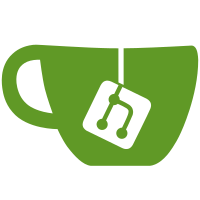
Move non-kubernetes-dependent portions of server struct to libkpod. So far, only the struct fields have been moved and not their dependent functions Signed-off-by: Ryan Cole <rcyoalne@gmail.com>
41 lines
882 B
Go
41 lines
882 B
Go
package server
|
|
|
|
import (
|
|
"golang.org/x/net/context"
|
|
pb "k8s.io/kubernetes/pkg/kubelet/api/v1alpha1/runtime"
|
|
)
|
|
|
|
// Status returns the status of the runtime
|
|
func (s *Server) Status(ctx context.Context, req *pb.StatusRequest) (*pb.StatusResponse, error) {
|
|
|
|
// Deal with Runtime conditions
|
|
runtimeReady, err := s.Runtime().RuntimeReady()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
networkReady, err := s.Runtime().NetworkReady()
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
// Use vendored strings
|
|
runtimeReadyConditionString := pb.RuntimeReady
|
|
networkReadyConditionString := pb.NetworkReady
|
|
|
|
resp := &pb.StatusResponse{
|
|
Status: &pb.RuntimeStatus{
|
|
Conditions: []*pb.RuntimeCondition{
|
|
{
|
|
Type: runtimeReadyConditionString,
|
|
Status: runtimeReady,
|
|
},
|
|
{
|
|
Type: networkReadyConditionString,
|
|
Status: networkReady,
|
|
},
|
|
},
|
|
},
|
|
}
|
|
|
|
return resp, nil
|
|
}
|