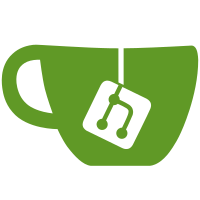
Update the vendored copy of github.com/containers/image to revision efae29995d4846ffa6163eb4d466fd61bda43aae. Signed-off-by: Nalin Dahyabhai <nalin@redhat.com>
30 lines
772 B
Go
30 lines
772 B
Go
package strslice
|
|
|
|
import "encoding/json"
|
|
|
|
// StrSlice represents a string or an array of strings.
|
|
// We need to override the json decoder to accept both options.
|
|
type StrSlice []string
|
|
|
|
// UnmarshalJSON decodes the byte slice whether it's a string or an array of
|
|
// strings. This method is needed to implement json.Unmarshaler.
|
|
func (e *StrSlice) UnmarshalJSON(b []byte) error {
|
|
if len(b) == 0 {
|
|
// With no input, we preserve the existing value by returning nil and
|
|
// leaving the target alone. This allows defining default values for
|
|
// the type.
|
|
return nil
|
|
}
|
|
|
|
p := make([]string, 0, 1)
|
|
if err := json.Unmarshal(b, &p); err != nil {
|
|
var s string
|
|
if err := json.Unmarshal(b, &s); err != nil {
|
|
return err
|
|
}
|
|
p = append(p, s)
|
|
}
|
|
|
|
*e = p
|
|
return nil
|
|
}
|