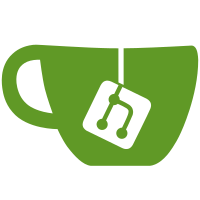
When a user enters a CLI with a StringFlags or StringSliceFlags and does not add a value the CLI mistakently takes the next option and uses it as a value. This usually ends up with an error like not enough options or others. Some times it could also succeed, with weird results. This patch looks for any values that begin with a "-" and return an error. Signed-off-by: Daniel J Walsh <dwalsh@redhat.com>
131 lines
3 KiB
Go
131 lines
3 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/containers/storage/pkg/archive"
|
|
"github.com/kubernetes-incubator/cri-o/cmd/kpod/formats"
|
|
"github.com/kubernetes-incubator/cri-o/libkpod"
|
|
"github.com/pkg/errors"
|
|
"github.com/urfave/cli"
|
|
)
|
|
|
|
type diffJSONOutput struct {
|
|
Changed []string `json:"changed,omitempty"`
|
|
Added []string `json:"added,omitempty"`
|
|
Deleted []string `json:"deleted,omitempty"`
|
|
}
|
|
|
|
type diffOutputParams struct {
|
|
Change archive.ChangeType
|
|
Path string
|
|
}
|
|
|
|
type stdoutStruct struct {
|
|
output []diffOutputParams
|
|
}
|
|
|
|
func (so stdoutStruct) Out() error {
|
|
for _, d := range so.output {
|
|
fmt.Printf("%s %s\n", d.Change, d.Path)
|
|
}
|
|
return nil
|
|
}
|
|
|
|
var (
|
|
diffFlags = []cli.Flag{
|
|
cli.BoolFlag{
|
|
Name: "archive",
|
|
Usage: "Save the diff as a tar archive",
|
|
Hidden: true,
|
|
},
|
|
cli.StringFlag{
|
|
Name: "format",
|
|
Usage: "Change the output format.",
|
|
},
|
|
}
|
|
diffDescription = fmt.Sprint(`Displays changes on a container or image's filesystem. The
|
|
container or image will be compared to its parent layer`)
|
|
|
|
diffCommand = cli.Command{
|
|
Name: "diff",
|
|
Usage: "Inspect changes on container's file systems",
|
|
Description: diffDescription,
|
|
Flags: diffFlags,
|
|
Action: diffCmd,
|
|
ArgsUsage: "ID-NAME",
|
|
}
|
|
)
|
|
|
|
func formatJSON(output []diffOutputParams) (diffJSONOutput, error) {
|
|
jsonStruct := diffJSONOutput{}
|
|
for _, output := range output {
|
|
switch output.Change {
|
|
case archive.ChangeModify:
|
|
jsonStruct.Changed = append(jsonStruct.Changed, output.Path)
|
|
case archive.ChangeAdd:
|
|
jsonStruct.Added = append(jsonStruct.Added, output.Path)
|
|
case archive.ChangeDelete:
|
|
jsonStruct.Deleted = append(jsonStruct.Deleted, output.Path)
|
|
default:
|
|
return jsonStruct, errors.Errorf("output kind %q not recognized", output.Change.String())
|
|
}
|
|
}
|
|
return jsonStruct, nil
|
|
}
|
|
|
|
func diffCmd(c *cli.Context) error {
|
|
if len(c.Args()) != 1 {
|
|
return errors.Errorf("container, layer, or image name must be specified: kpod diff [options [...]] ID-NAME")
|
|
}
|
|
if err := validateFlags(c, diffFlags); err != nil {
|
|
return err
|
|
}
|
|
config, err := getConfig(c)
|
|
if err != nil {
|
|
return errors.Wrapf(err, "could not get config")
|
|
}
|
|
|
|
server, err := libkpod.New(config)
|
|
if err != nil {
|
|
return errors.Wrapf(err, "could not get container server")
|
|
}
|
|
defer server.Shutdown()
|
|
|
|
to := c.Args().Get(0)
|
|
changes, err := server.GetDiff("", to)
|
|
if err != nil {
|
|
return errors.Wrapf(err, "could not get changes for %q", to)
|
|
}
|
|
|
|
diffOutput := []diffOutputParams{}
|
|
outputFormat := c.String("format")
|
|
|
|
for _, change := range changes {
|
|
|
|
params := diffOutputParams{
|
|
Change: change.Kind,
|
|
Path: change.Path,
|
|
}
|
|
diffOutput = append(diffOutput, params)
|
|
}
|
|
|
|
var out formats.Writer
|
|
|
|
if outputFormat != "" {
|
|
switch outputFormat {
|
|
case formats.JSONString:
|
|
data, err := formatJSON(diffOutput)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
out = formats.JSONStruct{Output: data}
|
|
default:
|
|
return errors.New("only valid format for diff is 'json'")
|
|
}
|
|
} else {
|
|
out = stdoutStruct{output: diffOutput}
|
|
}
|
|
formats.Writer(out).Out()
|
|
|
|
return nil
|
|
}
|