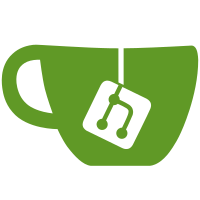
Vendor updated containers/image and containers/storage, along with any new dependencies they drag in, and updated versions of other dependencies that happen to get pulled in. github.com/coreos/go-systemd/daemon/SdNotify() now takes a boolean to control whether or not it unsets the NOTIFY_SOCKET variable from the calling process's environment. Adapt. github.com/opencontainers/runtime-tools/generate/Generator.AddProcessEnv() now takes the environment variable name and value as two arguments, not one. Adapt. Signed-off-by: Nalin Dahyabhai <nalin@redhat.com>
61 lines
2.1 KiB
Go
61 lines
2.1 KiB
Go
// Note: Consider the API unstable until the code supports at least three different image formats or transports.
|
|
|
|
package signature
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/containers/image/manifest"
|
|
"github.com/opencontainers/go-digest"
|
|
)
|
|
|
|
// SignDockerManifest returns a signature for manifest as the specified dockerReference,
|
|
// using mech and keyIdentity.
|
|
func SignDockerManifest(m []byte, dockerReference string, mech SigningMechanism, keyIdentity string) ([]byte, error) {
|
|
manifestDigest, err := manifest.Digest(m)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
sig := privateSignature{
|
|
Signature{
|
|
DockerManifestDigest: manifestDigest,
|
|
DockerReference: dockerReference,
|
|
},
|
|
}
|
|
return sig.sign(mech, keyIdentity)
|
|
}
|
|
|
|
// VerifyDockerManifestSignature checks that unverifiedSignature uses expectedKeyIdentity to sign unverifiedManifest as expectedDockerReference,
|
|
// using mech.
|
|
func VerifyDockerManifestSignature(unverifiedSignature, unverifiedManifest []byte,
|
|
expectedDockerReference string, mech SigningMechanism, expectedKeyIdentity string) (*Signature, error) {
|
|
sig, err := verifyAndExtractSignature(mech, unverifiedSignature, signatureAcceptanceRules{
|
|
validateKeyIdentity: func(keyIdentity string) error {
|
|
if keyIdentity != expectedKeyIdentity {
|
|
return InvalidSignatureError{msg: fmt.Sprintf("Signature by %s does not match expected fingerprint %s", keyIdentity, expectedKeyIdentity)}
|
|
}
|
|
return nil
|
|
},
|
|
validateSignedDockerReference: func(signedDockerReference string) error {
|
|
if signedDockerReference != expectedDockerReference {
|
|
return InvalidSignatureError{msg: fmt.Sprintf("Docker reference %s does not match %s",
|
|
signedDockerReference, expectedDockerReference)}
|
|
}
|
|
return nil
|
|
},
|
|
validateSignedDockerManifestDigest: func(signedDockerManifestDigest digest.Digest) error {
|
|
matches, err := manifest.MatchesDigest(unverifiedManifest, signedDockerManifestDigest)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if !matches {
|
|
return InvalidSignatureError{msg: fmt.Sprintf("Signature for docker digest %q does not match", signedDockerManifestDigest)}
|
|
}
|
|
return nil
|
|
},
|
|
})
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return sig, nil
|
|
}
|