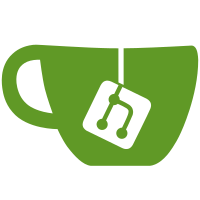
Kubelet can send cap add/drop ALL. Handle that in CRI-O as well. Also, this PR is re-vendoring runtime-tools to fix capabilities add to add caps to _all_ caps set **and** fix a shared memory issue (caps set were initialized with the same slice, if one modifies one slice, it's reflected on the other slices, the vendoring fixes this as well) Signed-off-by: Antonio Murdaca <runcom@redhat.com>
92 lines
2 KiB
Go
92 lines
2 KiB
Go
// Package error implements generic tooling for tracking RFC 2119
|
|
// violations and linking back to the appropriate specification section.
|
|
package error
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
)
|
|
|
|
// Level represents the RFC 2119 compliance levels
|
|
type Level int
|
|
|
|
const (
|
|
// MAY-level
|
|
|
|
// May represents 'MAY' in RFC 2119.
|
|
May Level = iota
|
|
// Optional represents 'OPTIONAL' in RFC 2119.
|
|
Optional
|
|
|
|
// SHOULD-level
|
|
|
|
// Should represents 'SHOULD' in RFC 2119.
|
|
Should
|
|
// ShouldNot represents 'SHOULD NOT' in RFC 2119.
|
|
ShouldNot
|
|
// Recommended represents 'RECOMMENDED' in RFC 2119.
|
|
Recommended
|
|
// NotRecommended represents 'NOT RECOMMENDED' in RFC 2119.
|
|
NotRecommended
|
|
|
|
// MUST-level
|
|
|
|
// Must represents 'MUST' in RFC 2119
|
|
Must
|
|
// MustNot represents 'MUST NOT' in RFC 2119.
|
|
MustNot
|
|
// Shall represents 'SHALL' in RFC 2119.
|
|
Shall
|
|
// ShallNot represents 'SHALL NOT' in RFC 2119.
|
|
ShallNot
|
|
// Required represents 'REQUIRED' in RFC 2119.
|
|
Required
|
|
)
|
|
|
|
// Error represents an error with compliance level and specification reference.
|
|
type Error struct {
|
|
// Level represents the RFC 2119 compliance level.
|
|
Level Level
|
|
|
|
// Reference is a URL for the violated specification requirement.
|
|
Reference string
|
|
|
|
// Err holds additional details about the violation.
|
|
Err error
|
|
}
|
|
|
|
// ParseLevel takes a string level and returns the RFC 2119 compliance level constant.
|
|
func ParseLevel(level string) (Level, error) {
|
|
switch strings.ToUpper(level) {
|
|
case "MAY":
|
|
fallthrough
|
|
case "OPTIONAL":
|
|
return May, nil
|
|
case "SHOULD":
|
|
fallthrough
|
|
case "SHOULDNOT":
|
|
fallthrough
|
|
case "RECOMMENDED":
|
|
fallthrough
|
|
case "NOTRECOMMENDED":
|
|
return Should, nil
|
|
case "MUST":
|
|
fallthrough
|
|
case "MUSTNOT":
|
|
fallthrough
|
|
case "SHALL":
|
|
fallthrough
|
|
case "SHALLNOT":
|
|
fallthrough
|
|
case "REQUIRED":
|
|
return Must, nil
|
|
}
|
|
|
|
var l Level
|
|
return l, fmt.Errorf("%q is not a valid compliance level", level)
|
|
}
|
|
|
|
// Error returns the error message with specification reference.
|
|
func (err *Error) Error() string {
|
|
return fmt.Sprintf("%s\nRefer to: %s", err.Err.Error(), err.Reference)
|
|
}
|