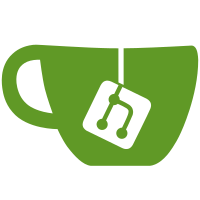
Signed-off-by: Jacek J. Łakis <jacek.lakis@intel.com> Signed-off-by: Samuel Ortiz <sameo@linux.intel.com>
73 lines
2.3 KiB
Go
73 lines
2.3 KiB
Go
// Copyright 2016 Google Inc. All Rights Reserved.
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
// This file supports generating unique IDs so that multiple test executions
|
|
// don't interfere with each other, and cleaning up old entities that may
|
|
// remain if tests exit early.
|
|
|
|
package testing
|
|
|
|
import (
|
|
"fmt"
|
|
"strconv"
|
|
"strings"
|
|
"time"
|
|
)
|
|
|
|
var (
|
|
startTime = time.Now()
|
|
uniqueIDCounter int
|
|
// Items older than expiredAge are remnants from previous tests and can be deleted.
|
|
expiredAge = 24 * time.Hour
|
|
)
|
|
|
|
// UniqueID generates unique IDs so tests don't interfere with each other.
|
|
// All unique IDs generated in the same test execution will have the same timestamp.
|
|
func UniqueID(prefix string) string {
|
|
uniqueIDCounter++
|
|
// Zero-pad the counter for lexical sort order.
|
|
return fmt.Sprintf("%s-t%d-%04d", prefix, startTime.UnixNano(), uniqueIDCounter)
|
|
}
|
|
|
|
// ExpiredUniqueIDs returns a subset of ids that are unique IDs as generated by
|
|
// UniqueID(prefix) and are older than expiredAge.
|
|
func ExpiredUniqueIDs(ids []string, prefix string) []string {
|
|
var expired []string
|
|
for _, id := range ids {
|
|
t, ok := extractTime(id, prefix)
|
|
if ok && time.Since(t) > expiredAge {
|
|
expired = append(expired, id)
|
|
}
|
|
}
|
|
return expired
|
|
}
|
|
|
|
// extractTime extracts the timestamp of s, which must begin with prefix and
|
|
// match the form generated by uniqueID. The second return value is true on
|
|
// success, false if there was a problem.
|
|
func extractTime(s, prefix string) (time.Time, bool) {
|
|
if !strings.HasPrefix(s, prefix+"-t") {
|
|
return time.Time{}, false
|
|
}
|
|
s = s[len(prefix)+2:]
|
|
i := strings.Index(s, "-")
|
|
if i < 0 {
|
|
return time.Time{}, false
|
|
}
|
|
nanos, err := strconv.ParseInt(s[:i], 10, 64)
|
|
if err != nil {
|
|
return time.Time{}, false
|
|
}
|
|
return time.Unix(0, nanos), true
|
|
}
|