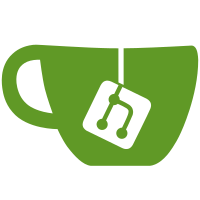
Vendor updated containers/image and containers/storage, along with any new dependencies they drag in, and updated versions of other dependencies that happen to get pulled in. github.com/coreos/go-systemd/daemon/SdNotify() now takes a boolean to control whether or not it unsets the NOTIFY_SOCKET variable from the calling process's environment. Adapt. github.com/opencontainers/runtime-tools/generate/Generator.AddProcessEnv() now takes the environment variable name and value as two arguments, not one. Adapt. Signed-off-by: Nalin Dahyabhai <nalin@redhat.com>
79 lines
1.8 KiB
Go
79 lines
1.8 KiB
Go
// Copyright 2015 CNI authors
|
|
//
|
|
// Licensed under the Apache License, Version 2.0 (the "License");
|
|
// you may not use this file except in compliance with the License.
|
|
// You may obtain a copy of the License at
|
|
//
|
|
// http://www.apache.org/licenses/LICENSE-2.0
|
|
//
|
|
// Unless required by applicable law or agreed to in writing, software
|
|
// distributed under the License is distributed on an "AS IS" BASIS,
|
|
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
// See the License for the specific language governing permissions and
|
|
// limitations under the License.
|
|
|
|
package invoke
|
|
|
|
import (
|
|
"os"
|
|
"strings"
|
|
)
|
|
|
|
type CNIArgs interface {
|
|
// For use with os/exec; i.e., return nil to inherit the
|
|
// environment from this process
|
|
AsEnv() []string
|
|
}
|
|
|
|
type inherited struct{}
|
|
|
|
var inheritArgsFromEnv inherited
|
|
|
|
func (_ *inherited) AsEnv() []string {
|
|
return nil
|
|
}
|
|
|
|
func ArgsFromEnv() CNIArgs {
|
|
return &inheritArgsFromEnv
|
|
}
|
|
|
|
type Args struct {
|
|
Command string
|
|
ContainerID string
|
|
NetNS string
|
|
PluginArgs [][2]string
|
|
PluginArgsStr string
|
|
IfName string
|
|
Path string
|
|
}
|
|
|
|
// Args implements the CNIArgs interface
|
|
var _ CNIArgs = &Args{}
|
|
|
|
func (args *Args) AsEnv() []string {
|
|
env := os.Environ()
|
|
pluginArgsStr := args.PluginArgsStr
|
|
if pluginArgsStr == "" {
|
|
pluginArgsStr = stringify(args.PluginArgs)
|
|
}
|
|
|
|
env = append(env,
|
|
"CNI_COMMAND="+args.Command,
|
|
"CNI_CONTAINERID="+args.ContainerID,
|
|
"CNI_NETNS="+args.NetNS,
|
|
"CNI_ARGS="+pluginArgsStr,
|
|
"CNI_IFNAME="+args.IfName,
|
|
"CNI_PATH="+args.Path)
|
|
return env
|
|
}
|
|
|
|
// taken from rkt/networking/net_plugin.go
|
|
func stringify(pluginArgs [][2]string) string {
|
|
entries := make([]string, len(pluginArgs))
|
|
|
|
for i, kv := range pluginArgs {
|
|
entries[i] = strings.Join(kv[:], "=")
|
|
}
|
|
|
|
return strings.Join(entries, ";")
|
|
}
|