $options_with_args" -- "$cur"))
|
|
;;
|
|
*)
|
|
__kpod_list_images
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_kpod_info() {
|
|
local boolean_options="
|
|
--help
|
|
-h
|
|
--json
|
|
--debug
|
|
"
|
|
local options_with_args="
|
|
"
|
|
|
|
local all_options="$options_with_args $boolean_options"
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=($(compgen -W "$boolean_options $options_with_args" -- "$cur"))
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_kpod_images() {
|
|
local boolean_options="
|
|
--help
|
|
-h
|
|
--quiet
|
|
-q
|
|
--noheading
|
|
-n
|
|
--no-trunc
|
|
--digests
|
|
--format
|
|
--filter
|
|
-f
|
|
"
|
|
local options_with_args="
|
|
"
|
|
|
|
local all_options="$options_with_args $boolean_options"
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=($(compgen -W "$boolean_options $options_with_args" -- "$cur"))
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_kpod_launch() {
|
|
local options_with_args="
|
|
"
|
|
local boolean_options="
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
}
|
|
|
|
_kpod_pull() {
|
|
local options_with_args="
|
|
"
|
|
local boolean_options="
|
|
--all-tags -a
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
}
|
|
|
|
_kpod_unmount() {
|
|
_kpod_umount $@
|
|
}
|
|
|
|
_kpod_umount() {
|
|
local boolean_options="
|
|
--help
|
|
-h
|
|
"
|
|
local options_with_args="
|
|
"
|
|
|
|
local all_options="$options_with_args $boolean_options"
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=($(compgen -W "$boolean_options $options_with_args" -- "$cur"))
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_kpod_mount() {
|
|
local boolean_options="
|
|
--help
|
|
-h
|
|
--notruncate
|
|
"
|
|
|
|
local options_with_args="
|
|
--label
|
|
--format
|
|
"
|
|
|
|
local all_options="$options_with_args $boolean_options"
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=($(compgen -W "$boolean_options $options_with_args" -- "$cur"))
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_kpod_push() {
|
|
local boolean_options="
|
|
--disable-compression
|
|
-D
|
|
--quiet
|
|
-q
|
|
--signature-policy
|
|
--certs
|
|
--tls-verify
|
|
--remove-signatures
|
|
--sign-by
|
|
"
|
|
|
|
local options_with_args="
|
|
"
|
|
|
|
local all_options="$options_with_args $boolean_options"
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=($(compgen -W "$boolean_options $options_with_args" -- "$cur"))
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_kpod_rmi() {
|
|
local boolean_options="
|
|
--help
|
|
-h
|
|
--force
|
|
-f
|
|
"
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=($(compgen -W "$boolean_options $options_with_args" -- "$cur"))
|
|
;;
|
|
*)
|
|
__kpod_list_images
|
|
;;
|
|
esac
|
|
}
|
|
|
|
kpod_tag() {
|
|
local options_with_args="
|
|
"
|
|
local boolean_options="
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
}
|
|
|
|
_kpod_version() {
|
|
local options_with_args="
|
|
"
|
|
local boolean_options="
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
}
|
|
|
|
_kpod_save() {
|
|
local options_with_args="
|
|
--output -o
|
|
"
|
|
local boolean_options="
|
|
--quiet -q
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
}
|
|
|
|
_kpod_export() {
|
|
local options_with_args="
|
|
--output -o
|
|
"
|
|
local boolean_options="
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
}
|
|
|
|
_complete_() {
|
|
local options_with_args=$1
|
|
local boolean_options="$2 -h --help"
|
|
|
|
case "$prev" in
|
|
$options_with_args)
|
|
return
|
|
;;
|
|
esac
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=( $( compgen -W "$boolean_options $options_with_args" -- "$cur" ) )
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_kpod_load() {
|
|
local options_with_args="
|
|
--input -i
|
|
"
|
|
local boolean_options="
|
|
--quiet -q
|
|
"
|
|
_complete_ "$options_with_args" "$boolean_options"
|
|
}
|
|
|
|
_kpod_kpod() {
|
|
local options_with_args="
|
|
--config -c
|
|
--root
|
|
--runroot
|
|
--storage-driver
|
|
--storage-opt
|
|
"
|
|
local boolean_options="
|
|
--debug
|
|
--help -h
|
|
--version -v
|
|
"
|
|
commands="
|
|
history
|
|
images
|
|
info
|
|
launch
|
|
load
|
|
mount
|
|
pull
|
|
push
|
|
rmi
|
|
tag
|
|
umount
|
|
unmount
|
|
version
|
|
"
|
|
|
|
case "$prev" in
|
|
$main_options_with_args_glob )
|
|
return
|
|
;;
|
|
esac
|
|
|
|
case "$cur" in
|
|
-*)
|
|
COMPREPLY=( $( compgen -W "$boolean_options $options_with_args" -- "$cur" ) )
|
|
;;
|
|
*)
|
|
COMPREPLY=( $( compgen -W "${commands[*]} help" -- "$cur" ) )
|
|
;;
|
|
esac
|
|
}
|
|
|
|
_cli_bash_autocomplete() {
|
|
local cur opts base
|
|
|
|
COMPREPLY=()
|
|
cur="${COMP_WORDS[COMP_CWORD]}"
|
|
COMPREPLY=()
|
|
local cur prev words cword
|
|
|
|
_get_comp_words_by_ref -n : cur prev words cword
|
|
|
|
local command=${PROG} cpos=0
|
|
local counter=1
|
|
counter=1
|
|
while [ $counter -lt $cword ]; do
|
|
case "!${words[$counter]}" in
|
|
*)
|
|
command=$(echo "${words[$counter]}" | sed 's/-/_/g')
|
|
cpos=$counter
|
|
(( cpos++ ))
|
|
break
|
|
;;
|
|
esac
|
|
(( counter++ ))
|
|
done
|
|
|
|
local completions_func=_kpod_${command}
|
|
declare -F $completions_func >/dev/null && $completions_func
|
|
|
|
eval "$previous_extglob_setting"
|
|
return 0
|
|
}
|
|
|
|
complete -F _cli_bash_autocomplete $PROG
|